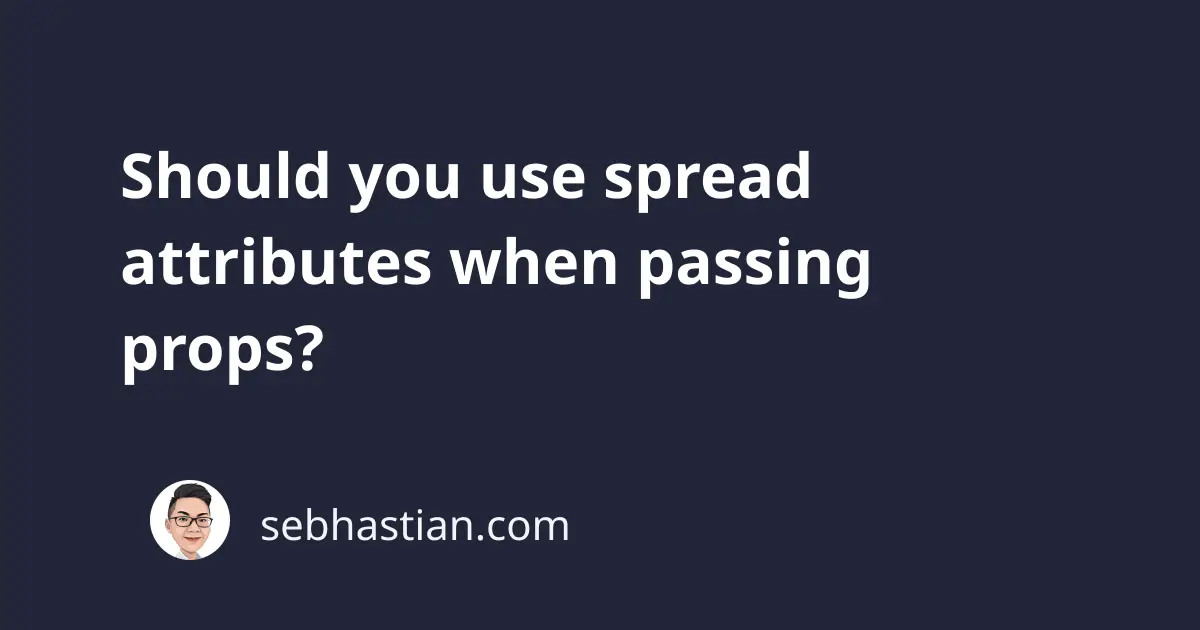
As you’ve probably learned, React library passes data around its components by using props
and state
. To pass data from one component to another, you just need to define the attributes after the component name:
function MainComponent(){
return <Hello name="Jack" />
}
You can then retrieve the attributes from the props
parameter, which always gets passed as the first argument in a function component:
function Hello(props){
return <div>Hello World! My name is {props.name}</div>
}
Ocassionally, you will need to pass multiple props into a component. For example:
function MainComponent(){
return <Hello firstName="Jack" lastName="Skeld" />
}
When you find you need to pass multiple props, you can wrap them as an object and use the spread operator to pass the whole props object:
function MainComponent(){
const props = { firstName: "Jack", lastName: "Skeld" }
return <Hello {...props} />
}
This is a totally valid way to pass props in React, but should you do it?
Personally, I think the spread props pattern is a bad way to pass props because as you develop your React project, you will have a hard time tracking what props are actually being passed from one component to another.
What if the props you passed came from state that gets its values from an API call?
function App() {
const [data, setData] = useState([]);
useEffect(() => {
fetch("https://api.github.com/users?per_page=3")
.then((res) => res.json())
.then(
(data) => {
setData(data);
}
);
}, []);
return (
<Hello {...data} />
);
}
You’ll be confused as to what exactly is being passed from data
state into <Hello>
component. The spread props method is hard to maintain when you have more than ten components around, as you need to look for what actually is being passed into the component.
It’s always much better to explicitly define the attributes you’re passing into the component rather than using the spread method.