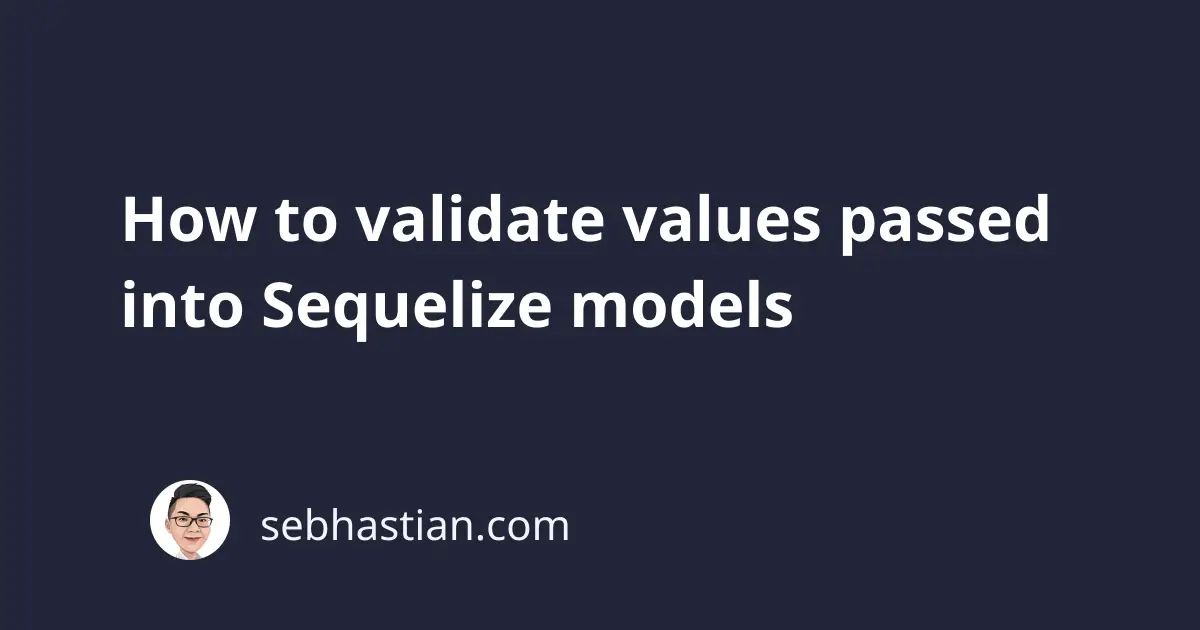
Sequelize provides a validate
option that you can use to validate the attribute values passed into your models.
You can validate individual attributes or the whole model depending on how you define the validate
option.
Let’s see an example of validating attributes in Sequelize.
Suppose you have a Users
table with two columns: firstName
and email
.
CREATE TABLE `Users` (
`id` int NOT NULL AUTO_INCREMENT,
`firstName` varchar(255) DEFAULT NULL,
`email` varchar(255) DEFAULT NULL,
PRIMARY KEY (`id`)
)
When defining the model for the table, you can pass the validate
option to any of your attributes that requires validation.
To validate the email
field, you can use the provided isEmail
validator as follows:
const User = sequelize.define(
"User",
{
firstName: Sequelize.STRING,
email: {
type: Sequelize.STRING,
validate: { isEmail: true },
},
},
{ timestamps: false }
);
By adding the isEmail
validation, Sequelize will throw an error when the email
value you pass fails the validation check.
For example, when inserting a new row using the create()
method as follows:
const response = await User.create({
firstName: "Jack",
email: "jack", // Error: Validation isEmail on email failed
});
Because the email
value above isn’t a valid email address, Sequelize will throw an error and stop your code execution.
You need to add a try..catch
block around the method call to gracefully handle Sequelize validation error.
You can add a validate
option to any of your model attributes.
Here’s how to add length validation to the firstName
attribute:
const User = sequelize.define(
"User",
{
firstName: {
type: Sequelize.STRING,
validate: { len: 5 },
},
email: {
type: Sequelize.STRING,
validate: { isEmail: true },
},
},
{ timestamps: false }
);
The len
validation property above will validate that the firstName
attribute length is 5
or greater.
You can also set a range of minimum and maximum length for the len
validation as follows:
validate: {
len: [5, 10];
}
Now the attribute length must be between 5
and 10
.
Sequelize custom validators
Sequelize provides many built-in validators that you can use in your validate
option.
The len
and isEmail
are two of these built-in validators.
All available validators are listed on Sequelize validation documentation.
When the built-in validators are not sufficient for your project, you can even create a custom validator by passing a function.
For example, here’s a custom validator to check the length of the firstName
attribute:
const User = sequelize.define(
"User",
{
firstName: {
type: Sequelize.STRING,
validate: {
checkLength(value) {
if (value.length < 7) {
throw new Error("Length must be 7 or greater!");
}
},
},
},
},
{ timestamps: false }
);
And that’s how you create a custom validation using Sequelize.
Sequelize validation on the model level
All validations you see above are created on the attributes level.
Sequelize also allows you to write validations on the model level by passing the validate
option to the model (inside the define()
method call)
Here’s an example of a model level validation:
const User = sequelize.define(
"User",
{
firstName: Sequelize.STRING,
email: Sequelize.STRING,
},
{
timestamps: false,
validate: {
userValidation() {
if (this.firstName.length < 7) {
throw new Error("firstName length must be 7 or greater!");
}
if (this.email.includes("@mail.com")) {
throw new Error("Email must not use mail.com address!");
}
},
},
}
);
The userValidation()
function above is defined on the model level. You can use any name for the custom function.
When validating at the model level, the this
keyword refers to the model instance created when you call the create()
method.
The attributes for the model are available as properties of the this
keyword as shown in the example above.
Inside the validation function, you can define as many or as few conditions as you require for the model.
Validating bulkCreate function
By default, Sequelize will turn off the validations for the bulkCreate()
function.
When you need to validate a bulkCreate()
method call, you need to pass the validate: true
option to the method:
await User.bulkCreate(
[
{
firstName: "Jack",
email: "[email protected]",
},
{
firstName: "Susan",
email: "susan",
},
],
{ validate: true }
);
And that’s how you enable validation for the bulkCreate()
method.
Now you’ve learned how to validate values passed into Sequelize models and attributes. Nice work! 👍
For more information on Sequelize validation, you can refer to the Sequelize validations and constraints documentation.