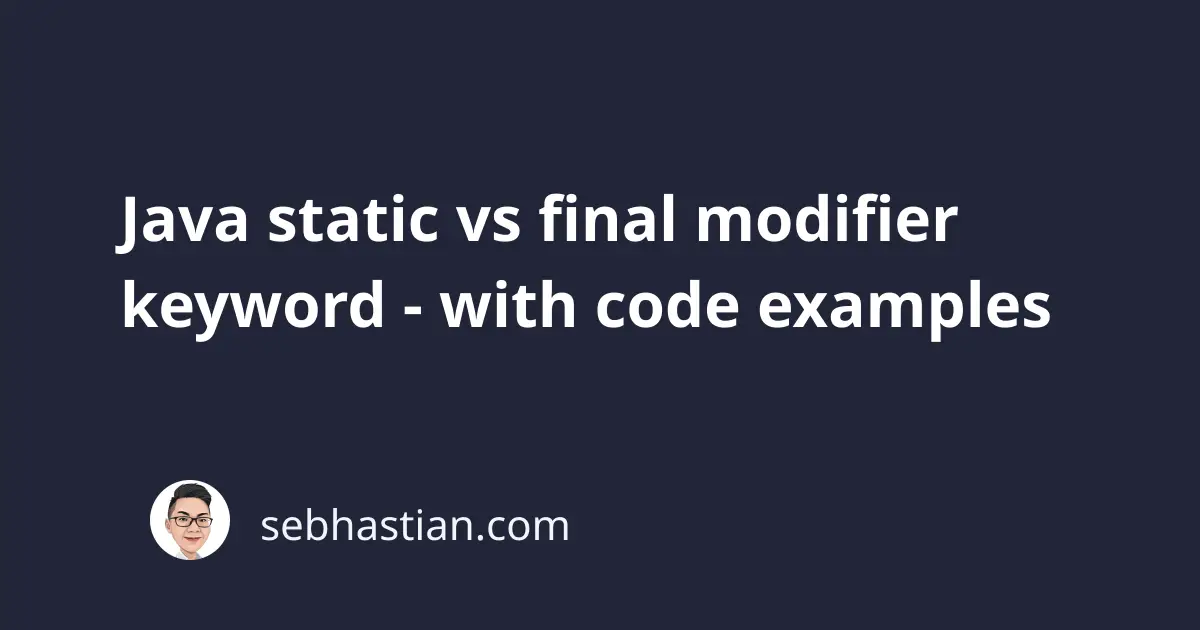
In Java, both static
and final
modifiers are non-access modifiers that modify the way your defined entities behave.
Although both are non-access modifier keywords, the static
and final
keywords are used for different purposes.
This tutorial will help you learn the differences between the two keywords, starting with the static
keyword.
Java static modifier explained
The static
keyword is used to define classes, variables, methods, or blocks that are static in nature.
Static variables and methods of a class
can be accessed without having to create an instance of that class
.
In the example below, the Helper
class has a static variable name
and a static method hello()
:
class Helper {
static String name = "Nathan";
static void hello() {
System.out.println("Hello World!");
}
}
Because both name
and hello()
are static, you can call them without having to create an instance of the Helper
class.
The following code will work just fine:
class Main {
public static void main(String[] args) {
System.out.println(Helper.name); // Nathan
Helper.hello(); // Hello World!
Helper helper = new Helper(); // create an instance of helper
System.out.println(helper.name); // Nathan
}
}
As you can see from the example above, you can still create an instance of the Helper
class and access its members just fine.
But if you’re using IDEs like Android Studio or IntelliJ, you would be recommended to access the members from the class directly.
The static
variable values can be changed, and you can also declare one without initializing it:
class Main {
public static void main(String[] args) {
Helper.name = "John Doe";
Helper.age = 29;
}
}
class Helper {
static String name = "Nathan";
static int age;
}
And the static
methods can be overridden:
class Helper {
static String name = "Nathan";
static void hello() {
System.out.println("Hello World!");
}
}
class Speak extends Helper {
// Override hello() method
static void hello(String name){
System.out.println("Hi! My name is "+ name);
}
}
You can also create a nested class using the static
modifier:
class Main {
public static void main(String[] args) {
System.out.println(Author.name);
}
static class Author {
static String name = "Nathan";
}
}
Without the static
modifier, the inner class Author
class can’t have static members.
Finally, you can also write a static
block as follows:
class Main {
public static void main(String[] args) {
System.out.println("Main block output");
}
static {
System.out.println("Static block output");
}
static {
System.out.println("Static block output 2");
}
}
When you run the Main
class above, the generated output will be as follows:
Static block output
Main block output
Notice that the order of the output is reversed. The static
block output is printed before the main
block.
This is because static
blocks are executed as soon as the class
is loaded.
You can add as many static
blocks as you need in one Java class
.
To summarize, the static
modifier keyword can be used to create four entities:
static
variablesstatic
methodsstatic
nested class- And
static
blocks
Now that you’ve learned about the static
keyword, let’s learn about the final
keyword next.
Java final modifier explained
The final
modifier in Java is used to create an entity that is final or fixed in nature.
This means the entity value or definition can’t be changed after initialization.
For example, you can create a Human
class with fixed tribe and genus specifications:
class Human {
final String tribe = "Hominini";
final String genus = "Homo";
String name = "Nathan";
}
You can’t change any of the final
variables from the class
instance as shown below:
class Main {
public static void main(String[] args) {
Human person = new Human();
person.tribe = "Panina"; // Error can't assign to static variable
person.name = "Jack"; // OK
}
}
A final
method can’t be overridden, and a final
class can’t be extended by another class.
Also, you can’t use the final
keyword when creating a block.
To summarize, you can use the final
keyword to create three entities:
final
variablesfinal
methodsfinal
classes
Differences between final and static keywords
To conclude, the static
modifier is used to create entities that can be accessed without having to instantiate the holding class
first.
With the static
modifier, you can:
- Access
static
variables directly from theclass
- Access
static
methods directly from theclass
- Create
static
nested classes withstatic
members - Create
static
blocks that get executed as soon as the holdingclass
is loaded.
On the other hand, the final
modifier is used to create entities that are fixed:
- You can’t change the value of
final
variables - You can’t override
final
methods - You can’t extend
final
classes
Using both static and final modifiers together
Finally, you can also use both static
and final
modifiers to create fixed variables or methods that can be accessed from the holding class
directly.
Here’s an example:
class Human {
static final String tribe = "Hominini";
static final String genus = "Homo";
}
Both tribe
and genus
can be accessed directly, but their values can’t be changed:
class Main {
public static void main(String[] args) {
System.out.println(Human.tribe);
System.out.println(Human.genus);
Human.tribe = "Gorillini"; // ERROR
Human.genus = "Gorilla"; // ERROR
}
}
Now you’ve learned the differences between static
and final
modifiers in Java. Nice work! 👍