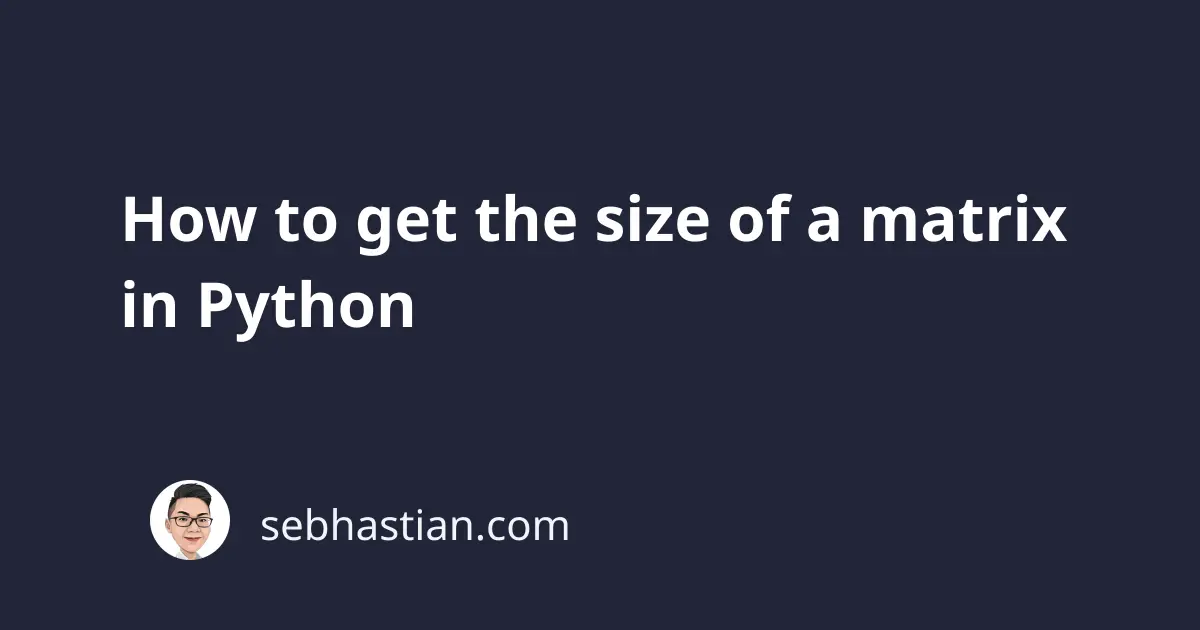
To get the size of a matrix in Python, you need to call the shape
property of the matrix object.
For example, suppose you created a matrix object using NumPy as shown below:
from numpy import matrix
my_matrix = matrix([[1,2],[3,4], [5,6]])
To know the size of the matrix and get how many rows and columns it has, you need to access the shape
attribute of the object:
print(my_matrix.shape)
Output:
(3, 2)
The shape
attribute returns a tuple that shows the size of the matrix in N rows and N columns format.
In this example, the matrix has 3 rows and 2 columns. You can use this attribute for any matrix you have in your source code.