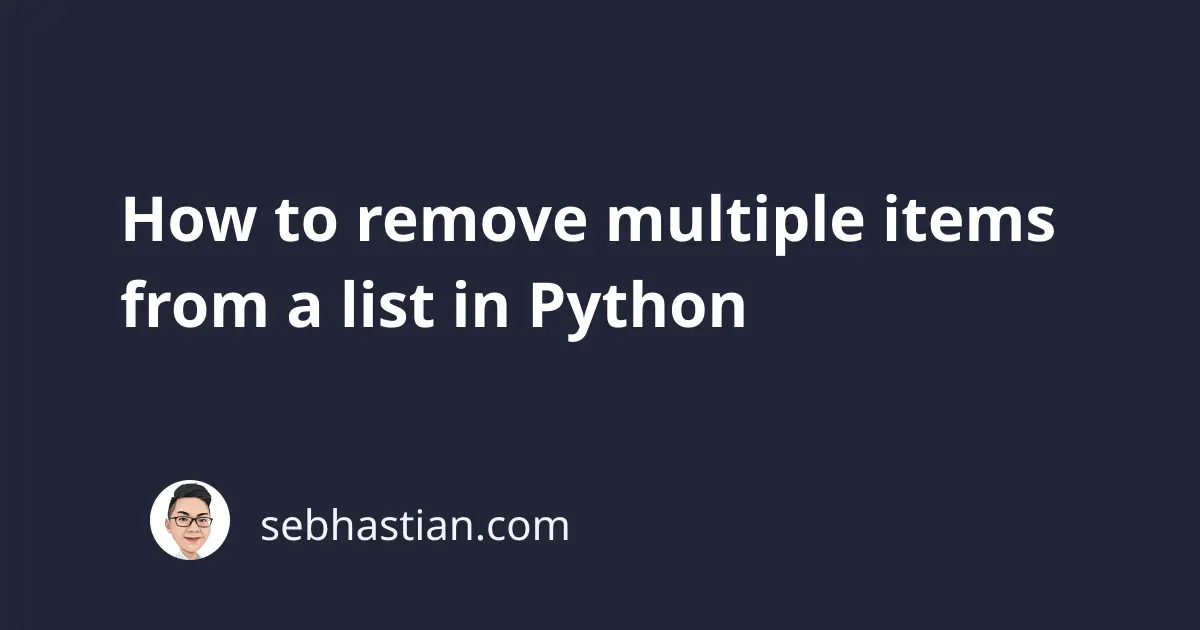
Depending on the items you want to remove, there are 3 ways you can remove multiple items from a list in Python:
- Using a slice
- Using a list comprehension and a second list
- Using the
clear()
method
This tutorial will show you how to use the three methods above in practice.
1. Using a slice
When you need to remove multiple items from a list, you can use a slice to remove a portion of the list from a specific index.
The slice syntax is as follows:
list_object[start_index:end_index]
The start_index
is the starting position of the slice and end_index
is the ending position of the slice. The two are separated using a colon (:
) operator.
When start_index
is not specified, it is assumed to be 0. If end_index
is not specified, it is assumed to be the length of the list itself.
The start_index
is included in the output, but the end_index
is excluded.
For example, suppose you want to remove the first three items from a list:
my_list = [1, 2, 3, 4, 5]
new_list = my_list[3:]
print(new_list) # [4, 5]
Or if you want to remove the last two items from a list:
my_list = [1, 2, 3, 4, 5]
new_list = my_list[:3]
print(new_list) # [1, 2, 3]
The end_index
is 3, and the element at index 3 is 4. The example list has 5 items, so the index starts from 0 and ends at 4.
This slice [:3]
causes elements at index 3 and 4 to be excluded from the result.
This method is useful when you want to remove consecutive elements from a list. But if you want to remove elements at random positions from the list, you need to use the next method.
2. Using a list comprehension and a second list
When you need to remove elements at random positions, you need to create a second list that acts as the filter and use that list in a list comprehension.
First, add all elements you want to remove in the second list:
my_list = [1, 2, 3, 1, 6, 7, 2]
second_list = [1, 2, 7] # elements to remove
Next, you need to write a list comprehension and place a condition so that only elements not in the second list will be included in the new list:
my_list = [1, 2, 3, 1, 6, 7, 2]
second_list = [1, 2, 7]
new_list = [item for item in my_list if item not in second_list]
print(new_list)
Output:
[3, 6]
Notice that the if
condition filters the item
in the my_list
object using the second_list
.
Only items that are not in
the second list will be included in the result.
3. Using the clear()
method
The list.clear()
method is useful when you need to remove all elements from a list:
my_list = [1, 2, 3, 1, 6, 7, 2]
my_list.clear()
print(my_list) # []
The clear()
method will empty the list, effectively removing all elements in it.
Now you’ve learned how to remove multiple items from a list in Python.
I hope this tutorial is useful. Until next time! 👋