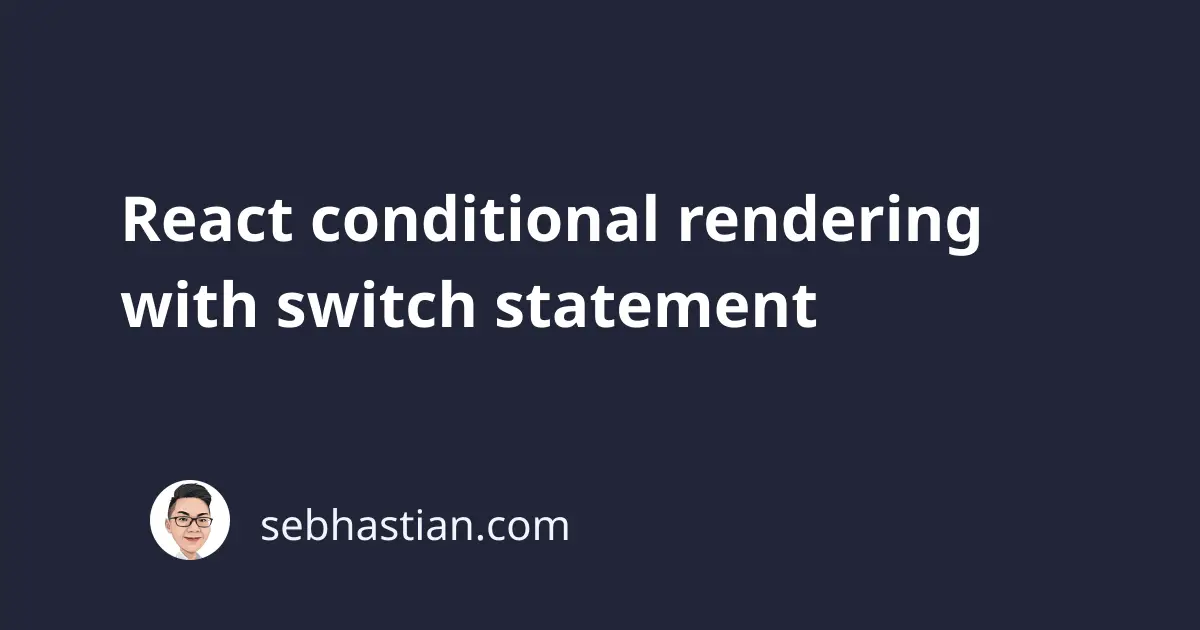
The switch/case
statement is a conditional operator that’s commonly used as an alternative to if...else
statement.
For example, let’s say you have a blogging application. You want to display different layout based on the user’s role:
function App(props){
const { role } = props
if (role === 'author'){
return <AuthorLayout>What will you write today?</AuthorLayout>
}
if (role === 'admin'){
return <AdminLayout>Your latest reports </AdminLayout>
}
if (role === 'moderator'){
return <ModeratorLayout>Your ongoing events</ModeratorLayout>
}
return <GuestLayout>Your current feed</GuestLayout>
}
You can refactor the above component using switch/case
statement as follows:
function App(props){
const { role } = props
switch(role) {
case 'author':
return <AuthorLayout>What will you write today?</AuthorLayout>
case 'admin':
return <AdminLayout>Your latest reports </AdminLayout>
case 'moderator':
return <ModeratorLayout>Your ongoing events</ModeratorLayout>
default:
return <GuestLayout>Your current feed</GuestLayout>
}
}
Unlike in general JavaScript function where you need to use break
statement, React component already has return
statement that stops the switch
operation.
But if you use switch
without return
, then you still need the break
statement to prevent “falling through” the next case:
function App(props){
const { role } = props
let component
switch(role) {
case 'author':
component = <AuthorLayout>What will you write today?</AuthorLayout>
break
case 'admin':
component = <AdminLayout>Your latest reports </AdminLayout>
break
case 'moderator':
component = <ModeratorLayout>Your ongoing events</ModeratorLayout>
break
default:
component = <GuestLayout>Your current feed</GuestLayout>
}
return component
}
Both patterns are valid in React, so you can choose which one’s perfect for your case. When you have a range of conditions, it’s better to use if...else...then
:
if(role === 'author' && isSuspended === false && published === true){
// run the code
}
When you have only one value to evaluate, it’s generally recommended to use switch
.