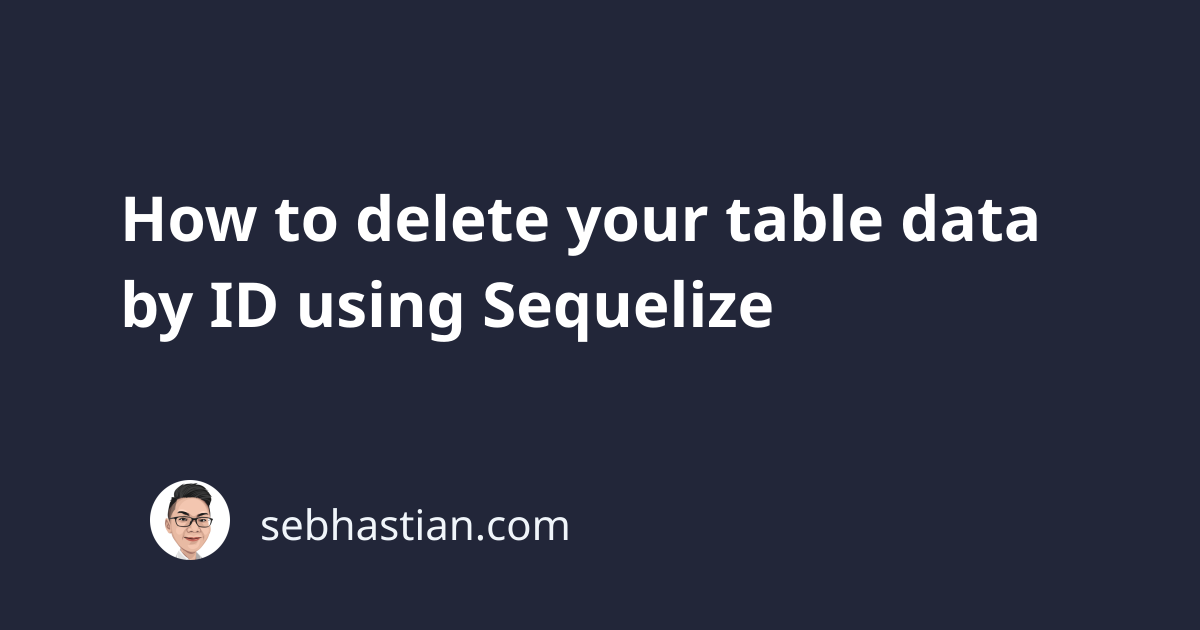
Sequelize provides a Model.destroy()
method that you can use to delete rows of data from your database table.
The method requires the where
option to be passed so that it knows which row(s) you want to delete from the table.
First, you need to create a Sequelize model of your existing SQL table.
Suppose you have a table named Invoices
with the following data:
+----+-------+
| id | total |
+----+-------+
| 1 | 300 |
| 2 | 150 |
| 3 | 100 |
+----+-------+
In the example below, the model Invoice
is defined that corresponds to the Invoices
table:
const Invoice = sequelize.define(
"Invoice",
{ amount: Sequelize.INTEGER },
{ timestamps: false }
);
By default, Sequelize will assume the table uses an id
column as its primary key.
Now you can call destroy()
from the Invoice
model to delete a row by its id
value:
const count = await Invoice.destroy({ where: { id: 2 } });
console.log(`deleted row(s): ${count}`);
The destroy()
method will return a number representing the number of row(s) deleted from the table. It will be 1
for the example above.
The table data will now look as follows:
+----+-------+
| id | total |
+----+-------+
| 1 | 300 |
| 3 | 100 |
+----+-------+
And that’s how you can delete a table row by its id
value using Sequelize 😉