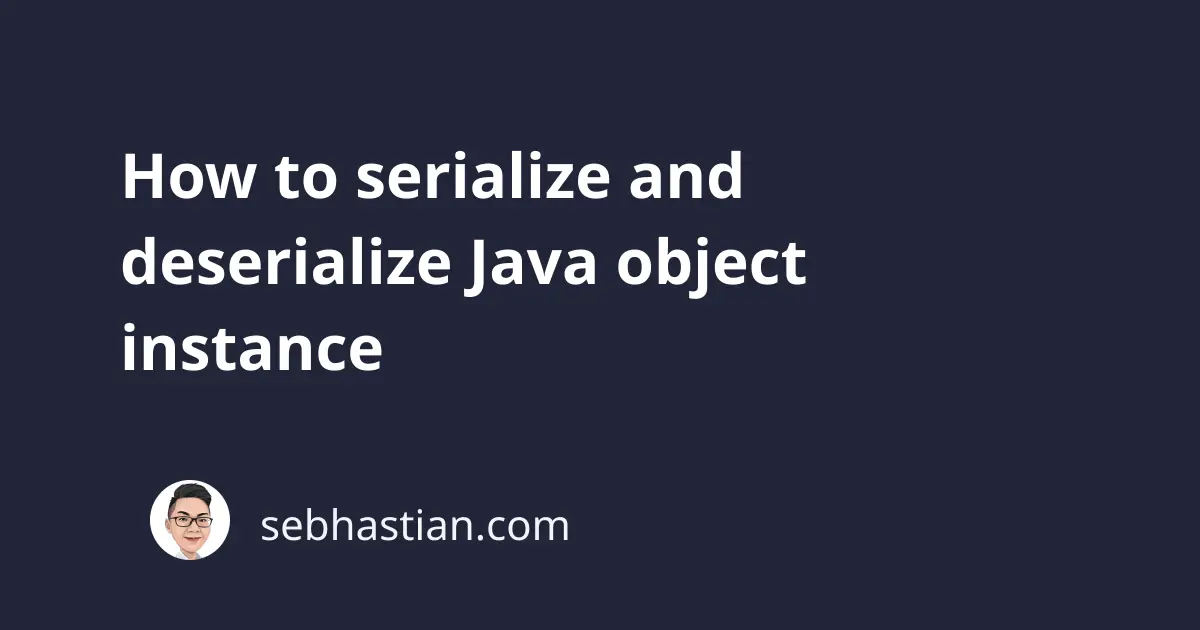
Serialization is the process of converting a Java object instance into a series of bytes. You can then write the serialized object into a file or save it to a database for later use.
Deserialization is the reverse of serialization, which means you convert the Java object bytes back into a Java object instance, allowing you to read the information contained within the serialized object.
The ability to serialize and deserialize Java object allows you to carry information out from one Java Virtual Machine to another.
This tutorial will help you learn how to serialize and deserialize a Java object. Let’s start with serializing an object first.
How to serialize a Java object
To serialize a Java object, the class that you use to instantiate that object must implement the Serializable
interface.
Here’s an example:
import java.io.Serializable;
class Car implements Serializable {
String name = "Tesla Model S";
String owner = "Nathan";
int year = 2019;
}
Most built-in Java classes like ArrayList
and HashSet
already implements the Serializable
class, so you can serialize them too.
To start the serialization process, you need to create a new object from the class first:
Car myCar = new Car();
Next, you need to create a new instance of FileOutputStream
and ObjectOutputStream
classes from the java.io
package.
The FileOutputStream
creates an output stream, enabling you to write data into a computer file and save that file outside of Java application. You need to pass the String name
of the new file that will be created by the object as its argument:
FileOutputStream fos = new FileOutputStream("serializable.ser");
The ObjectOutputStream
allows you to convert and write object data into an output stream. After you create the FileOutputStream
instance fos
above, pass that stream into the ObjectOutputStream
.
Also, wrap the instantiation of both classes using a try..catch
block to handle their exceptions:
import java.io.*;
Car myCar = new Car();
try{
FileOutputStream fos = new FileOutputStream("serializable.ser");
ObjectOutputStream oos = new ObjectOutputStream(fos);
} catch (Exception e) {
System.out.println(e);
}
Finally, call the ObjectOutputStream.writeObject()
method and pass the myCar
object as its argument as follows:
Car myCar = new Car();
try{
FileOutputStream fos = new FileOutputStream("serializable.ser");
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(myCar);
} catch (Exception e) {
System.out.println(e);
}
After that, you can call the flush()
and close()
method to close the stream and release the memory used for the writing process.
