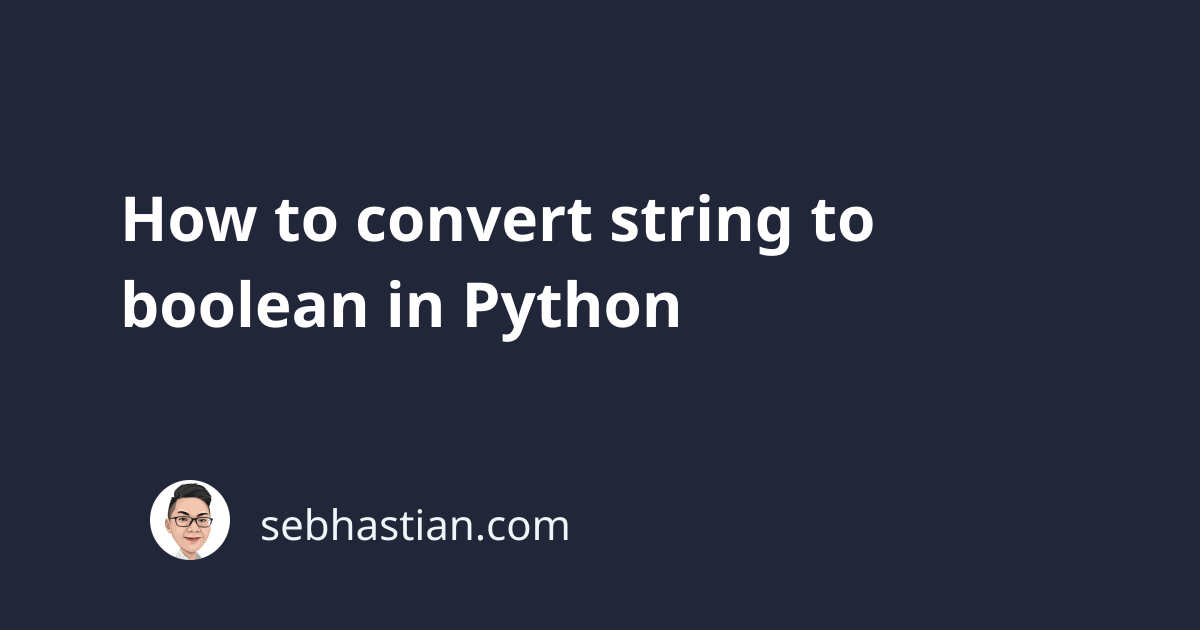
To convert a string value to a boolean in Python, you can use either the bool()
or eval()
function.
Which function to use depends on the string value you have and the result you want to achieve.
The bool()
function converts all non-empty string values to True
, returning False
only for an empty string:
>>> bool("True")
True
>>> bool("False")
True
>>> bool("")
False
>>> bool(" ")
True
As you can see, a string with a single white space still returns True
when using the bool()
function.
If you want to get the boolean False
when the string is ‘False’, then you need to use the eval()
function:
>>> eval("True")
True
>>> eval("False")
False
>>> eval("")
SyntaxError: invalid syntax
But remember that the eval()
function is dangerous as it can run the string argument as if it were part of your source code.
If you pass "os.system('rm -rf /')"
as the string to evaluate, then eval()
will start deleting all files on your computer.
To prevent this security problem, you can use the ast.literal_eval()
function, which only evaluates valid Python data types.
import ast
>>> ast.literal_eval("True")
True
>>> ast.literal_eval("False")
False
>>> ast.literal_eval("")
SyntaxError: invalid syntax
The literal_eval()
is used in the same way as eval()
only with the extra security of validating if what you pass is a valid Python expression.
And that’s how you convert a string to a boolean in Python. I hope this tutorial is helpful. Happy coding! 🙌