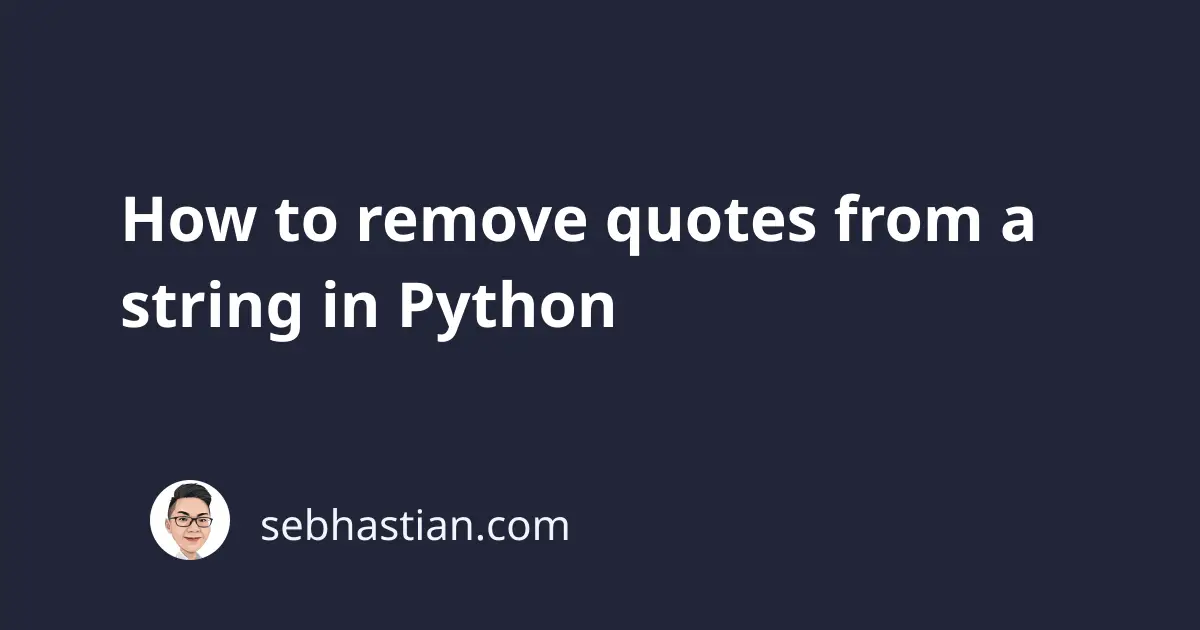
In my experience, I found there are two easy ways to remove quotes from a string in Python:
- Using the
str.replace()
method - Using the
re
modulesub()
function
This tutorial will show you how to use these methods in practice.
1. Use the str.replace() method
The str.replace()
method is used to replace all occurrences of a substring with a new string.
Suppose you have a string with quotations, you can use this method to remove those quotations as shown below:
my_str = 'Hello "there"! How are" you?'
new_str = my_str.replace('"', '')
print(my_str)
print(new_str)
Output:
Hello "there"! How are" you?
Hello there! How are you?
As you can see, the double quotes in the string is cleared using the str.replace()
method.
This method also works when your string uses the escape character \
to escape the quotes:
my_str = "Hello \"there\"! How are\" you?"
new_str = my_str.replace("\"", "")
print(my_str)
print(new_str)
The output will be the same as in the previous code. If you have both double and single quotes in your string, then you can call the replace()
method twice in successions.
Consider the example below:
my_str = "Hello' \"there\"! How' are\" you?"
str_1 = my_str.replace("\"", "")
str_2 = str_1.replace("'", "")
print(my_str)
print(str_1)
print(str_2)
Output:
Hello' "there"! How' are" you?
Hello' there! How' are you?
Hello there! How are you?
After calling replace()
from the original string, you need to call it again from the modified string to clear the single quotes left behind.
2. Use the sub() function from re module
The regular expression re
module is a built-in Python module that contains the sub()
function.
The sub()
function is short for “substitute”, and you can use it to replace all occurrences of a substring with a new string as shown below:
from re import sub
my_str = "Jack \"and\" Jill are \"siblings"
new_str = sub("\"", "", my_str)
print(my_str)
print(new_str)
Output:
Jack "and" Jill are "siblings
Jack and Jill are siblings
As you can see, the sub()
function works as well as the replace()
method.
Unless you have a specific reason, I say using the replace()
method should be enough.
I hope this tutorial helps. See you in other tutorials! 🙌