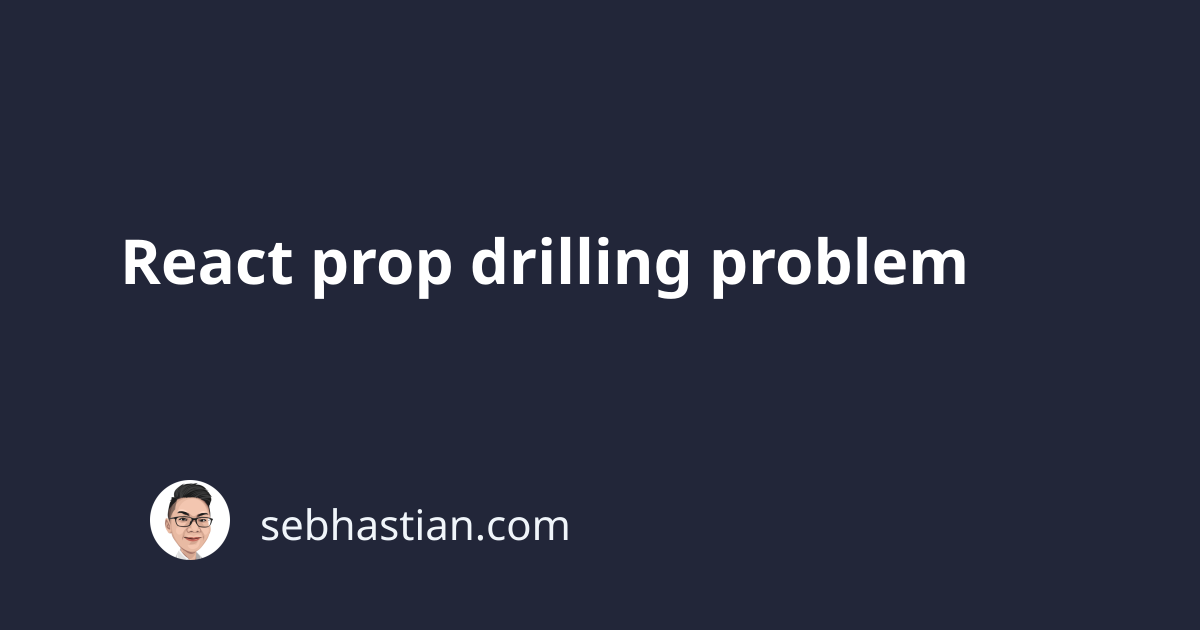
Prop drilling (or also called “threading”) is the code pattern you create when you need to get data from one component into another by passing props multiple times through other components.
For example, imagine you have the following components in your React application:
Here’s an example you can play with in Code Sandbox.
The pattern where you send setTextSwitch from <App>
to <ChangeGreeting>
is called prop drilling. The component <Greeting>
doesn’t need the prop, but you send it anyway because you need to get it into <ChangeGreeting>
.
One way to avoid prop drilling is to keep the number of components down and only create new components when that particular piece of component needs to be reused. Back to the example, you can refactor the code by putting <ChangeGreeting>
inside <Greeting>
component:
function Greeting(props) {
let element;
if (props.text) {
element = (
<p>
Hello! I'm Nathan and I'm a Software Developer. Pleased to meet you!
</p>
);
} else {
element = (
<p>Hello! I'm Jane and I'm a Frontend Developer. Pleased to meet you!</p>
);
}
return (
<div>
{element}
<button onClick={props.handleClick} type="button">
Toggle Name
</button>
</div>
);
}
To be fair, prop drilling isn’t all bad because the pattern provides you with a very specific way of passing data using a top-down approach. But sometimes, it’s just very annoying to specifically pass props down between components, especially when your React application is ten layers deep.
Imagine if you can just declare a global variable in any component and then retrieve it from any component. Without a clear pattern and one-way data flow, you’d have a very confusing data model and have a hard time tracking where some data is initialized, updated and used.
But React does acknowledge that it’s no fun to pass props down multiple components from the initial component. This is why React provides a way to simulate the nature of global variable. Enter the Context API.