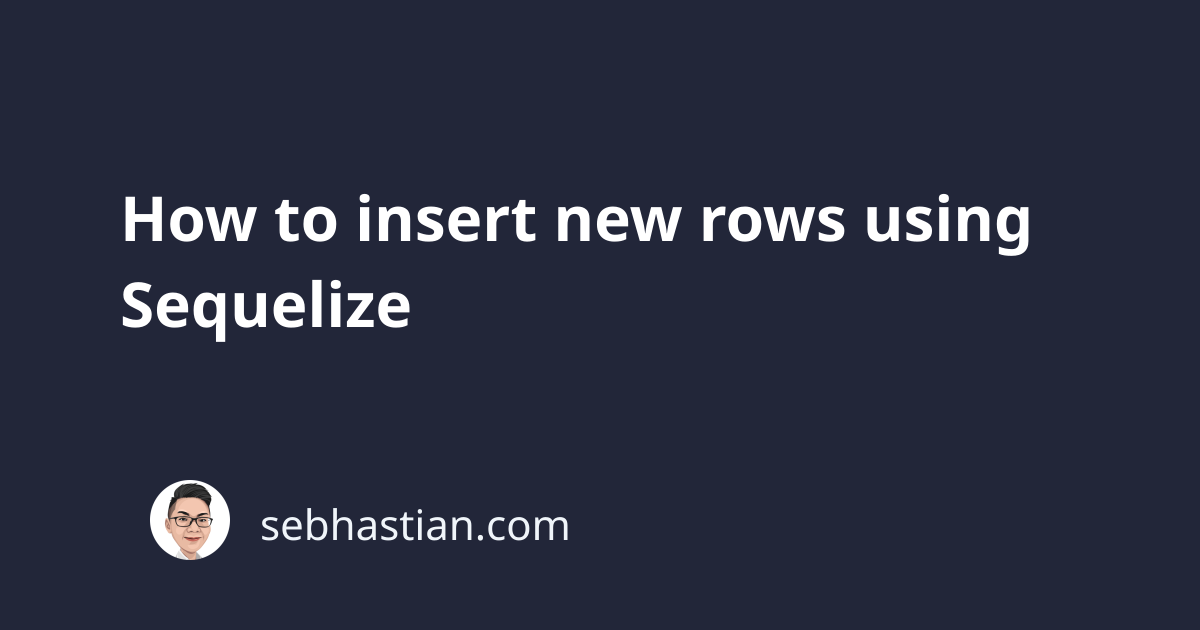
To insert new rows into your SQL table, you need to first create a Sequelize Model
object.
In Sequelize, a Model
is an object that represents your SQL table, giving information to Sequelize about the name, the columns, and their data types.
From the Model
, you can then call the create()
method, which performs an INSERT
SQL statement to your table.
Let’s see a practical example showing you how to insert new rows.
Suppose you have a table named Users
with the following definition:
+-----------+--------------+------+-----+---------+----------------+
| Field | Type | Null | Key | Default | Extra |
+-----------+--------------+------+-----+---------+----------------+
| id | int | NO | PRI | NULL | auto_increment |
| firstName | varchar(255) | YES | | NULL | |
+-----------+--------------+------+-----+---------+----------------+
To create a Model
that represents the Users
table above, you need to call the define()
method as follows:
const User = sequelize.define(
"User",
{ firstName: Sequelize.STRING },
{ timestamps: false }
);
The first argument for the define()
method is the model name, defined as User
in the example above.
By default, Sequelize uses the pluralized form of the model name to search for the represented table.
In this case, the User
model will connect to the Users
table.
The second argument is an attribute object representing the column(s) that the table has. Notice how the id
column is not defined in this object.
This is because Sequelize adds the id
attribute to the Model
automatically. That way, you don’t need to define the same id
attribute for each of your Sequelize models.
The third argument is an option object to modify the Model
generated by the define()
method.
The timestamps: false
option means that Sequelize won’t try to send createdAt
and updatedAt
data when you insert or update a row.
Now that you have the User
model, it’s time to INSERT
a new row to the table using the create()
method:
await User.create({
firstName: "Nathan"
});
The above create()
method call will cause Sequelize to generate and execute the following SQL statement:
INSERT INTO `Users` (`id`, `firstName`)
VALUES (DEFAULT, ?);
A new row will be inserted into the table as shown below:
+----+-----------+
| id | firstName |
+----+-----------+
| 1 | Nathan |
+----+-----------+
The create()
method is used to insert a single row into your SQL table.
When you need to insert multiple rows at once, you need to use the bulkCreate()
method instead.
Finally, you can also write and execute a raw SQL statement using the sequelize.raw()
method.
Now you’ve learned how to insert new rows into your SQL table with Sequelize. Nice work! 😉