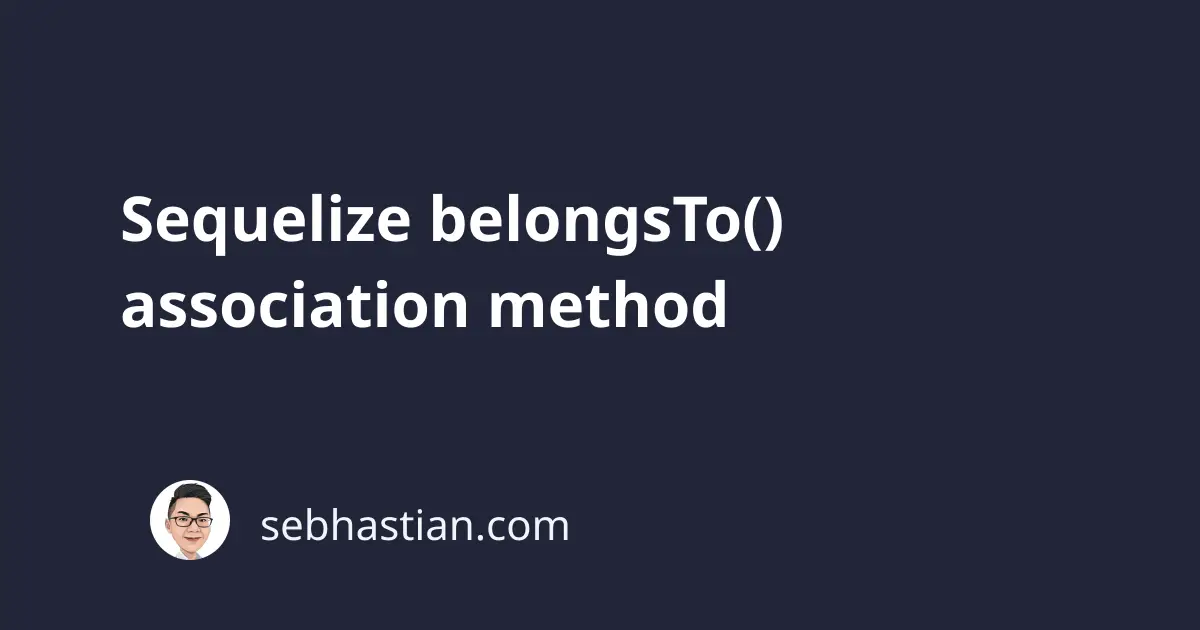
The Sequelize belongsTo()
method allows you to create a One-To-One (1:1
) relationship between two Sequelize models.
The method is called from a source model and pointed towards the model passed as its argument.
For example, suppose you have a User
and Task
models defined like this:
const User = sequelize.define(
"User",
{ firstName: Sequelize.STRING },
{ timestamps: false }
);
const Task = sequelize.define(
"Task",
{ taskName: Sequelize.STRING },
{ timestamps: false }
);
The defined models above will represent the Users
and Tasks
tables respectively. This is because Sequelize uses the plural forms of the model names when looking for the tables by default.
You can make the Task
model belongs to the User
model by calling the belongsTo()
method from the Task
model like this:
Task.belongsTo(User);
The belongsTo()
method above will associate the Task
model with the User
model, adding the UserId
attribute to the Task
model as the foreign key constraint.
But keep in mind that you need to add the UserId
column manually to your SQL table to make the association works.
You can let Sequelize alter the tables for you by calling the sync()
method on the Task
model as follows:
await Task.sync({ alter: true });
With that, the Tasks
table in your database should have the UserId
column and the foreign key constraint.
The belongsTo()
method also provide several options to let you modify the relationship details:
Task.belongsTo(User, {
foreignKey: "myUserId", // change column name
targetKey: "firstName", // change the referenced column
uniqueKey: "task_user_fk", // foreign key constraint name
onDelete: "SET DEFAULT", // ON DELETE config
onUpdate: "SET DEFAULT", // ON UPDATE config
constraints: false, // remove ON DELETE and ON UPDATE constraints
});
Keep in mind that the column referenced as the targetKey
must be indexed. Else, you will get an error saying SQL failed to add foreign key constraint as shown below:
Error {
code: 'ER_FK_NO_INDEX_PARENT',
errno: 1822,
sqlState: 'HY000',
sqlMessage: "Failed to add the foreign key constraint...",
}
For more information, you can view the Sequelize belongsTo documentation
Now you’ve learned how the Sequelize belongsTo()
method works. Way to go! 😉