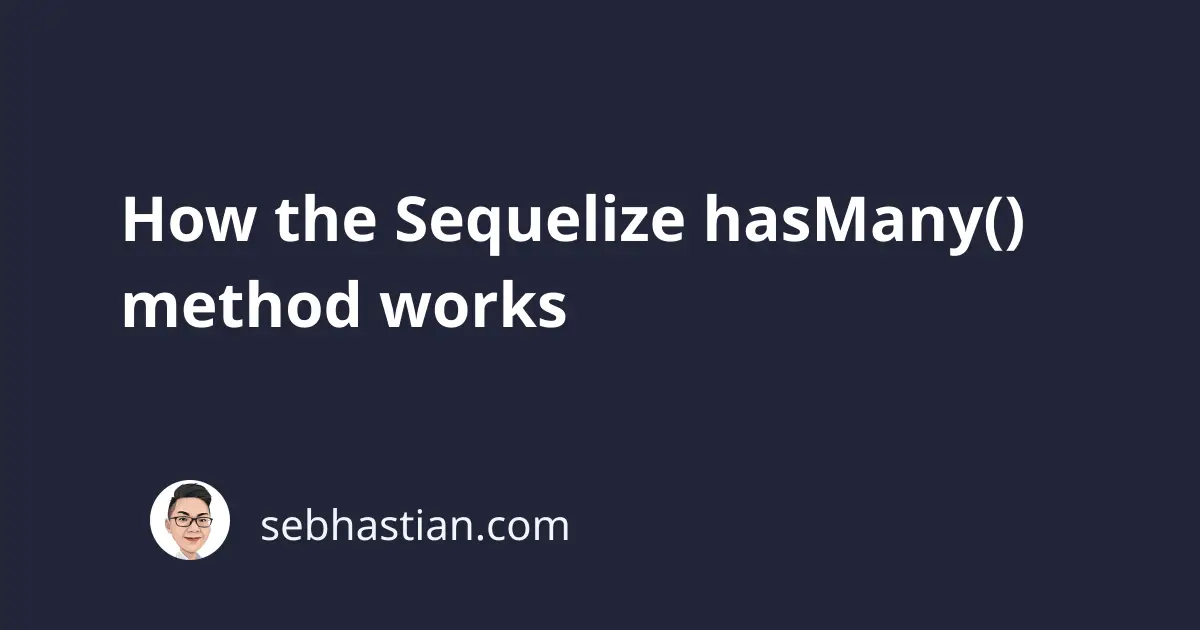
The Sequelize hasMany()
method is used to create a One-To-Many association between two Sequelize models.
For example, suppose you have SQL tables named Countries
and Cities
in your database.
These two tables are related where one country row can have many city rows.
The sample data for the tables are as follows:
# Countries
+----+----------------+
| id | countryName |
+----+----------------+
| 1 | United Kingdom |
+----+----------------+
# Cities
+----+----------+-----------+
| id | cityName | CountryId |
+----+----------+-----------+
| 1 | York | 1 |
| 2 | Bristol | 1 |
| 3 | London | 1 |
| 4 | Glasgow | 1 |
+----+----------+-----------+
To create the relation in Sequelize, you need to create the models representing the tables first:
const City = sequelize.define(
"City",
{ cityName: Sequelize.STRING },
{ timestamps: false }
);
const Country = sequelize.define(
"Country",
{ countryName: Sequelize.STRING },
{ timestamps: false }
);
With the models defined, you can call the hasMany()
method from the model that can have many rows of the other model.
In this case, it’s the Country
model:
Country.hasMany(City);
The code above will add the CountryId
attribute to the City
model.
Now when you query data from the Country
model, you can add the include
option to also retrieve the City
model data.
Here’s an example of retrieving both models data:
const data = await Country.findByPk(1, {
include: City,
});
console.log(data.toJSON());
The console log will output the following data:
{
id: 1,
countryName: 'United Kingdom',
Cities: [
{ id: 1, cityName: 'York', CountryId: 1 },
{ id: 2, cityName: 'Bristol', CountryId: 1 },
{ id: 3, cityName: 'London', CountryId: 1 },
{ id: 4, cityName: 'Glasgow', CountryId: 1 }
]
}
But keep in mind that you can’t query the data of the Country
model from the City
model like this:
const data = await City.findByPk(1, {
include: Country,
});
This is because the Country
model is not associated with the City
model in Sequelize.
You need to call the belongsTo()
method from the City
model as shown below:
Country.hasMany(City);
City.belongsTo(Country);
Now you can query for the Country
data from the City
model:
{
id: 1,
cityName: 'York',
CountryId: 1,
Country: { id: 1, countryName: 'United Kingdom' }
}
And that’s how the Sequelize hasMany()
method works.