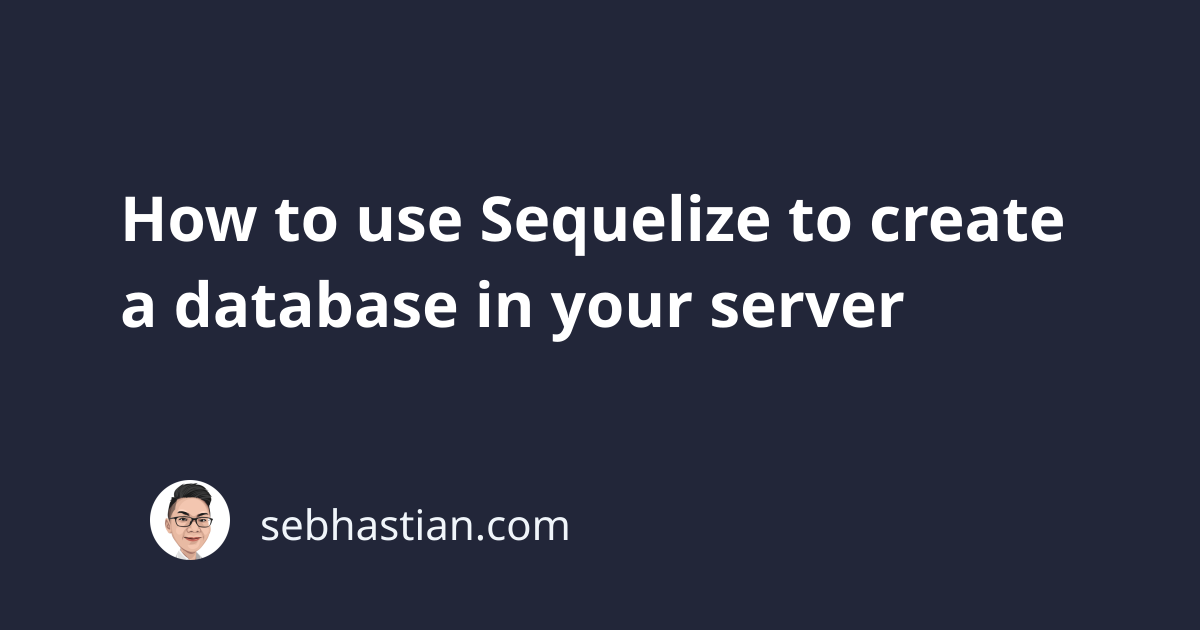
Each Sequelize ORM instance initializes a new connection to your database server with a specific database name.
This means you must already have the database exists in your server before opening a connection with Sequelize.
To create a database, you need to use the client application of your database vendor, like the mysql
command-line client for MySQL database.
If you really need to create a database using JavaScript, then you need to use the Node SQL driver specific for your database vendor.
These drivers are also used by Sequelize under the hood:
pg
andpg-hstore
for PostgreSQLmysql2
for MySQLmariadb
for MariaDBsqlite3
for SQLitetedious
for Microsoft SQL Serveribm_db
for DB2
If you use the MySQL database, then you can open a connection and run the CREATE DATABASE
statement using the mysql2
library.
The following example shows how to connect to your MySQL server using root
credentials and create a database named BlogDB
:
const mysql = require("mysql2");
// Open the connection to MySQL server
const connection = mysql.createConnection({
host: "localhost",
user: "root",
password: "root",
});
// Run create database statement
connection.query(
`CREATE DATABASE IF NOT EXISTS BlogDB`,
function (err, results) {
console.log(results);
console.log(err);
}
);
// Close the connection
connection.end();
Once you have the database, you can run the Sequelize code to connect to the database as usual.
const { Sequelize } = require("sequelize");
const sequelize = new Sequelize("BlogDB", "root", "root", {
host: "localhost",
dialect: "mysql",
});
After that, you can create the Sequelize models to represent the tables in your database.
You can also let Sequelize create the tables in your database using the Model.sync()
method.
Although you can’t use Sequelize to create your database, the underlying drivers that Sequelize uses to connect to your database can be used to send CREATE DATABASE
statements.
Here’s another example of using pg
library for creating a database in PostgreSQL:
const { Client } = require("pg");
const client = new Client({
user: "nsebhastian",
password: "mypassword",
host: "localhost",
database: "postgres",
});
client.connect();
client.query('CREATE DATABASE "BlogDB"', (err, res) => {
console.log(err, res);
client.end();
});
Except for SQLite which has one .db
file for one database, you need to refer to the documentation of the Node drivers specific to your database and find out how to create a database with it.
After that, you can use Sequelize to connect and create tables for storing your data.