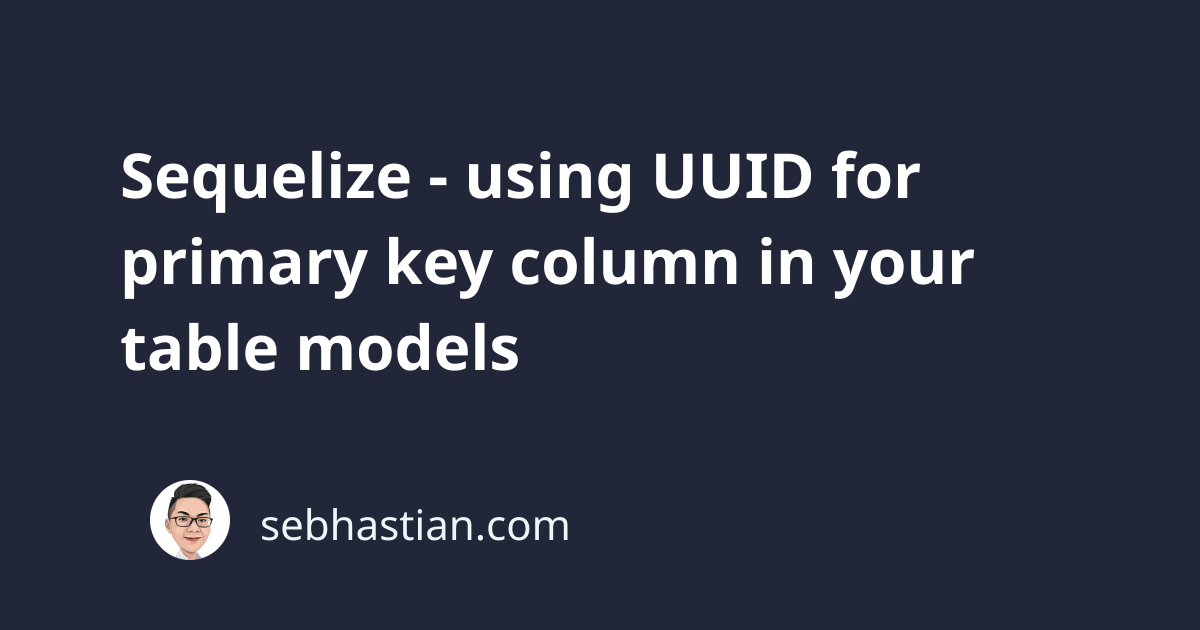
In Sequelize, the UUID
type is used to create a column that stores unique universal identifiers.
When you connect to MySQL, the column with UUID
type will become a CHAR(36)
type because MySQL doesn’t have a native UUID
type (Only PostgreSQL and SQLite has that type)
Sequelize provides UUIDV1
and UUIDV4
as the default value for UUID
type columns that you can use when defining the model.
Let’s see how you can create a Sequelize model with UUID
type as the table’s primary key.
First, create a new Sequelize
instance to connect to your database. I will use MySQL for this example:
const { Sequelize } = require("sequelize");
const sequelize = new Sequelize("[database name]", "[username]", "[password]", {
host: "localhost",
dialect: "mysql",
});
You can change the database name
, [username]
, [password]
, and dialect
options above to match your database choice.
Next, create a new model using sequelize.define()
method as follows:
const User = sequelize.define("Users", {
id: {
type: Sequelize.UUID,
defaultValue: Sequelize.UUIDV4,
primaryKey: true,
},
firstName: {
type: Sequelize.STRING,
},
lastName: {
type: Sequelize.STRING,
},
});
The model User
above will have three columns:
id
primary key column withUUID
type withSequelize.UUIDV4
as the default valuefirstName
column withSTRING
typelastName
column withSTRING
type
Once you have the model, you can call the sync()
method on the model to create the table (this assumes the table doesn’t exist already in your database)
Once the synchronization is done, you can test the model by inserting a value.
Here’s the complete JavaScript code for testing the UUID
column:
const { Sequelize } = require("sequelize");
const sequelize = new Sequelize("[database name]", "[username]", "[password]", {
host: "localhost",
dialect: "mysql",
});
const User = sequelize.define("Users", {
id: {
type: Sequelize.UUID,
defaultValue: Sequelize.UUIDV4,
primaryKey: true,
},
firstName: {
type: Sequelize.STRING,
},
lastName: {
type: Sequelize.STRING,
},
});
User.sync().then((res) => {
User.create({ firstName: "Nathan", lastName: "Sebhastian" }).then((res) => {
console.log(`Insert successful: ${res.id}`);
sequelize.close();
console.log("connection closed");
});
});
You can copy and save the code above as a file named index.js
and run it using Node server:
node index.js
The output would be similar as shown below:
Executing (default): CREATE TABLE IF NOT EXISTS `Users` (`id` CHAR(36) BINARY ,
`firstName` VARCHAR(255), `lastName` VARCHAR(255), `createdAt` DATETIME NOT NULL,
`updatedAt` DATETIME NOT NULL, PRIMARY KEY (`id`)) ENGINE=InnoDB;
Executing (default): SHOW INDEX FROM `Users`
Executing (default): INSERT INTO `Users`
(`id`,`firstName`,`lastName`,`createdAt`,`updatedAt`)
VALUES (?,?,?,?,?);
Insert successful: d45735b1-27a9-49d9-9fd3-476cec88bd9e
connection closed
You can see the id
primary column populated with UUIDV4
value as shown below:
SELECT id, firstName FROM Users;
-- Output:
-- +--------------------------------------+-----------+
-- | id | firstName |
-- +--------------------------------------+-----------+
-- | 8d8bc566-6a75-4f36-914e-6d4cfec8466b | Nathan |
-- +--------------------------------------+-----------+
Now you’ve learned how to use UUID
type in Sequelize.
Sequelize UUID type for migrations
If you’re using Sequelize migrations to keep track of your database versioning, then you may want to define the columns with UUID
type in your migration files too.
Unfortunately, the Sequelize command-line tool doesn’t have the ability to generate a migration file with the UUID
column yet.
You can generate your migration file with the following command:
npx sequelize-cli model:generate --name Users --attributes firstName:string,lastName:string
The id
column generated for the Users
table will use Sequelize.INTEGER
by default, so you need to change it manually as shown below:
"use strict";
module.exports = {
up: async (queryInterface, Sequelize) => {
await queryInterface.createTable("Users", {
id: {
allowNull: false,
defaultValue: Sequelize.UUIDV4,
primaryKey: true,
type: Sequelize.UUID,
},
firstName: {
type: Sequelize.STRING,
},
lastName: {
type: Sequelize.STRING,
},
createdAt: {
allowNull: false,
type: Sequelize.DATE,
},
updatedAt: {
allowNull: false,
type: Sequelize.DATE,
},
});
},
down: async (queryInterface, Sequelize) => {
await queryInterface.dropTable("Users");
},
};
And that’s how you create a Sequelize migration file with UUID
type column(s).