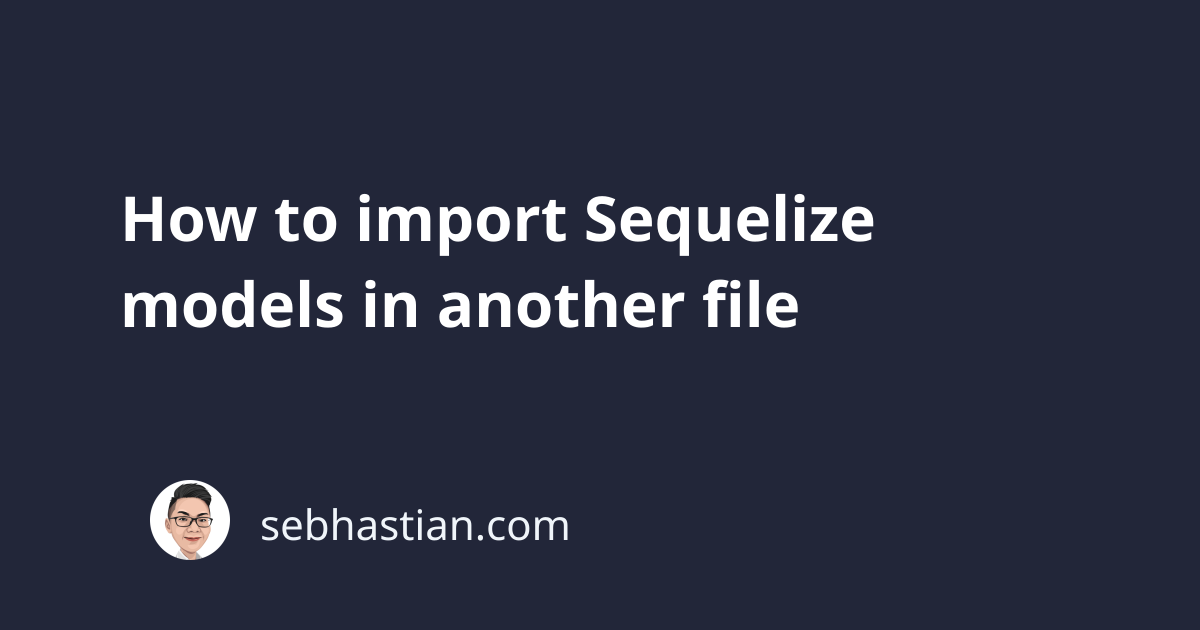
Since Sequelize is a JavaScript library, you can organize your project and put Sequelize models in their own folder.
Here’s an example of your JavaScript project structure that you can follow:
src
├── index.js
└── models
└── User.js
The structure above means that the Sequelize models are stored inside the models/
folder and need to be imported by the index.js
file.
To import Sequelize models, you need to export the model as a module first.
Suppose the User.js
file contains the User
model as follows:
const { Sequelize } = require("sequelize");
module.exports = (sequelize) => {
const User = sequelize.define("User", {
firstName: {
type: Sequelize.STRING,
},
lastName: {
type: Sequelize.STRING,
},
},
{
timestamps: false,
});
return User;
};
Now that you have the User
model exported as a module, you can import it from index.js
file like this:
const { Sequelize } = require('sequelize')
const sequelize = new Sequelize('database', 'username', 'password', {
host: 'localhost',
dialect: /* one of 'mysql' | 'mariadb' | 'postgres' | 'mssql' */
});
const User = require(`${__dirname}/models/user`)(sequelize)
User.findAll().then((users) => {
console.log(users)
sequelize.close()
})
The code above creates a new instance of the Sequelize
class to connect to your database. The sequelize
instance needs to be passed into the user.js
model file when you call the require()
function.
Notice the sequelize
variable passed inside the second parentheses below:
const User = require(`${__dirname}/models/user`)(sequelize);
In the model file, the same sequelize
instance is used to define the model:
module.exports = (sequelize) => {
const User = sequelize.define("User", {
// ...
});
return User;
};
And that’s how you can import Sequelize models in your JavaScript project.
Using Sequelize import instead of require
Additionally, Sequelize has the import()
function which is used for importing models from a file.
const User = sequelize.import(`${__dirname}/models/user`);
This function is already deprecated in Sequelize version 6, so JavaScript will throw the sequelize.import is not a function
error when you run the code.
If you’re using a JavaScript environment that doesn’t support require()
function, then you need to use Sequelize version < 6.0.0
to use the import()
function.
Generating models using sequelize-cli
Finally, the sequelize-cli
command line tool is available for you to use when you need to structure a new JavaScript project with Sequelize in mind.
When you run the init
command, the tool will generate the following folders in your current working directory:
config/
- contains config file that takes your environment into account (production
ordevelopment
)models/
- contains all models for your projectmigrations/
- contains all migration files for you to runseeders/
- contains all seed files
In the generated models/
folder, there’s an index.js
file that imports all files created in the models/
folder.
With the help of this index.js
file, you can import any models that you create by simply importing the right Model
name.
For example, here’s how to import the User
model:
const { User } = require("./models");
When you have many models, you can import them all in one line as follows:
const { User, Project } = require("./models");
By using sequelize-cli
, you don’t need to require your models one by one.
For instructions on how to generate models and migrations using the sequelize-cli
tool, you can visit the sequelize-cli documentation here.
Now you’ve learned how to import Sequelize models created as modules. Nice work! 👍