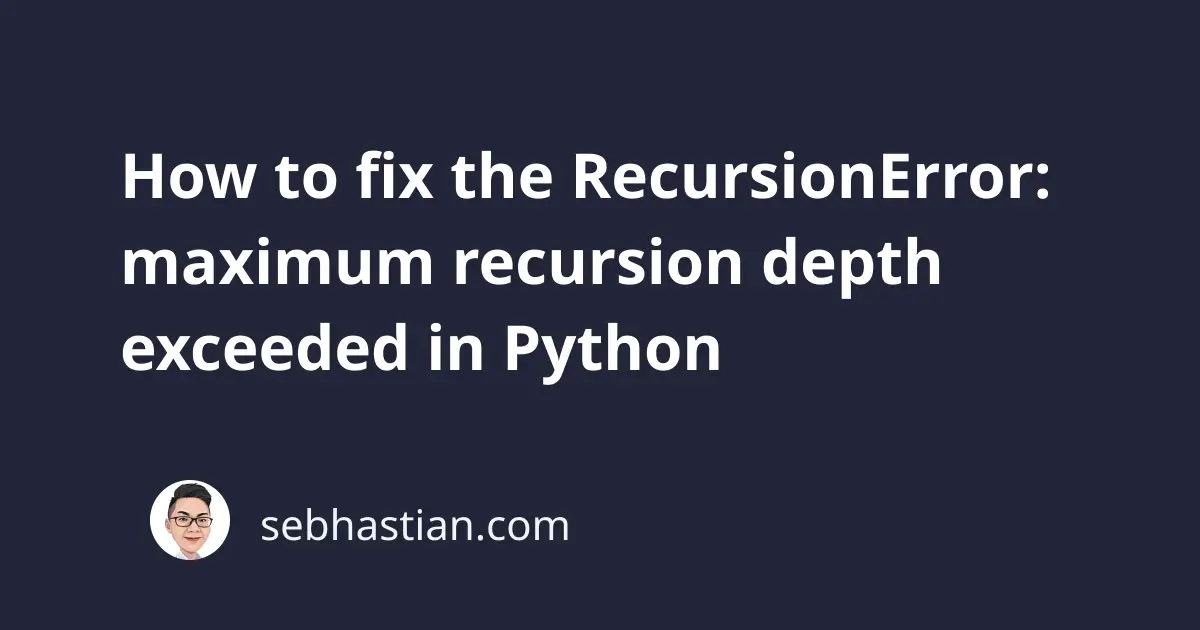
When running a Python program, you might encounter the following error:
RecursionError: maximum recursion depth exceeded
while calling a Python object
After doing some research, I found that this error occurs when a recursive function exceeded the default recursion limit set by Python, which is 1000 times.
Usually, this error occurs because of 2 possible scenarios:
- You don’t have a base case to stop the recursion
- Your recursive code requires more than 1000 depth
This tutorial will help you solve the error in both scenarios.
You don’t have a base case to stop the recursion
In practice, a recursive function will always call itself until a specific condition is met that stops the recursion.
That specific condition is also known as the base case, and your code must be able to reach that condition to stop running.
For example, the following countup()
function prints the number n
recursively:
def countup(n):
print(n)
countup(n + 1)
countup(1)
But since there’s no base case in this function, it will call itself forever, eventually causing a stack overflow.
To prevent the code from running forever, Python placed a soft limit on recursion to 1000 depth. This means when the function calls itself up to 1000 times, Python stops the execution and raises an error:
...
996
997
Traceback (most recent call last):
File "main.py", line 6, in <module>
countup(1)
File "main.py", line 3, in countup
countup(n + 1)
File "main.py", line 3, in countup
countup(n + 1)
File "main.py", line 3, in countup
countup(n + 1)
[Previous line repeated 993 more times]
File "main.py", line 2, in countup
print(n)
RecursionError: maximum recursion depth exceeded
while calling a Python object
To resolve this error, you need to add a base case that prevents the recursion from running infinitely.
For example, stop the countup()
function when it reaches 10
as shown below:
def countup(n):
print(n)
if n < 10:
countup(n + 1)
countup(1)
This way, the countup()
function will only be called as long as the n
variable is less than 10.
This code won’t cause a RecursionError because as soon as the n
variable is 10 or greater, the recursion won’t happen.
Your recursive code requires more than 1000 depth
Python sets the limit of recursion to 1000 to avoid recursive code repeating forever.
You can find this out by calling the getrecursionlimit()
function from the sys
module:
import sys
print(sys.getrecursionlimit())
# Output: 1000
To get over this limitation, you can set the recursion limit using the setrecursionlimit()
function.
This code modifies the recursion limit to 1500:
import sys
sys.setrecursionlimit(1500)
But keep in mind that increasing the limit too many would degrade the performance of Python itself.
If you could, you should use the iterative approach using a while
loop instead of recursion. For example, here’s a modified countup()
function that uses the iterative approach:
def countup(n):
while n < 10:
print(n)
n += 1
countup(1)
You can use the base case as the condition that tells the while
loop when to stop, and do the required operations inside the while block. At the end of the block, add an operation that allows the while
loop to reach the base case.
Conclusion
Python raises the RecursionError: maximum recursion depth exceeded while calling a Python object
when your recursive code exceeded the maximum recursion depth, which is set to 1000 by default.
To resolve this error, you need to create a base case to stop the recursion from going forever, and make sure that the base case can be reached within the allowed recursion depth.
You can change the recursion depth limit, or you can also use an iterative approach.
I hope this tutorial helps. See you in other tutorials! 👋