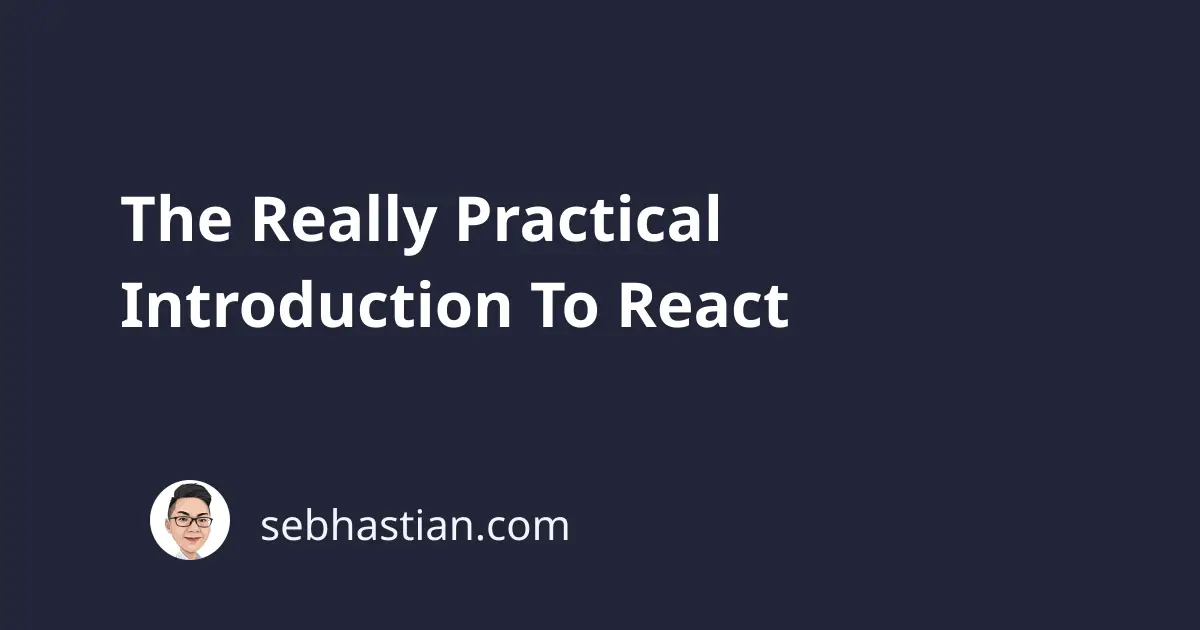
In my last post, I’ve talked a lot about the ground breaking technologies behind React. Now let me guide you in kickstarting a React application. Forgot about all those libraries like Webpack, Redux, Router, and MobX. They won’t come into the scene until you’ve mastered the basics of React.
But how do I start writing React app without understanding the moving parts? Even React itself needs Babel and Webpack to run properly..
Umm.. That may be true in the past, but we have create-react-app
in the present, and I’m confident that it will be everything you need for getting React up and running. Let me show you how.
First, open your terminal and do
npm install -g create-react-app
Next, navigate into a directory where you wish to place your React application, for example:
mkdir react-applications && cd react-applications
Now create react app by running this command:
create-react-app hello-world
This will create a new directory named hello-world and build a React application inside it. Ignore all the stuff going on in your terminal for now. When it’s done, this message will come out:
Happy hacking!
Now let’s run your newborn React application, cd
into hello-world
and run npm start
.
When it’s done, your localhost
will automagically appear and React logo will greet you 🙌 Yay!
Now we’re ready to understand the code.
Go into the src
directory and open up App.js
with your favorite Text Editor. This is the entire code that render our welcome page.
import React, { Component } from 'react';
import logo from './logo.svg';
import './App.css';
class App extends Component {
render() {
return (
<div className="App">
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<p>
Edit <code>src/App.js</code> and save to reload.
</p>
<a
className="App-link"
href="https://reactjs.org"
target="_blank"
rel="noopener noreferrer"
>
Learn React
</a>
</header>
</div>
);
}
}
export default App;
This App.js
file is a React component implementation at its simplest form. You have a component class with a render method that returns a jsx element, and nothing else. It ends with an export statement, which means we have to see the file that import this class. Let’s open index.js
file now.
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import * as serviceWorker from './serviceWorker';
ReactDOM.render(<App />, document.getElementById('root'));
// If you want your app to work offline and load faster, you can change
// unregister() to register() below. Note this comes with some pitfalls.
// Learn more about service workers: http://bit.ly/CRA-PWA
serviceWorker.unregister();
Ignore the serviceWorker
part for now, and let’s focus on ReactDOM. This is code responsible for running the entire React application.
ReactDOM.render(<App />, document.getElementById('root'));
This ReactDOM package will access and modify the dom, thus the code above means render the React <App />
component into the DOM element node where the element has id root
.
You’ll find this root
element in the public/index.html
file. That’s where the entire React application is initialized.
<div id="root"></div>
We stil have a lot to do in order to master React, but for now let’s take a break. We’ll continue in the next lesson.
Let me take a moment to address some concerns you might have:
There is some magic code working behind the create-react-app
module, What should I do to understand them?
The answer, right now, is to forget about them. Yes, in the not so distant future, you have to understand Webpack, Babel, and all other configurations needed to run React application properly. But for now, this bundled React application is your new baby, from which you have to grow and make a viable application.
So what should you learn next?
Get your head wrapped around just React right now. States, props, lifecycles, and more recently, hooks. Master all these core React features first, and only then you may go and learn the configurations around React.
You CAN get really far by only learning React. The key is to write React application by thinking about the interface first. How to create a list of Tweets? How do I make a login interface? Think of what you have to display on screen, in the browser, first. I will post more about building React components in the future. Stay tuned!
Wanna learn more about React? Join my newsletter and be notified when the next post is released 🔔