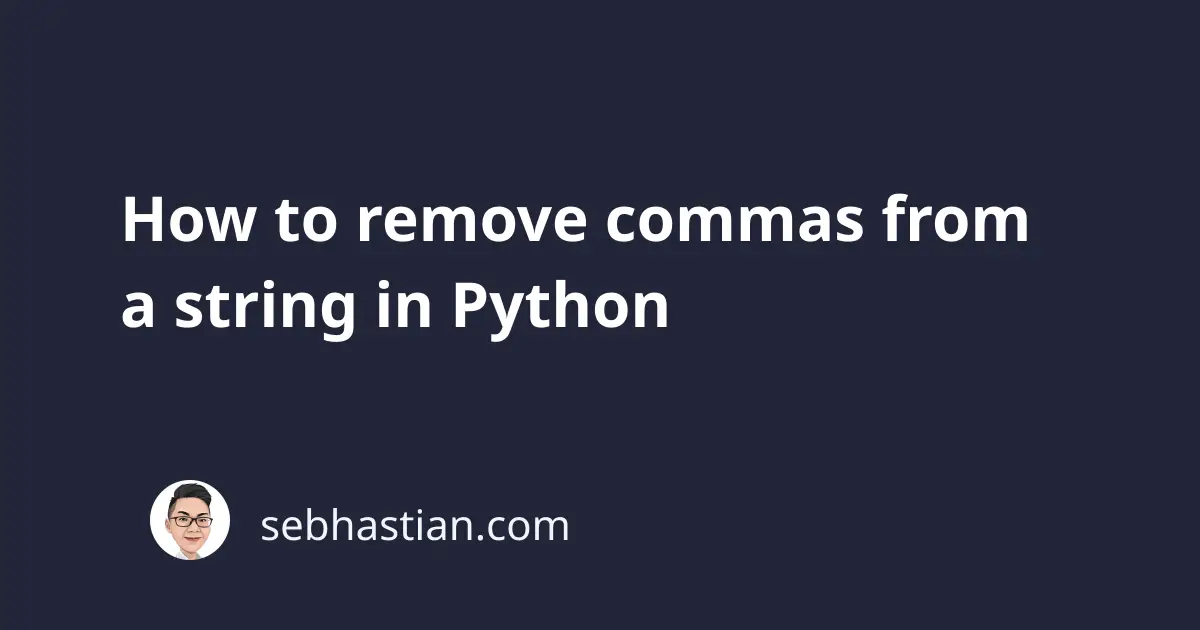
To remove commas from a Python string, you can use the str.replace()
method.
The str.replace()
method is used to replace all occurrences of a substring with a new one.
To remove commas, you can specify an empty string as the new substring as follows:
origin_str = "It, is, a, lovely, day,"
new_str = origin_str.replace(',', '')
print(origin_str) # It, is, a, lovely, day,
print(new_str) # It is a lovely day
As you can see, the commas from origin_str
are removed in new_str
. Note that the str.replace()
method returns a new string instance instead of modifying the original.
If you want to remove a specific number for commas from your string, you can pass a third argument that specifies how many times the old substring will be replaced.
Python searches for the occurrence of the substring from left to right. For example, here’s how to remove only the first occurrence of the comma:
origin_str = "It, is, a, lovely, day,"
new_str = origin_str.replace(',', '', 1)
print(origin_str) # It, is, a, lovely, day,
print(new_str) # It is, a, lovely, day,
In this example, only the first occurrence between It, is
is removed from the string. To remove more, you only need to change the third parameter.
I hope this tutorial is helpful. Happy coding! 🙌