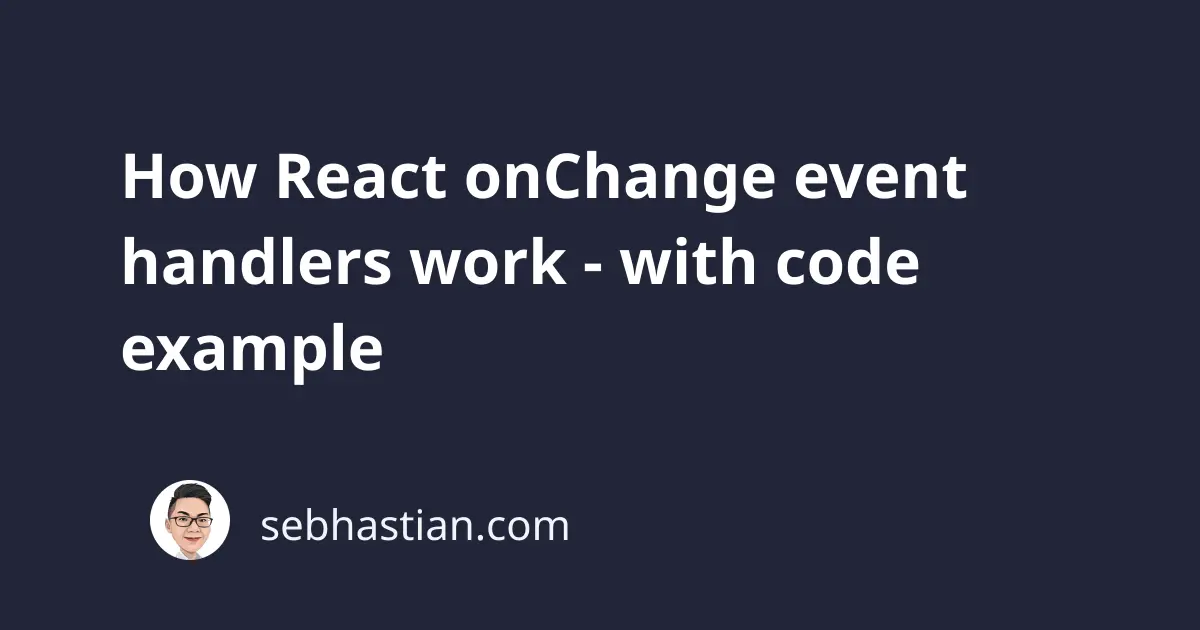
The onChange
event handler is a prop that you can pass into JSX <input>
elements.
This prop is provided by React so that your application can listen to user input in real-time.
When an onChange
event occurs, the prop will call the function you passed as its parameter.
Here’s an example of the onChange
event in action:
import React from "react";
function App() {
function handleChange(event) {
console.log(event.target.value);
}
return (
<input
type="text"
name="firstName"
onChange={handleChange}
/>
);
}
export default App;
In the example above, the handleChange()
function will be called every time the onchange
event occurs for the <input>
element.
The event
object passed into the handleChange()
function contains all the detail about the input event.
You can also declare a function right inside the onChange
prop like this:
import React from "react";
function App() {
return (
<input
type="text"
name="firstName"
onChange={event => console.log("onchange is triggered")}
/>
);
}
export default App;
Now whenever you type something into the text box, React will trigger the function that we passed into the onChange
prop.
Common use cases for React onChange event handler
In regular HTML, form elements such as and usually maintain their own value:
<input id="name" type="text" />
Which you can retrieve by using the document
selector:
var name = document.getElementById("name").value;
In React however, it is encouraged for developers to store input values in the component’s state object.
This way, React component that render <input>
elements will also control what happens on subsequent user inputs.
First, you create a state for the input as follows:
import React, { useState } from "react";
function App(props) {
const [name, setName] = useState("");
}
Then, you create an input element and call the setName
function to update the name
state.
Every time the onChange
event is triggered, React will pass the event
argument into the function that you define inside the prop:
import React, { useState } from "react";
function App(props) {
const [name, setName] = useState("");
return (
<input
type="text"
name="firstName"
onChange={event => setName(event.target.value)}
/>
);
}
Finally, you use the value of name
state and put it inside the input’s value
prop:
return (
<input
type="text"
name="firstName"
onChange={event => setName(event.target.value)}
value={name}
/>
);
You can retrieve input value in event.target.value
and input name in event.target.name
.
As in the previous example, you can also separate the onChange
handler into its own function. The event
object is commonly shortened as e
like this:
import React, { useState } from "react";
function App(props) {
const [name, setName] = useState("");
function handleChange(e) {
setName(e.target.value);
}
return (
<input
type="text"
name="firstName"
onChange={handleChange}
value={name}
/>
);
}
This pattern of using React’s onChange event and the component state will encourage developers to use state as the single source of truth.
Instead of using the Document
object to retrieve input values, you retrieve them from the state.
And now you’ve learned how React onChange
event handler works. Nice job! 👍