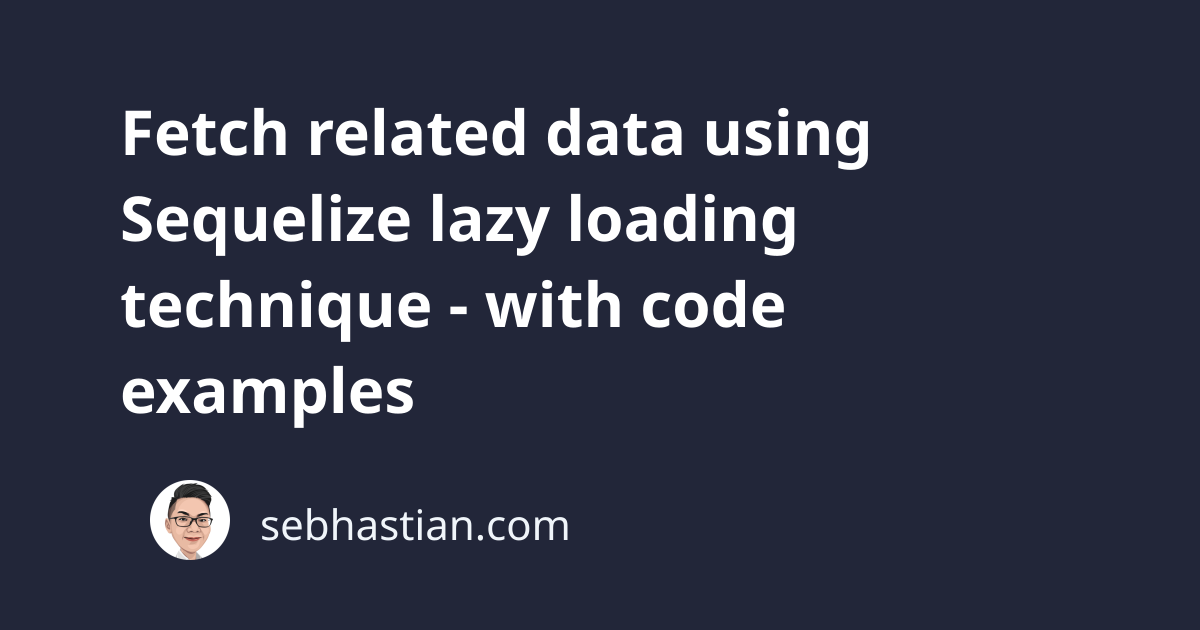
Sequelize lazy loading technique is used to fetch data from model(s) that are related to the main model that you are currently interacting with.
This technique allows you to fetch data that are related to your main model only when you need it.
Let’s see an example of lazy loading in action to understand how it works.
Suppose you have two SQL tables named Users
and Invoices
with the following details:
# Users
+----+-----------+
| id | firstName |
+----+-----------+
| 1 | Nathan |
| 2 | John |
+----+-----------+
#Invoices
+----+--------+--------+
| id | amount | userId |
+----+--------+--------+
| 1 | 300 | 1 |
| 2 | 100 | 2 |
+----+--------+--------+
From the above table, you need to create the models in Sequelize as follows:
const Invoice = sequelize.define(
"Invoice",
{ amount: Sequelize.INTEGER },
{ timestamps: false }
);
const User = sequelize.define(
"User",
{ firstName: Sequelize.STRING },
{ timestamps: false }
);
Now that you have the models to represent the tables, you need to define the associations (or relations) between the models.
One Users
row can have many Invoices
rows, so you need to use User.hasMany()
and Invoice.belongsTo()
methods to create the right associations:
User.hasMany(Invoice);
Invoice.belongsTo(User);
With the associations defined, you can now fetch one model data from the other model that’s associated with it.
The example below fetches the Invoices
rows using the getInvoices()
method generated by Sequelize:
const user = await User.findByPk(1);
const invoices = await user.getInvoices();
console.log(JSON.stringify(invoices, null, 2));
Here’s the output of the console log above:
[
{
"id": 1,
"amount": 300,
"UserId": 1
}
]
The above is an example of how lazy loading technique works. It allows you to fetch the Invoices
data that’s related to the Users
data you’ve already fetched previously.
The getInvoices()
method that you saw above is generated by Sequelize so that you can fetch data related to the Model
where you call the method.
The name of the get
method that’s generated by Sequelize will follow the name of the model you defined in the association methods.
If you associate the User
model with the Role
model, then the name would be getRoles()
:
User.hasMany(Role);
const user = await User.findByPk(1);
const roles = await user.getRoles();
Also, pay attention to the association you set between the models.
If you use the hasOne()
or belongsTo()
methods, then the name of the get
method will be the singular form of the model name.
Take a look at the example below:
User.hasOne(Invoice)
// the get method would be the singular form of the model name
const invoice = await user.getInvoice();
User.hasMany(Invoice)
// the get method would be the plural form of the model name
const invoice = await user.getInvoices();
Now you’ve learned how the lazy loading technique works in Sequelize.
Please note that you can also fetch data from multiple related tables simultaneously (similar to the SQL JOIN
clauses) using Sequelize eager loading method, which you can learn here:
Sequelize eager loading explained with examples
Depending on how you want to fetch your data, you can use either the lazy loading or eager loading technique.