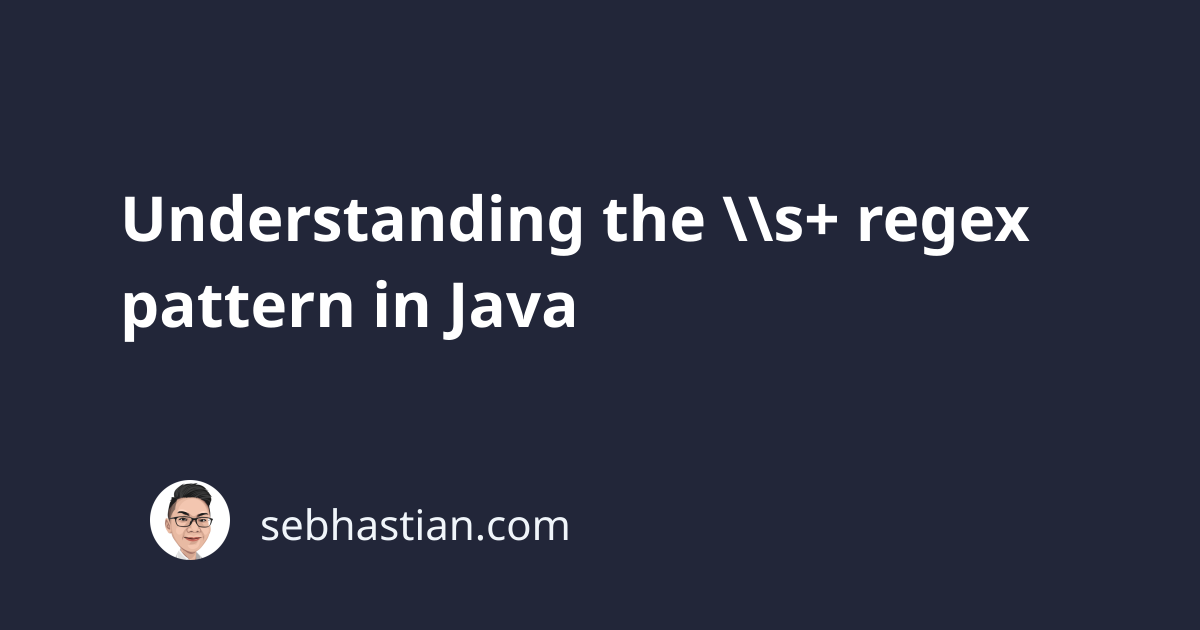
The Java regex pattern \\s+
is used to match multiple whitespace characters when applying a regex search to your specified value.
The pattern is a modified version of \\s
which is used to match a single whitespace character.
The difference is easy to see with an example. Suppose you have a String
type variable named myStr
as shown below:
String myStr = "A Wonderful World";
System.out.println(myStr.replaceAll("\\s", "?"));
System.out.println(myStr.replaceAll("\\s+", "?"));
The string has three whitespaces between the words, and there are two replaceAll()
method calls using the pattern \\s
and \\s+
.
The output of the println()
method will be as shown below. Note the different amount of ?
marks in the two strings:
A???Wonderful???World
A?Wonderful?World
When you use the \\s
pattern, each whitespace is counted as a separate match.
With the \\s+
pattern, the three whitespaces in the string will be counted as one, so they will be replaced with a single ?
symbol instead of three.
The +
sign in the regex pattern is also known as the greedy quantifier. It allows you to count multiple occurrences of the pattern as a single occurrence.
And that’s how the \\s+
regex pattern works in Java. 😉