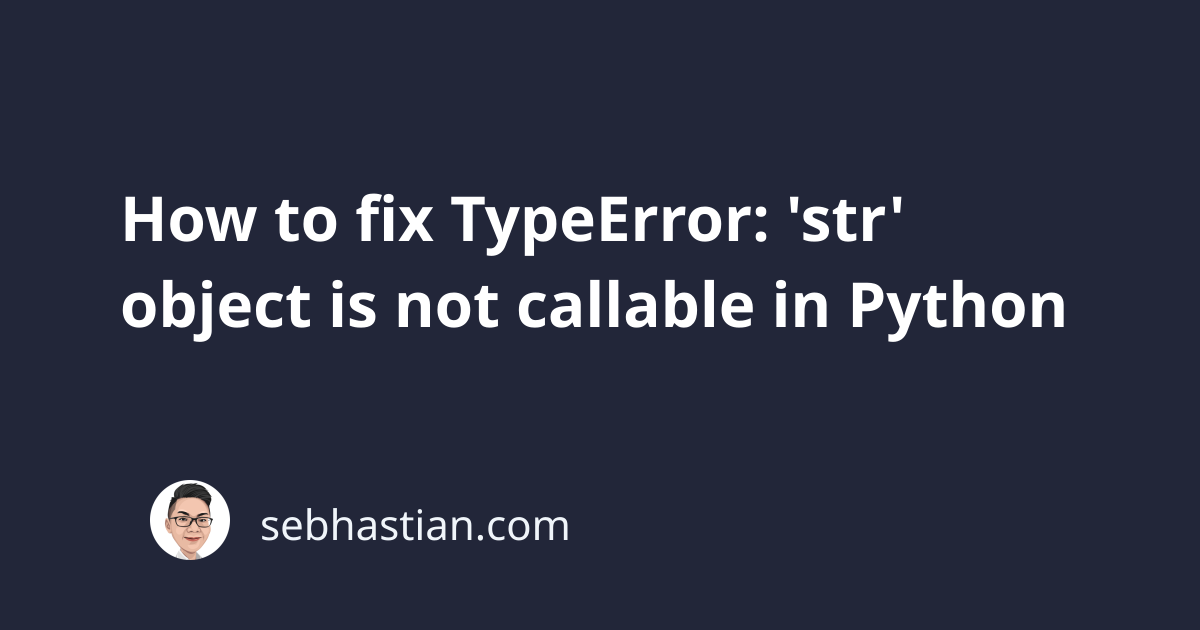
One error that you might encounter when working with Python is:
TypeError: 'str' object is not callable
Through my experience, there are two possible causes for this error:
- You mistakenly called a string as if it were a function
- You involuntarily replaced the
str()
function with an actual string.
This tutorial will show you how I fixed this error in both scenarios.
1. You mistakenly called a string as if it were a function
Suppose you declare a string object named my_string
as follows:
my_string = "Hello"
Next, you attempt to print the string value as follows:
print(my_string())
Notice how there’s a pair of round brackets (parentheses) added next to my_string
.
When you run the code, an error is raised:
Traceback (most recent call last):
File "main.py", line 3, in <module>
print(my_string())
TypeError: 'str' object is not callable
This error occurs because unlike a function that’s callable, a string object can’t be called using parentheses.
If you want to print the value of a variable, just write the variable name in the print()
function without extra parentheses:
my_string = "Hello"
print(my_string) # Hello
Without adding the parentheses, Python is able to print the value of the string object and you don’t receive any error.
2. You involuntarily replaced the str()
function
The str()
function is used to convert an object into a string str
object.
This function comes in handy when you need to concatenate a value of a different type with a string.
For example, you can convert an integer into a string when concatenating it:
my_age = 29
print("I'm " + str(my_age) + " years old.")
If you don’t convert my_age
to string, you’ll get an error output.
In my experience, Python programmers tend to accidentally replace the str()
function with an actual string as follows:
str = "Hello"
The above code assigns a string object to the str
variable, replacing the str()
function with an actual string.
This will cause the error when you need to convert a value using str()
as follows:
str = "Hello!"
my_age = 29
print(str)
print("I'm " + str(my_age) + " years old.")
Output:
Hello!
Traceback (most recent call last):
File "index.py", line 5, in <module>
print("I'm " + str(my_age) + " years old.")
TypeError: 'str' object is not callable
While Python can print the string ‘Hello!’ stored in str
just fine, calling the same variable as a function in str(my_age)
causes an error.
To resolve this error, you need to avoid replacing the str()
function with a string.
You need to choose another name for that string. For example:
greeting = "Hello!" # ✅
my_age = 29
print(greeting) # ✅
print("I'm " + str(my_age) + " years old.")
Output:
Hello!
I'm 29 years old.
As you can see, the error is resolved because now the str
variable correctly points to the built-in str()
function.
Summary
The error TypeError: 'str' object is not callable
occurs when you attempt to call a string as if it were a function. To resolve this error, you need to avoid calling a string object as if it were a function.
I usually saw this error happens because of two common scenarios: a pair of rounds brackets added when accessing the string, or the str()
function got replaced with an actual string.
With the examples shown above, you should be able to identify the cause of this error and fix it yourself.
I hope this tutorial helps. See you in other tutorials! 👍