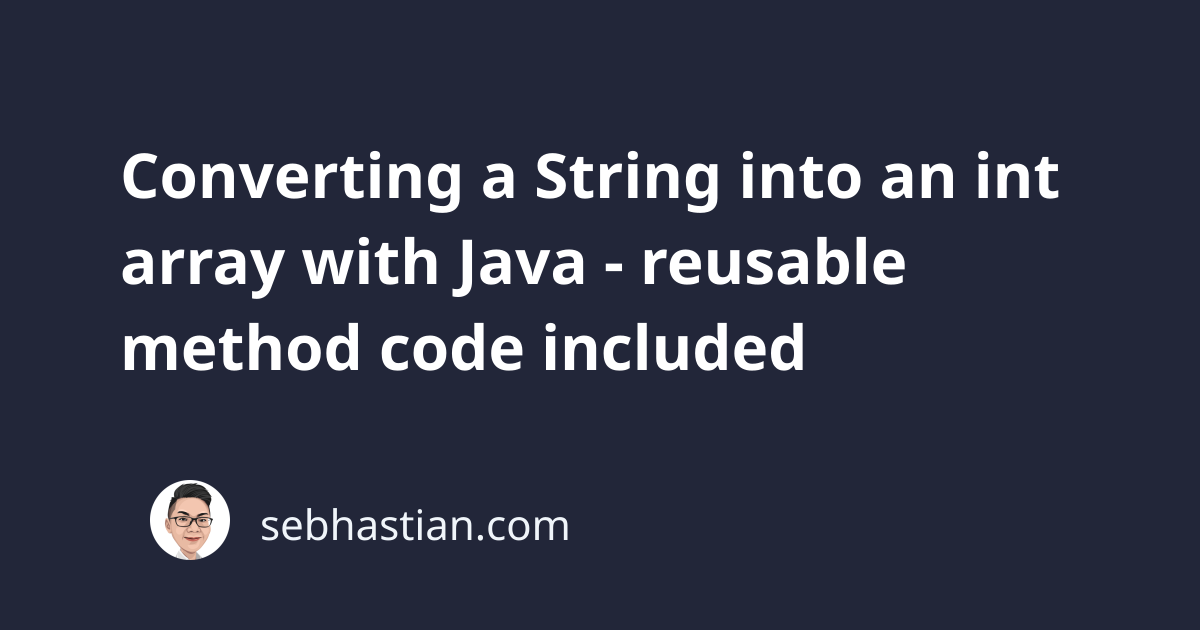
In Java, you can convert a String
type variable into an int[]
(or int
array) by using a combination of the String.split()
method and a for
loop.
Suppose you want to have a String
variable as follows:
String myStr = "12345";
To convert the type, you need to use the String.split()
method to create an array of strings first:
String[] strArr = myStr.split("");
Once you have an array of String
, you need to create an array of int
and initialize the size using the value of strArr.length
property.
After that, create a for
loop statement that will iterate as many times as the strArr.length
property.
For each loop, assign the value of strArr[i]
as the value of the intArr[i]
element.
You also need to call the Integer.parseInt()
method to convert each String
into an int
as shown below:
int[] intArr = new int[strArr.length];
for (int i = 0; i < strArr.length; i++) {
intArr[i] = Integer.parseInt(strArr[i]);
}
System.out.println(Arrays.toString(intArr));
Here’s the output of the println()
method above:
[1, 2, 3, 4, 5]
The complete code for converting a String
into an int[]
can then be extracted into a Java static method.
Each time you need to convert a String
type to an int[]
type, you can just call the method.
Let’s name the method as convertStrToIntArr()
:
static int[] convertStrToIntArr(String input) {
String[] strArr = input.split("");
int[] intArr = new int[strArr.length];
for (int i = 0; i < strArr.length; i++) {
intArr[i] = Integer.parseInt(strArr[i]);
}
return intArr;
}
Now that you have the method, you can call it whenever you need to do the conversion from String
type into an int[]
:
public static void main(String[] args) {
String myStr = "12345";
int[] output = convertStrToIntArr(myStr);
System.out.println(Arrays.toString(output));
// [1, 2, 3, 4, 5]
}
Keep in mind that you need to match the String.split()
pattern with the pattern of your String
variable.
If your String
variable contains white spaces, you might want to split it by removing the spaces:
public static void main(String[] args) {
String myStr = "12 34 5";
String[] strArr = myStr.split(" ");
int[] intArr = new int[strArr.length];
for (int i = 0; i < strArr.length; i++) {
intArr[i] = Integer.parseInt(strArr[i]);
}
System.out.println(Arrays.toString(intArr));
// [12, 34, 5]
}
Additionally, you can add a second parameter to the convertStrToIntArr()
method to handle the changing patterns you may have in your String
variable.
By adding the pattern
String parameter to the method and passing it as the split()
argument, you can determine the String
pattern on call.
To allow the pattern to default to an empty string, create an overload of the method as follows:
// Original method
static int[] convertStrToIntArr(String input) {
String[] strArr = input.split("");
int[] intArr = new int[strArr.length];
for (int i = 0; i < strArr.length; i++) {
intArr[i] = Integer.parseInt(strArr[i]);
}
return intArr;
}
// Overloaded method
static int[] convertStrToIntArr(String input, String pattern) {
String[] strArr = input.split(pattern);
int[] intArr = new int[strArr.length];
for (int i = 0; i < strArr.length; i++) {
intArr[i] = Integer.parseInt(strArr[i]);
}
return intArr;
}
Now you can call the overloaded method anytime you need a custom pattern:
String myStr1 = "12345";
int[] intArr1 = convertStrToIntArr(myStr1);
System.out.println(Arrays.toString(intArr1));
//[1, 2, 3, 4, 5]
String myStr2 = "12 34 5";
int[] intArr2 = convertStrToIntArr(myStr2, " ");
System.out.println(Arrays.toString(intArr2));
// [12, 34, 5]
Now you’ve learned how to convert a String
type into an int
array type in Java.
Feel free to use the convertStrToIntArr()
method from the examples above in your Java project. 😉