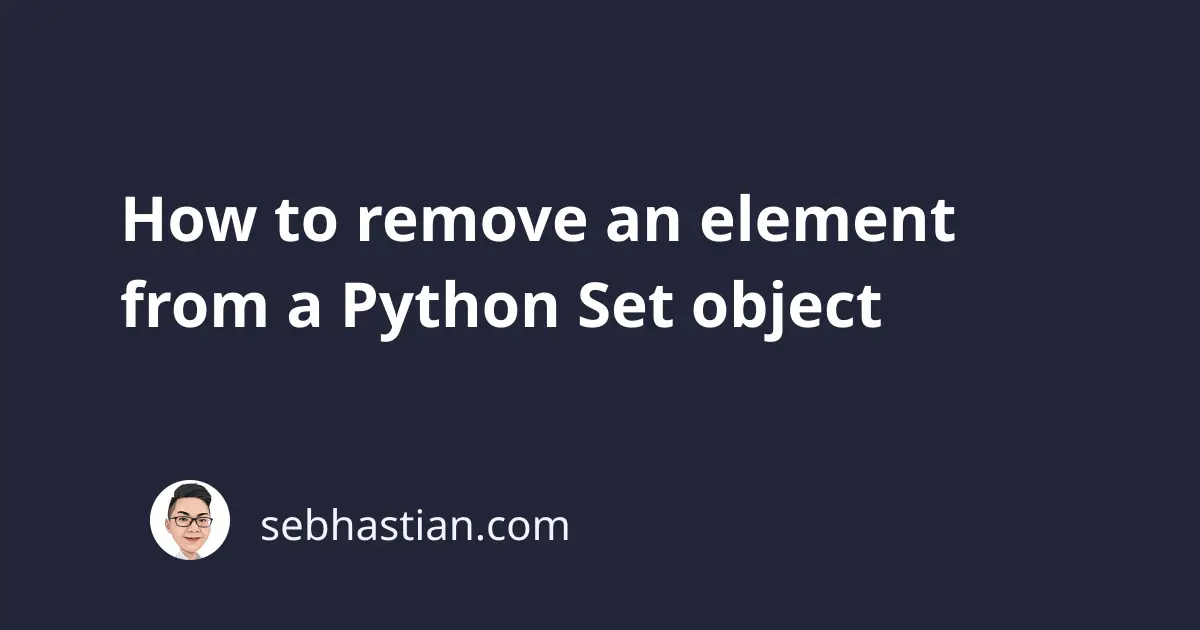
To remove elements from a Set object, you can call the set.remove()
or set.discard()
method.
You need to pass the element you want to remove to one of these methods as shown below:
my_set = {"a", "b", "c", "d"}
my_set.remove("a")
print(my_set)
Output:
{'c', 'b', 'd'}
What’s the difference between remove()
and discard()
? The remove()
method will raise a KeyError
when the element doesn’t exist in the Set object.
Here’s an example of removing an element that doesn’t exist with remove()
:
my_set = {"a", "b", "c", "d"}
my_set.remove("x") # ❌ KeyError: 'x'
print(my_set)
Output:
Traceback (most recent call last):
File "main.py", line 3, in <module>
my_set.remove("x")
KeyError: 'x'
In contrast, the discard()
method does nothing when the element is not found:
my_set = {"a", "b", "c", "d"}
my_set.discard("x") # Nothing happens
print(my_set)
Output:
{'a', 'c', 'b', 'd'}
As you can see, the discard()
method does nothing when the element isn’t found in the set.
This is useful if you don’t want to handle the KeyError
in your source code.
I hope this tutorial is helpful. Happy coding! 😉