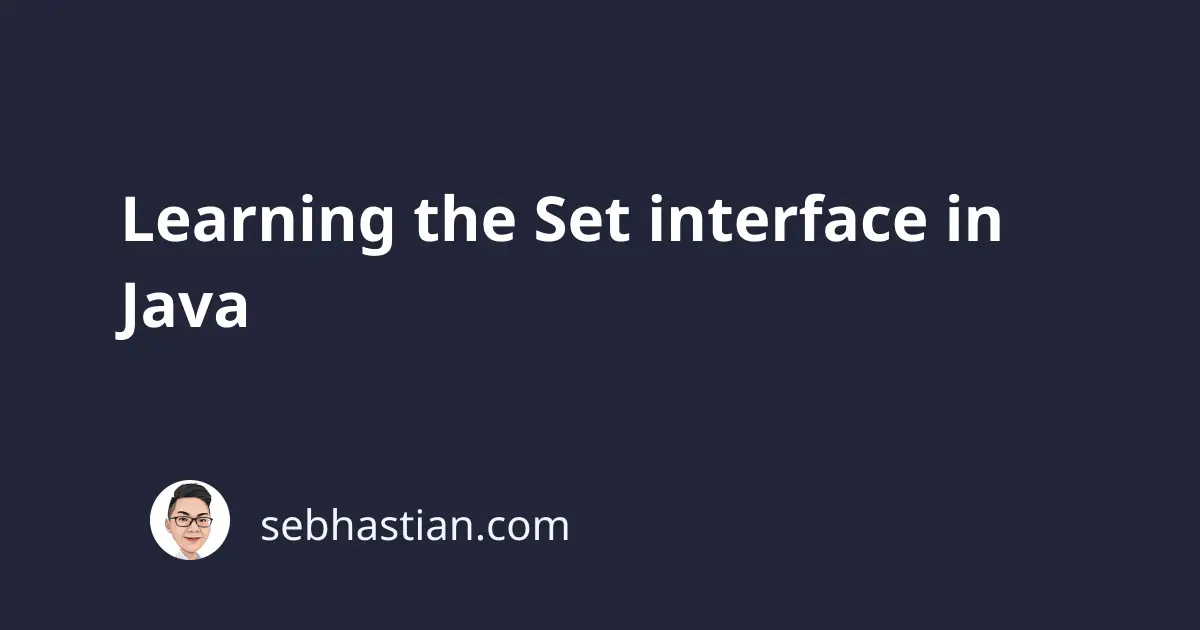
The Java Set
interface is one of the two sub-interfaces of the Collection
class, with the other being the List
interface.
The Set
interface describes the Set abstract data type on how it is implemented in Java.
A Set
type is a data type that can store unique values without saving the order of when the values are added.
A Set
cannot contain duplicate values. You need to use a List
when you need to store duplicate values.
In Java, there are several classes that implement the Set
interface:
AbstractSet
ConcurrentSkipListSet
CopyOnWriteArraySet
EnumSet
HashSet
JobStateReasons
LinkedHashSet
TreeSet
But among these implementing classes, only a few are used to create a Set
in Java. They are:
EnumSet
HashSet
LinkedHashSet
TreeSet
The four classes enable you to create a Set
instance with different specializations.
For example, the EnumSet
is a specialized implementation of the Set
interface to store Java enum
values.
On the other hand, the HashSet
class stores your values using a HashMap
instance internally.
All classes that implement the Set
interface have methods to help you manipulate the data contained in the type.
For example, there are add()
and remove()
methods to help you add and remove values from the type.
Here’s an example of creating a HashSet
variable:
Set<String> mySet = new HashSet<>();
mySet.add("Nathan");
mySet.add("Jack");
System.out.println("mySet values: " + mySet);
mySet.remove("Jack");
System.out.println("mySet values after remove: " + mySet);
As you can see from the code above, you can create a new instance of HashSet
for variables of Set
type.
This is because Set
is implemented on all Java classes used to create a Set data type.
The code above will generate the following output:
mySet values: [Nathan, Jack]
mySet values after remove: [Nathan]
The list of defined methods in the Set
interface is as follows:
add()
- adds an element passed as its argument to the setaddAll()
- add all elements of a collection to the set. You need to pass aCollection
as its argumentiterator()
- returns an iterator that can be used to access elements of the setremove()
- removes the element you passed as the argument from the setremoveAll()
- remove all elements from the set that is present in the argument. Accepts aCollection
as its argumentretainAll()
- retain all elements in the set that are also present in the argument. Accepts aCollection
typeclear()
- remove all elements from the setsize()
- returns the number of elements present in the settoArray()
- returns an array containing all elements stored in the setcontains()
- returnstrue
if the set contains the specified elementcontainsAll()
- returnstrue
if the set contains all elements of the specified argument. Accepts aCollection
typehashCode()
- returns a hash code value (address of the element in the set)
Now you’ve learned how the Set
interface works in Java.
Please remember that you need to use one of the classes that implement the Set
interface to create one.