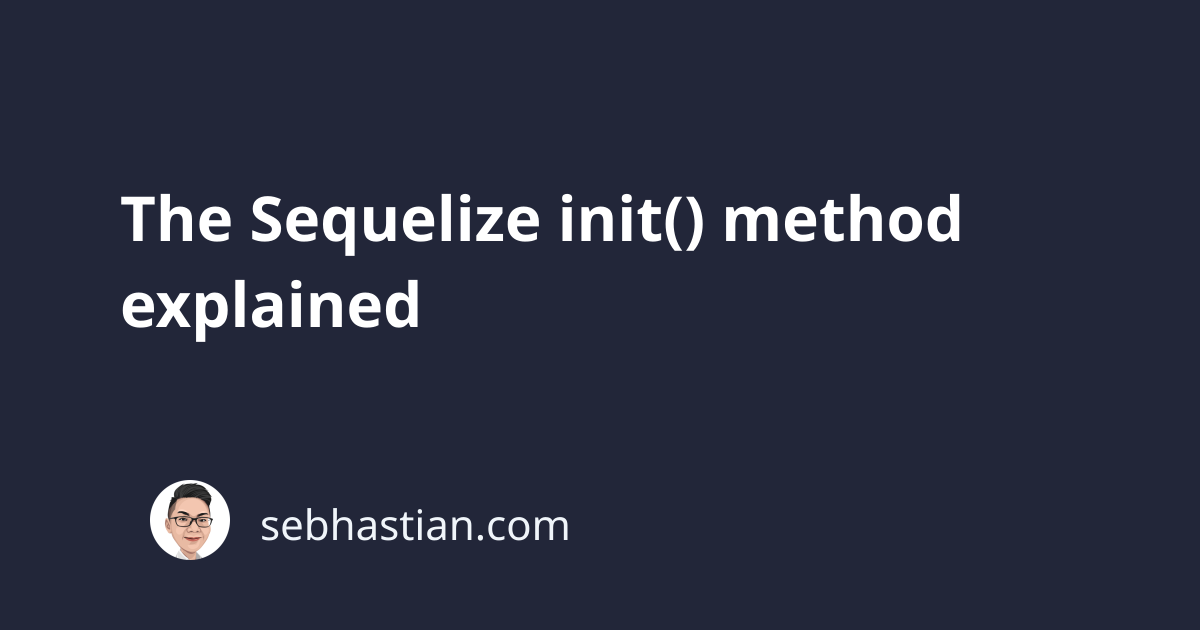
The Sequelize init()
method is a method of the Model
class that is used to initialize a model.
A Sequelize Model
represents a single table in your database.
Just like you specify a table’s columns in the CREATE TABLE
statement, you also need to define a model’s attributes in Sequelize Model.init()
call.
For example, the following code creates a Model
named City
:
const { Model, Sequelize } = require("sequelize");
const City = Model.init(
{
cityName: Sequelize.STRING,
},
{ sequelize, modelName: "City", timestamps: false }
);
Or you can also extend the Model
class as follows:
class City extends Model {}
City.init(
{
cityName: Sequelize.STRING,
},
{ sequelize, timestamps: false }
);
The init()
method requires you to pass a sequelize
instance where the Model
will be attached to.
You also need to provide the modelName
option to name the model when you’re not extending the Model
class.
A Sequelize Model
can also be initialized using the sequelize.define()
method as follows:
const City = sequelize.define(
"City",
{ cityName: Sequelize.STRING },
{ timestamps: false }
);
When using sequelize.define()
, the created Model
will be automatically attached to the sequelize
instance where you call the method.
Both Model.init()
and sequelize.define()
methods are equivalent. The only difference is that one follows the object-oriented programming pattern and the other follows the functional programming pattern.
You can learn more from Sequelize Model Definition documentation.