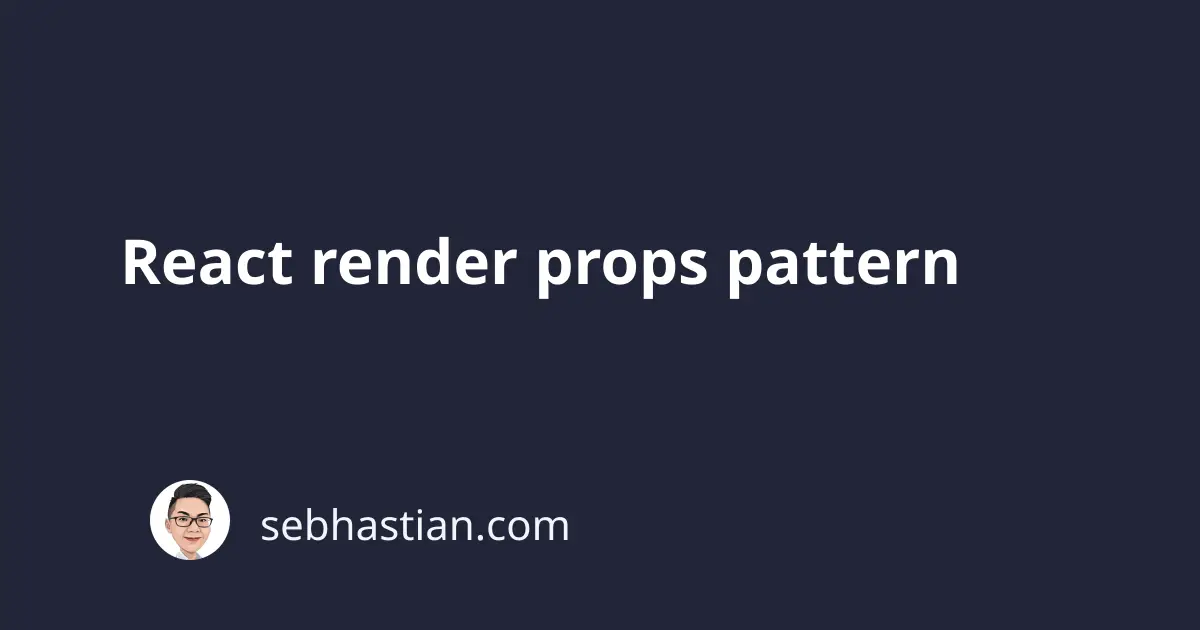
React render props pattern is another advanced pattern in React code beside higher-order component.
Put simply, A render props pattern emerges when you have a component that dynamically renders element to the screen from its prop value.
Yes, it’s a bit confusing, so let me show you a simple example. A render prop needs at least two components:
- the wrapper component who passes the render prop
- the base component who has calls the render prop
The wrapper component could look like this:
class WrapperComponent extends Component {
render(){
return <BaseComponent render={ name => (
<h1>Hello, {name} </h1>
)}/>
}
}
As you can see, the WrapperComponent
will call on BaseComponent
and passed a render
prop. Then the BaseComponent
would call the render
function like this:
class BaseComponent extends Component {
state = {
name: "Danny"
}
render(){
this.props.render(this.state.name)
}
}
Here’s the Code Sandbox example
This way, the BaseComponent
can share its logic across many components, and in the case of the example above, it can share its state value to be rendered in any way the WrapperComponent
wants to:
class WrapperComponent extends Component {
render(){
return <BaseComponent render={ name => (
<h1>Good to see you today, {name}!</h1>
)}/>
}
}
class AnotherWrapper extends Component {
render(){
return <BaseComponent render={ name => (
<p>Howdy Mister {name}!</p>
)}/>
}
}
Just like higher-order component, the render props pattern was very popular to use before the release of React hooks.
For example, to share state like the example above, you can create a new custom hook like this:
export function useName(initialValue = "Danny") {
const [name, setName] = useState(initialValue);
return [name, setName];
}
Then the wrapper components can simply import the function:
function WrapperComponent() {
const [name, setName] = useName();
return <h1>Good to see you today, {name}!</h1>
}
function AnotherWrapper extends Component {
const [name, setName] = useName();
return <p>Howdy Mister {name}!</p>
}
As you can see, using React hooks are more easy to your eyes and far simpler than render props.
If you’re working on new projects, consider using custom hooks instead of render props.