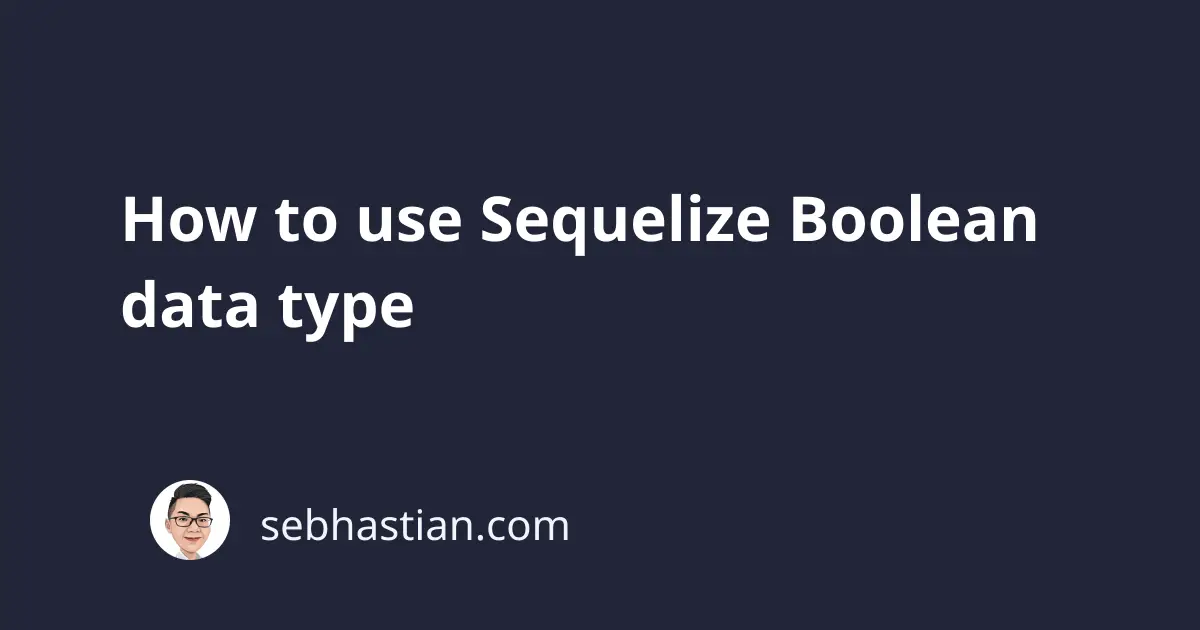
Sequelize has a BOOLEAN
data type that you can use to define model attributes that accept Boolean values.
The following example shows a User
model that has an isActive
attribute with BOOLEAN
type:
const User = sequelize.define("User", {
firstName: {
type: Sequelize.STRING,
},
lastName: {
type: Sequelize.STRING,
},
isActive: {
type: Sequelize.BOOLEAN,
defaultValue: false,
},
},
{
timestamps: false,
});
When you create a table from the model above, Sequelize will generate the isActive
column with TINYINT(1)
type for MySQL and SQLite or BOOLEAN
type for PostgreSQL.
In MySQL and SQLite, BOOLEAN
types use integer 1
as true
and 0
as false
.
Once the table and the model are synchronized, you can insert values to the column with JavaScript boolean literal true
or false
.
Here’s how to insert new values into the model:
User.bulkCreate([
{ firstName: "Nathan", lastName: "Sebhastian", isActive: true },
{ firstName: "John", lastName: "Doe" },
]);
When you omit the isActive
value from create()
or bulkCreate()
method, then the value will default to false
as defined in the model.
The Users
table data would look as follows in MySQL:
+----+-----------+------------+----------+
| id | firstName | lastName | isActive |
+----+-----------+------------+----------+
| 1 | Nathan | Sebhastian | 1 |
| 2 | John | Doe | 0 |
+----+-----------+------------+----------+
For PostgreSQL, the table would look as follows:
id | firstName | lastName | isActive
----+-----------+------------+----------
1 | Nathan | Sebhastian | t
2 | John | Doe | f
----+-----------+------------+----------
And that’s how you use the BOOLEAN
data type in Sequelize.
Keep in mind that when you create your table without using Sequelize, then you need to make sure that the BOOLEAN
column uses TINYINT(1)
type for MySQL and SQLite.