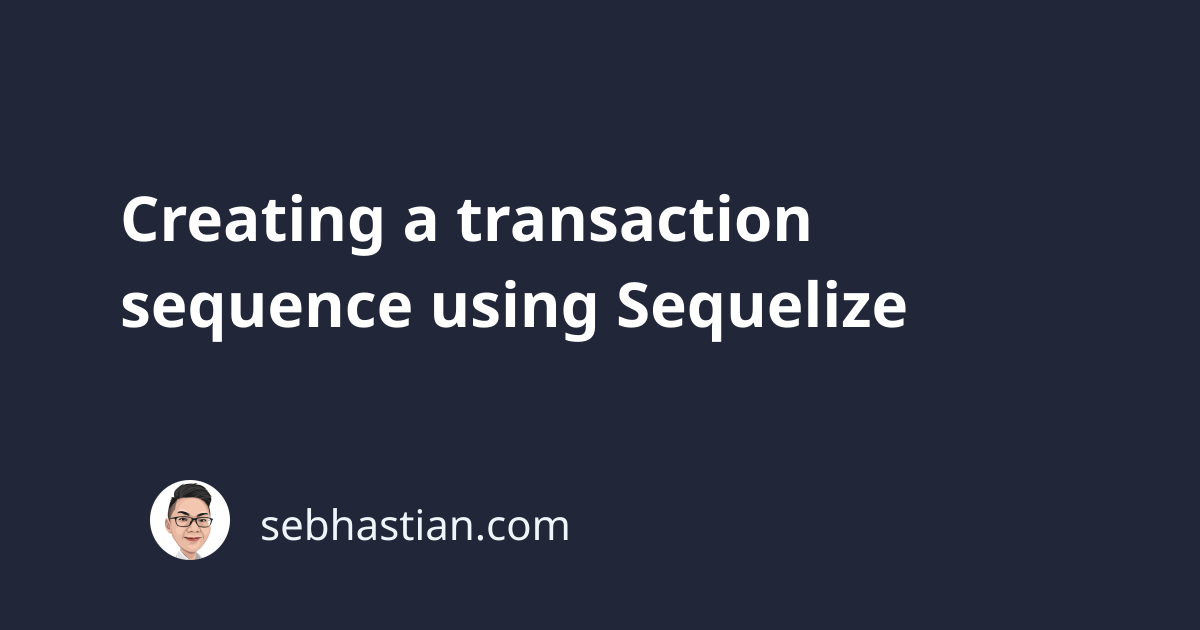
Sequelize supports running SQL statements under a transaction by using the Transaction
object.
To start a transaction using Sequelize, you need to create a Transaction
object by calling sequelize.transaction()
as shown below:
const trx = await sequelize.transaction();
The code above creates a Transaction
instance named trx
.
When you run query methods from your model, you need to pass the trx
object as the transaction
option.
For example, suppose you call a User.create()
method. Here’s how you pass a transaction
option to it:
const user = await User.create({
firstName: 'Nathan',
}, { transaction: trx });
Because you pass the transaction
option to the create()
method, Sequelize won’t execute the instruction to your database until you call the commit()
method from the transaction.
You can add as many statements to run under the transaction as needed.
When you’re ready, call the commit()
method to run the instructions:
const user = await User.create({
firstName: 'Nathan',
}, { transaction: trx });
const invoice = await Invoice.create({
amount: 300,
}, { transaction: trx });
await trx.commit(); // run the transaction
Now the Transaction
object also has the rollback()
method that you can call to roll back the statements.
But to do so, you need to catch the error thrown by the commit()
method.
You need to surround the transaction sequence inside a try..catch
block like this:
try {
const user = await User.create({
firstName: 'Nathan',
}, { transaction: trx });
const invoice = await Invoice.create({
amount: 300,
}, { transaction: trx });
await trx.commit();
} catch (e) {
await trx.rollback();
}
When the transaction execution throws an error, the rollback()
method will be called to undo the changes.
And that’s how you create a transaction sequence using Sequelize.
Sequelize also supports automatic commit and rollback of transaction sequence which is called managed transactions.
You can learn more about it on Sequelize transactions documentation.