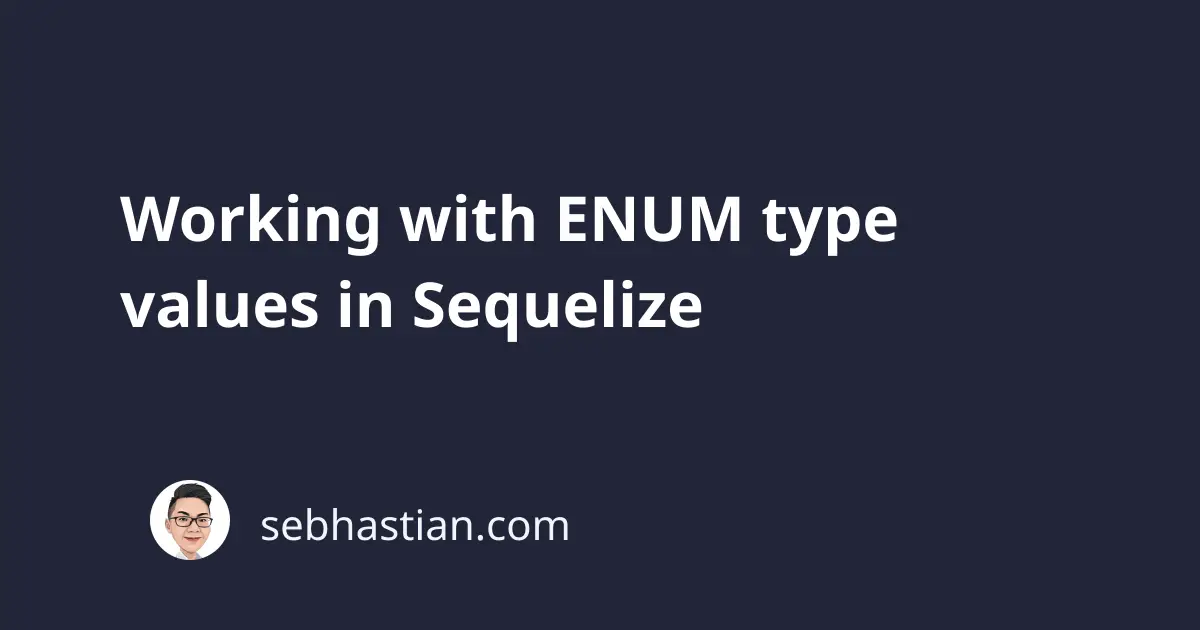
Sequelize provides the ENUM
data type that you can use to create and interact with SQL tables that has ENUM
as its column type.
To use the ENUM
type, you need to use either Sequelize.ENUM
or DataTypes.ENUM
as the type of your model attribute.
In the example below, the User
model has the status
attribute that’s an ENUM
type:
const User = sequelize.define(
"User",
{
firstName: Sequelize.STRING,
status: {
type: Sequelize.ENUM("pending", "active", "disabled"),
},
},
{
timestamps: false,
}
);
The ENUM
values of pending
, active
, and disabled
are passed as arguments to the ENUM()
function.
When you need to set a default value, you can add the defaultValue
option to the attribute as shown below:
const User = sequelize.define(
"User",
{
firstName: Sequelize.STRING,
status: {
type: Sequelize.ENUM("pending", "active", "disabled"),
defaultValue: "pending",
},
},
{
timestamps: false,
}
);
If you need the ENUM
values of your attribute, you can call the getAttributes()
method to get the attributes of your model.
The ENUM
values can be accessed from the values
property of the returned attributes object:
console.log(User.getAttributes().status.values);
The output of the log above will be as follows:
[ 'pending', 'active', 'disabled' ]
You need to change the status
property in getAttributes().status
to the name of your model attribute.
Now you’ve learned how to use and interact with ENUM
type values in Sequelize. Nice work! 👍