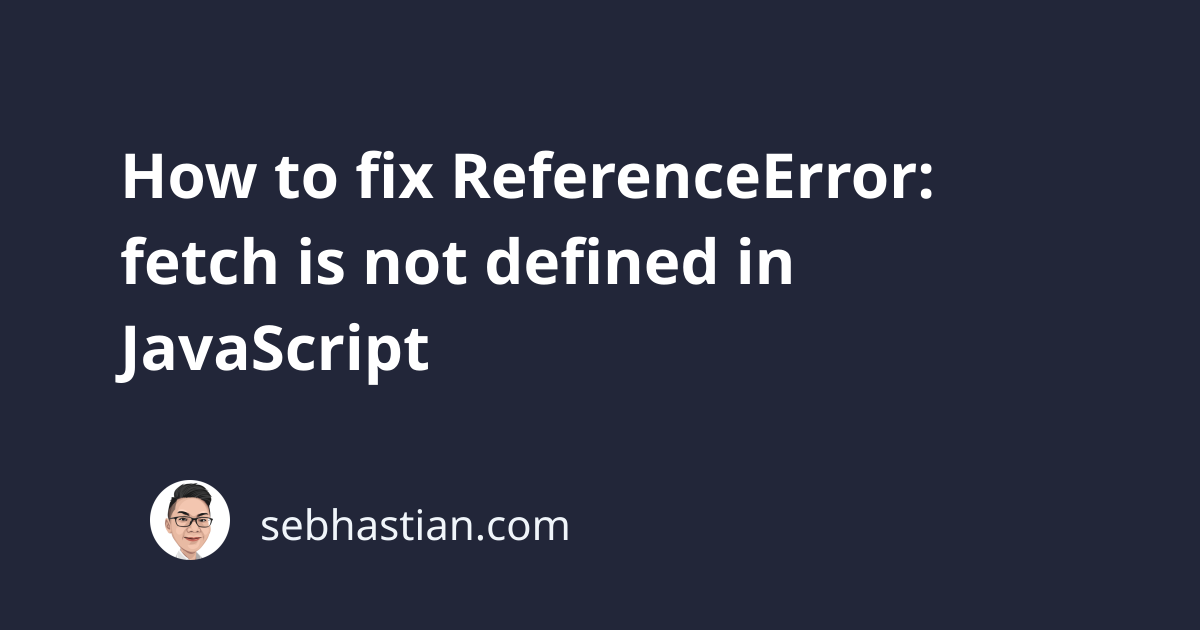
One error you might encounter when running JavaScript code is:
ReferenceError: fetch is not defined
This error occurs when you call the fetch()
function from a JavaScript environment that doesn’t support the fetch API natively.
This usually happens when you run JavaScript code in a Node.js environment.
Let me show you an example that causes this error and how to fix it
How to reproduce the error
Suppose you try to fetch data from an HTTP API using fetch()
as shown below:
fetch("https://api.github.com/users/nsebhastian")
.then(res => {
return res.json();
}).then(data => {
console.log(data);
});
But when you run the code, you get the following error:
fetch("https://api.github.com/users/nsebhastian")
^
ReferenceError: fetch is not defined
This error occurs because fetch
is not defined in the environment running the JavaScript code.
How to fix the error
To resolve this error, you need to add the fetch API into the environment where you run your code.
In Node.js, the fetch API is supported in version 18 and above, so you might need to upgrade the installed package.
Run the node -v
command to check the Node.js version you have installed on your computer.
If it’s less than 18, then you can download and install the newer version from nodejs.org.
If you can’t upgrade Node.js for compatibility reasons, then another way to get the fetch API is to install the node-fetch
package.
Use npm or Yarn to install the package as follows:
npm install node-fetch
# If you use TypeScript
npm install @types/node-fetch --save-dev
# For Yarn
yarn add node-fetch
# Yarn and using TypeScript
yarn add @types/node-fetch --dev
Once installed, you can add fetch to your file using the import
statement as follows:
import fetch from 'node-fetch';
This requires you to use the ES Modules syntax or a build tool such as Webpack.
If you want to use the CommonJS require
syntax, then you need to install version 2 of the node-fetch
package:
npm install node-fetch@2
# For Yarn:
yarn add node-fetch@2
Once installed, you can use the CommonJS Modules syntax:
const fetch = require('node-fetch');
fetch("https://api.github.com/users/nsebhastian")
.then(
// ...
)
This way, you can use the fetch API without having to install Node.js version 18 or above.
Conclusion
The ReferenceError: fetch is not defined
occurs when you try to call the fetch()
function from a JavaScript runtime that doesn’t support the fetch API.
This usually happens when you run JavaScript in Node.js, so one way to fix the error is to upgrade Node.js to version 18 which provides native support for the fetch API.
Another way to resolve the error is to download the node-fetch
package, which adds fetch API support to the Node.js runtime.
I hope this tutorial helps. Happy coding! 👍