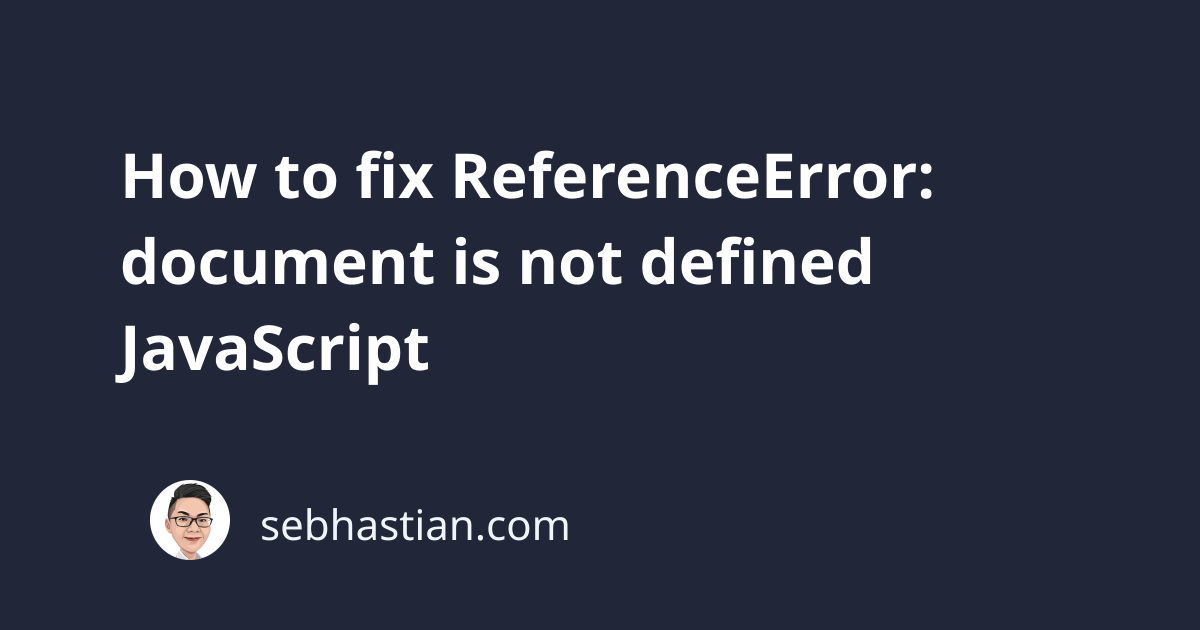
When running JavaScript code, the ReferenceError: document is not defined
occurs when you try to access the global document
object in an environment that doesn’t have the document
object.
Most likely, you’re trying to access the document
object from a Node.js, Next.js, or Deno environment.
Suppose you have an index.js
file with the following contents:
let childEl = document.getElementById("child");
console.log(childEl);
If you use Node.js or Deno to run the file like node index.js
or deno run index.js
, then you get this error:
$ node index.js
ReferenceError: document is not defined
This is because the document
object is part of the browser’s API that you can access using JavaScript. Outside of a browser, there’s no document
object.
To prevent this error, you can check if you’re on the browser environment before accessing the document
object.
The process.browser
property is true
only in the browser environment, so you can use an if
statement to check if this property is not false
like this:
if (process.browser) {
console.log("In Browser, you can access the document object");
var childEl = document.getElementById("child");
console.log(childEl);
} else {
console.log("In nodeJS");
console.log("Unable to access the document object");
}
This way, the document.getElementById()
method only runs when you’re running JavaScript in a browser environment.
I hope this tutorial helps. Happy coding! 👍