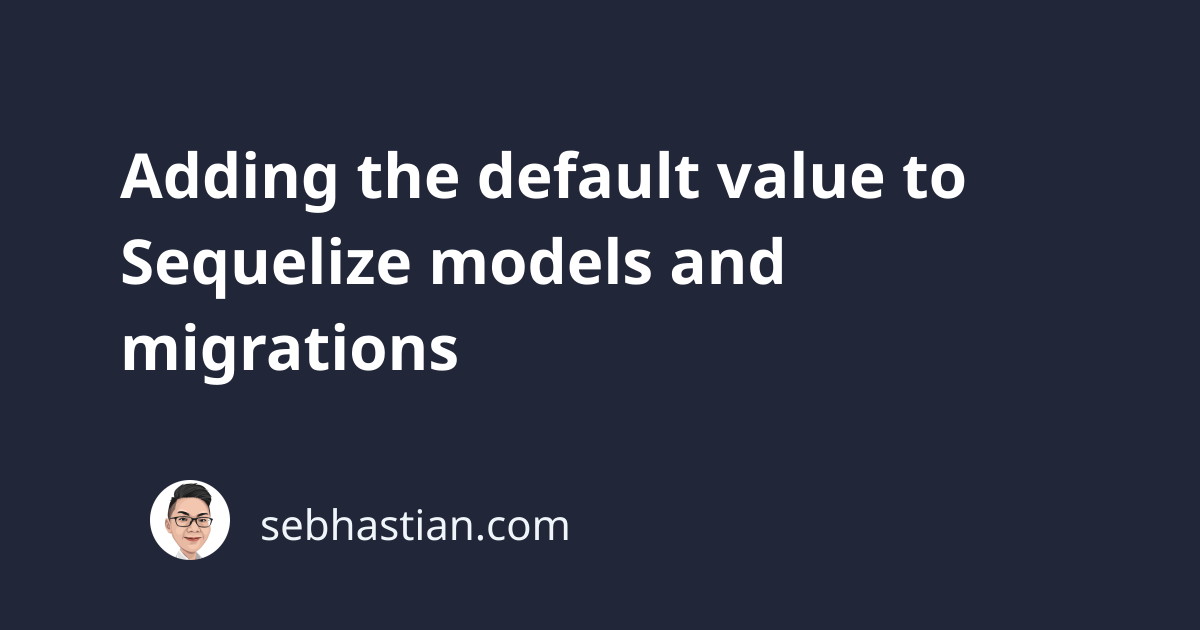
When you create a Sequelize model, you can add the default value for your model by adding the defaultValue
option to the column(s) definition.
The defaultValue
option will be used by Sequelize to define default value(s) for your SQL column(s) when you create a table using Sequelize.
For example, when you sync()
the following Sequelize model:
const User = sequelize.define("User", {
firstName: {
type: Sequelize.STRING,
},
lastName: {
type: Sequelize.STRING,
defaultValue: "Doe",
},
},
{
timestamps: false,
});
User.sync();
Then the Users
table will be created in your database with the following definition. Notice how the default value of the lastName
column is Doe
:
+-----------+--------------+------+-----+---------+----------------+
| Field | Type | Null | Key | Default | Extra |
+-----------+--------------+------+-----+---------+----------------+
| id | int | NO | PRI | NULL | auto_increment |
| firstName | varchar(255) | YES | | NULL | |
| lastName | varchar(255) | YES | | Doe | |
+-----------+--------------+------+-----+---------+----------------+
The same goes when you create a table using Sequelize migrations. You can add the column’s default value as follows:
"use strict";
module.exports = {
up: async (queryInterface, Sequelize) => {
await queryInterface.createTable("Users", {
id: {
allowNull: false,
autoIncrement: true,
primaryKey: true,
type: Sequelize.INTEGER,
},
firstName: {
type: Sequelize.STRING,
},
lastName: {
type: Sequelize.STRING,
defaultValue: "Doe",
},
createdAt: {
allowNull: false,
type: Sequelize.DATE,
},
updatedAt: {
allowNull: false,
type: Sequelize.DATE,
},
});
},
down: async (queryInterface, Sequelize) => {
await queryInterface.dropTable("Users");
},
};
Sequelize defaultValue option behavior
Additionally, the defaultValue
option will still take effect even when your table column has NULL
as its default value.
For example, suppose you have a MySQL table named Users
with the following structure:
+-----------+--------------+------+-----+---------+----------------+
| Field | Type | Null | Key | Default | Extra |
+-----------+--------------+------+-----+---------+----------------+
| id | int | NO | PRI | NULL | auto_increment |
| firstName | varchar(255) | YES | | NULL | |
| lastName | varchar(255) | YES | | NULL | |
+-----------+--------------+------+-----+---------+----------------+
In the description above, notice how the lastName
column of the Users
table defaults to NULL
value.
Next, you create a Sequelize model as follows:
const User = sequelize.define("User", {
firstName: {
type: Sequelize.STRING,
},
lastName: {
type: Sequelize.STRING,
defaultValue: "Doe",
},
},
{
timestamps: false,
});
With the defaultValue
option added to the lastName
column, Sequelize will use that value when you insert a new row with the create()
method:
await User.create({
firstName: "Nathan",
});
The above code will insert a row into your table as shown below:
+----+-----------+----------+
| id | firstName | lastName |
+----+-----------+----------+
| 1 | Nathan | Doe |
+----+-----------+----------+
As you can see, even when the MySQL column definition defaults to NULL
, the defaultValue
option added to the Sequelize model is still taken into account.
The defaultValue
option also takes priority over the default value defined in your MySQL table.
In the following example, the Sequelize model defines the lastName
column defaultValue
as Sebhastian
:
const User = sequelize.define(
"User",
{
firstName: {
type: Sequelize.STRING,
},
lastName: {
type: Sequelize.STRING,
defaultValue: "Sebhastian",
},
},
{
timestamps: false,
}
);
Even when the MySQL table has the lastName
column that defaults to Doe
:
+-----------+--------------+------+-----+---------+----------------+
| Field | Type | Null | Key | Default | Extra |
+-----------+--------------+------+-----+---------+----------------+
| id | int | NO | PRI | NULL | auto_increment |
| firstName | varchar(255) | YES | | NULL | |
| lastName | varchar(255) | YES | | Doe | |
+-----------+--------------+------+-----+---------+----------------+
In the case above, Sebhastian
will be used as the default value when you insert a new row instead of Doe
.
Now you’ve learned how to add default values to Sequelize models and migrations. Nice work! 👍