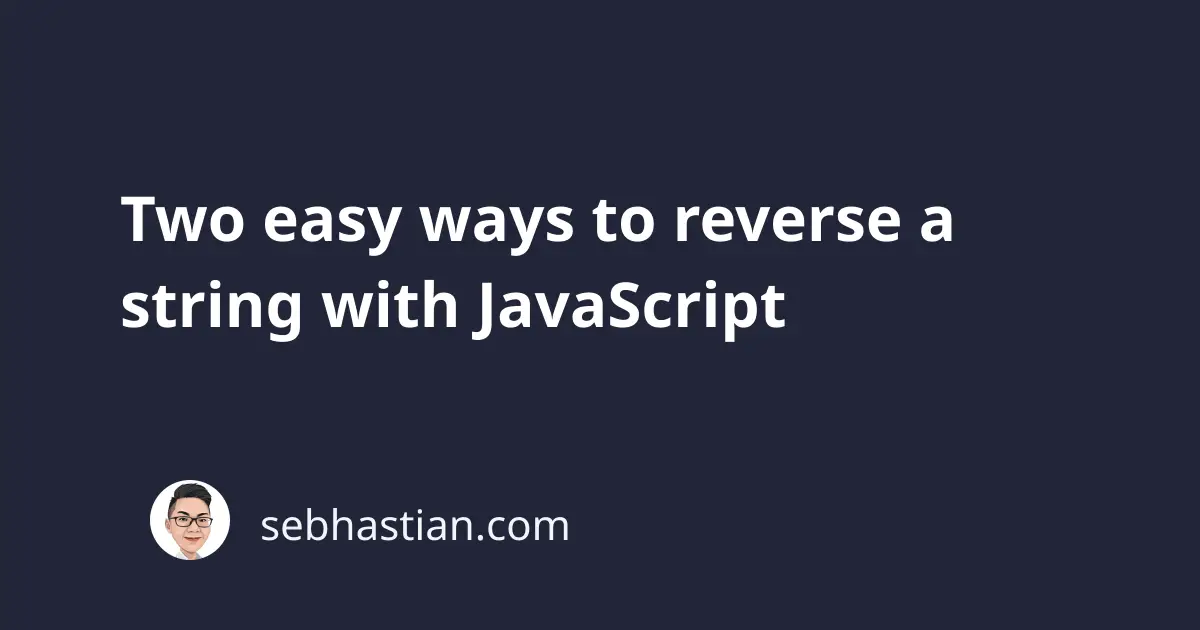
Reversing a string is one of the most common programming exercises, and since JavaScript String
object doesn’t have the reverse()
method, you need to create a work around to get the job done.
There are two easy ways you can reverse a string:
- Transform the string to array and call the
Array.reverse()
method - Use a
for
loop statement and create a new reversed string from the original string
This tutorial will help you learn how to do both. Let’s start with transforming the string to an array.
Reverse a string using Array reverse() method
You can reverse a string by first transforming that string to an array with String.split()
method.
The String.split()
method is a built-in method of the String
object that allows you to split a string into several array elements.
When you pass an empty string (""
) as an argument to the method, it will return an array with each character as a separate element.
let str = "Hello";
console.log(str.split("")); // ["H", "e", "l", "l", "o"]
Once you have the string split into an array, call the Array.reverse()
method on the array to reverse the elements:
let str = "Hello";
console.log(str.split("").reverse()); // ["o", "l", "l", "e", "H"]
Next, call the Array.join()
method so that the array will be transformed back to string.
You need to pass an empty string as an argument to the join()
method or the string will be concatenated with a comma:
let str = "Hello";
console.log(str.split("").reverse().join("")); // "olleH"
You can reverse any kind of string using the combination of split()
, reverse()
, and join()
methods.
Reverse a string using a for loop
To reverse a string using a for
loop, you need to use the string length - 1
as the initial expression value and decrement the loop value in each iteration as long as it’s greater than or equal to zero:
for (let i = str.length - 1; i >= 0; i--)
Next, create a new empty string and add each character from the original string backwards using its index.
The following code example shows how you do it:
let str = "hello";
let reverseStr = "";
for (let i = str.length - 1; i >= 0; i--) {
reverseStr += str[i];
}
console.log(reverseStr); // "olleh"
And that’s how you can reverse a string using a for
loop statement.