The flush()
method ensures that Java has finished writing the serialized object into the file before closing the stream. If your stream hasn’t finished writing yet, then the close()
method won’t be executed:
Car myCar = new Car();
try{
FileOutputStream fos = new FileOutputStream("serializable.ser");
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(myCar);
oos.flush();
oos.close();
System.out.println("Serialization success");
} catch (Exception e) {
System.out.println(e);
}
When you run the code above inside your main()
method, a new file named serializable.ser
should be created in your Java project root folder:
MyJavaProject
├── MyJavaProject.iml
├── out/
├── serializable.ser
└── src/
Please note that a serialized object file can only be read from inside a Java application. Opening the .ser
file using text editor programs will only show texts and symbols that don’t make sense.
Here’s the full code for serializing the Car
class:
public class Main {
public static void main(String[] args) {
try {
Car myCar = new Car();
FileOutputStream fos = new FileOutputStream("serializable.ser");
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(myCar);
oos.flush();
oos.close();
System.out.println("Serialization success");
} catch (Exception e) {
System.out.println(e);
}
}
}
class Car implements Serializable {
String name = "Tesla Model S";
String owner = "Nathan";
int year = 2019;
}
Now that you’ve learned how to serialize an object into a file, it’s time to learn how to deserialize and read the file content.
How to deserialize a Java object file
To deserialize and read the content of an object file, you need to create new instances of the FileInputStream
and the ObjectInputStream
.
You need to pass the file name into the FileInputStream
constructor just like in FileOutputStream
above:
FileInputStream fis = new FileInputStream("serializable.ser");
Then pass the fis
object into the ObjectInputStream
constructor:
ObjectInputStream ois = new ObjectInputStream();
Finally, call the ObjectInputStream.readObject()
method to return an object from the input stream.
You need to type cast the returned object into a Car
as shown below:
Car readCar = (Car) ois.readObject();
And with that, you can print out the readCar
object attributes as shown below:
Car readCar = (Car) ois.readObject();
System.out.println("Car name:" + readCar.name);
System.out.println("Car owner:" + readCar.owner);
System.out.println("Car production year:" + readCar.year);
Once you’re done accessing the object, you can call the ObjectInputStream.close()
method to close the input stream and release the memory used for the process.
The full code for deserializing an object is as follows:
public class Main {
public static void main(String[] args) {
try {
FileInputStream fis = new FileInputStream("serializable.ser");
ObjectInputStream ois = new ObjectInputStream(fis);
Car readCar = (Car) ois.readObject();
System.out.println("Car name:" + readCar.name);
System.out.println("Car owner:" + readCar.owner);
System.out.println("Car production year:" + readCar.year);
ois.close();
} catch (Exception e) {
System.out.println(e);
}
}
}
And that’s how you deserialize and read the content of a serialized object file.
You can also grab a copy of the source code at GitHub Gist.
Conclusion
The ability to serialize and deserialize Java objects allows you to save an object instance attributes after its instantiation.
Suppose you have a dynamic Car
class with the following constructor:
class Car implements Serializable {
String name;
String owner;
Car(String name, String owner){
this.name = name;
this.owner = owner;
}
}
Then you can serialize each instance of the Car
classes with different name
and owner
values into a file and read them later when you need to.
You need to call the writeObject()
method as many times as the object instance you have:
Car myCar = new Car("Tesla Model S", "Nathan");
Car myOtherCar = new Car("Mercedes-Benz EQS", "Nathan");
FileOutputStream fos = new FileOutputStream("serializable.ser");
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(myCar);
oos.writeObject(myOtherCar);
Then when you have two objects in one serialized file, you need to call the readObject()
method twice during the read process:
FileInputStream fis = new FileInputStream("serializable.ser");
ObjectInputStream ois = new ObjectInputStream(fis);
Car readCar = (Car) ois.readObject();
System.out.println("Car name:" + readCar.name);
System.out.println("Car owner:" + readCar.owner);
Car readOtherCar = (Car) ois.readObject();
System.out.println("Car name:" + readOtherCar.name);
System.out.println("Car owner:" + readOtherCar.owner);
You’ve just learned how serialization and deserialization work in Java. Great job! 😉