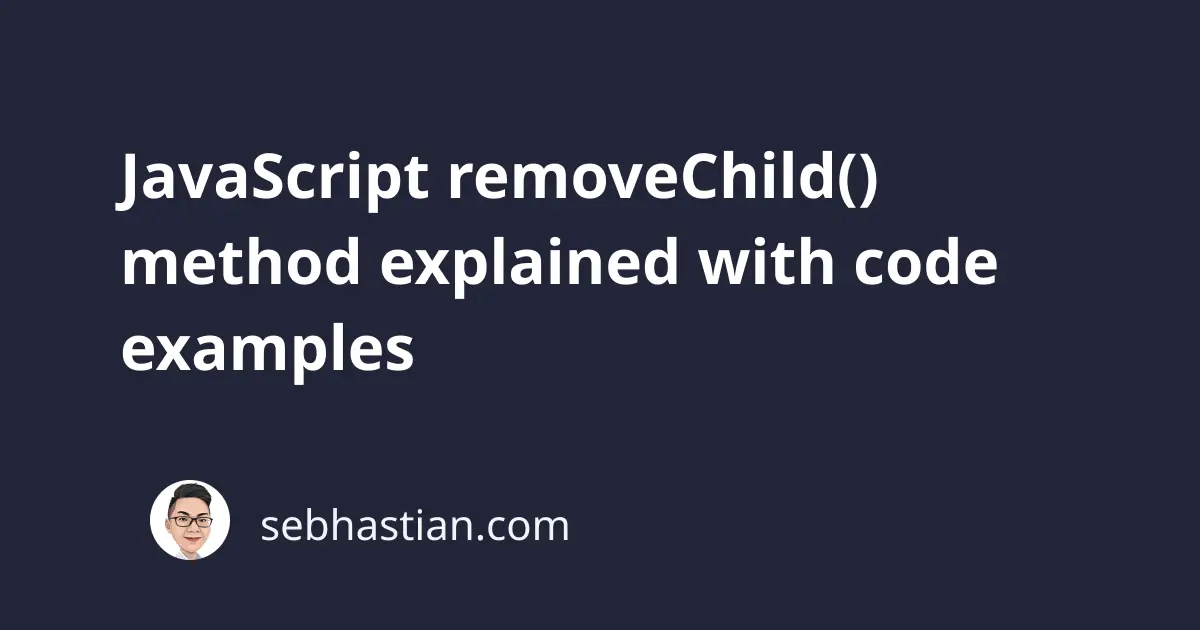
The JavaScript removeChild()
method allows you to remove a child node (element) from an existing element in the DOM (Document Object Model).
The method is defined in the JavaScript Node
interface. The method syntax is as follows:
Node.removeChild(childNode);
The childNode
parameter will be the element you want to remove from the DOM.
The Node
object will be the parent element of the childNode
.
For example, suppose you have a nested <div>
element in your HTML document as shown below:
<div id="parent">
<div id="child">
<h1>Hello World!</h1>
</div>
</div>
You can remove the child
div element by calling the removeChild()
method from the parent
element.
Consider the following JavaScript code:
let parent = document.getElementById("parent");
let child = document.getElementById("child");
parent.removeChild(child);
Running the above code will create the following output:
<div id="parent"></div>
First, you need to select both the parent element and the child element that you want to remove.
Next, you need to call the removeChild()
method from the parent element, passing the child
element as an argument to the method.
Any element nested inside the child
element will be removed altogether.
You can also remove a specified element without knowing its parent node by using the parentNode
property of the Node
object.
For example, suppose you have the following tree structure in your HTML document:
<body>
<div id="main"></div>
</body>
To remove the main
div, you can select the div and get its parent element reference saved in the parentNode
property:
let main = document.getElementById("main");
console.log(main.parentNode);
// remove main
main.parentNode.removeChild(main);
The main
div will be removed, leaving only the <body>
tag:
<body></body>
The method also returns the removed element.
You are free to keep or discard the returned element in a variable:
let parent = document.getElementById("parent");
let child = document.getElementById("child");
let removedNode = parent.removeChild(child);
console.log(removedNode); // equals child variable
When you have multiple child elements, you can use the while
statement to remove the child elements from the parent.
For example, suppose you have a <ul>
list element as shown below:
<ul id="list">
<li>HTML</li>
<li>CSS</li>
<li>JavaScript</li>
</ul>
Using the while
statement, you can all <li>
elements nested inside the <ul>
element as shown below:
let list = document.getElementById('list');
while (list.firstChild) {
list.removeChild(list.firstChild);
}
Only the list
ul element will be left after the above code has been executed:
<ul id="list">
</ul>
You can also use the bracket notation of the children
property to remove a specific element from the parent node:
let list = document.getElementById('list');
list.removeChild(list.children[1]); // <li>CSS</li>
list.removeChild(list.children[0]); // <li>HTML</li>
To remove the last child element, use the lastElementChild
property from the parent node:
list.removeChild(list.lastElementChild); // <li>JavaScript</li>
The lastElementChild
and firstElementChild
properties return the last and first child node while ignoring any text node or comment node on the list.
The lastChild
and firstChild
properties will return any text node or comment node that exists in your node.
It’s fine to use the lastChild
and firstChild
properties in a while
loop as shown above. But if you want to remove the individual element on the first or last position, use the lastElementChild
and firstElementChild
instead.
Now you’ve learned how the JavaScript removeChild()
method and see some examples of how to use the method. Good work! 👍