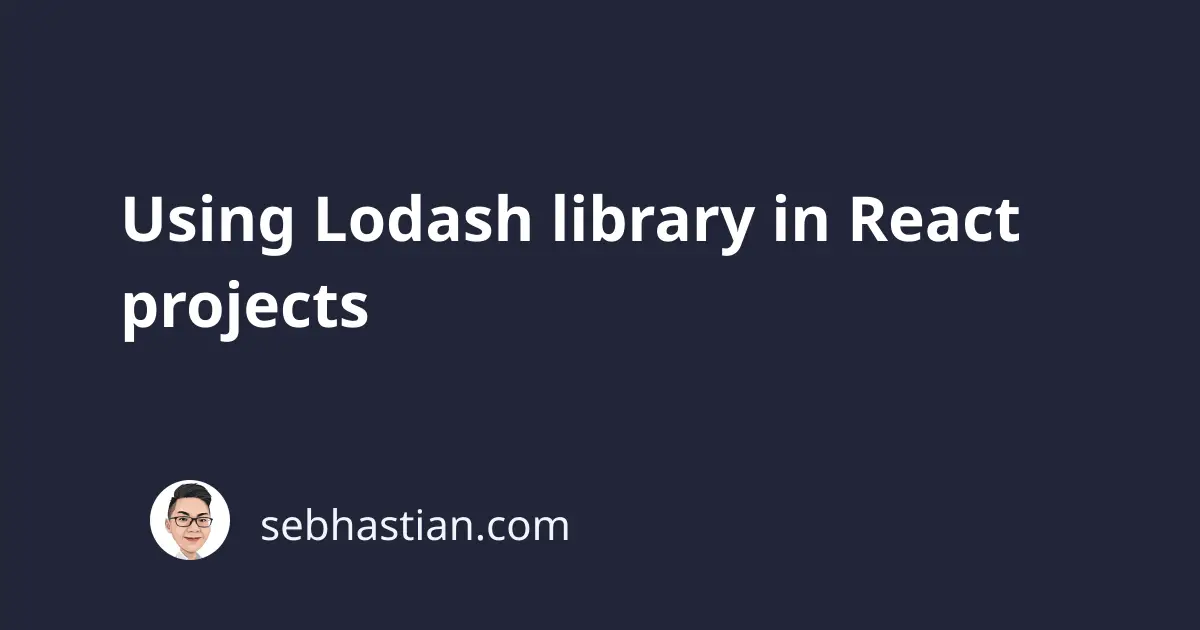
Lodash is a JavaScript library used for helping developers manipulate complex data structures. Lodash provides you with a set of ready to use functions created to operate or modify JavaScript data structures like arrays, numbers, objects, strings, and the rest.
For example, let’s say you want to create an array of numbers from 0 to 10. You can go the normal way with writing each number when you declare the array:
const sampleArray = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
or you can use a loop to make it shorter:
const sampleArray = []
for (let i = 0; i <= 10; i++) {
sampleArray.push(i)
}
// sampleArray = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Now with Lodash, you can do the same thing by calling a single function:
var _ = require('lodash');
const sampleArray = _.range(11);
// sampleArray = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
See how easy it is? Lodash is useful to integrate into React because it helps you do more with less code. Consider the following React component code. It has an array of daily expenses and counts the sum of the expenses as weekly expenses:
export default function App() {
const dailyExpenses = [4, 7, 3, 5, 6, 4, 7];
const weeklyExpenses = dailyExpenses.reduce(function (a, b) {
return a + b;
}, 0);
return (
<div>
<h1>My daily expenses</h1>
<ul>
{dailyExpenses.map(function (expense, index) {
return <li key={index}>{expense}</li>;
})}
</ul>
<h2>Total expenses this week: {weeklyExpenses}</h2>
</div>
);
}
With Lodash, you can use _.sum
to sum up all the expenses. No need to use reduce
and manually add two numbers step by step:
const weeklyExpenses = _.sum(dailyExpenses);
Very neat, isn’t it? Lodash is included in Create-React-App by default, so you don’t need to do another install to use it. Lodash is usually imported as underscore _
:
import React from "react";
import _ from "lodash";
function App() {
const greeting = _.join(["Hello", "World", "From", "React"], " ");
// Hello World From React
return <h1>{greeting}</h1>;
}
export default App;
There’s also a neat package called react-lodash
that transforms Lodash utility functions into React components. Here’s an example of conditional rendering by using Lodash’s isEmpty
and map
functions:
import react from 'react'
import { IsEmpty, Map } from "react-lodash"
export default function App() {
const dailyExpenses = [4, 7, 3, 5, 6, 4, 7];
return (
<IsEmpty
value={dailyExpenses}
yes="Empty list"
no={() => (
<ul>
<Map collection={dailyExpenses} iteratee={i => <li key={i}>{i}</li>} />
</ul>
)}
/>
);
}
Check out react-lodash’s documentation to explore its 296 available components.