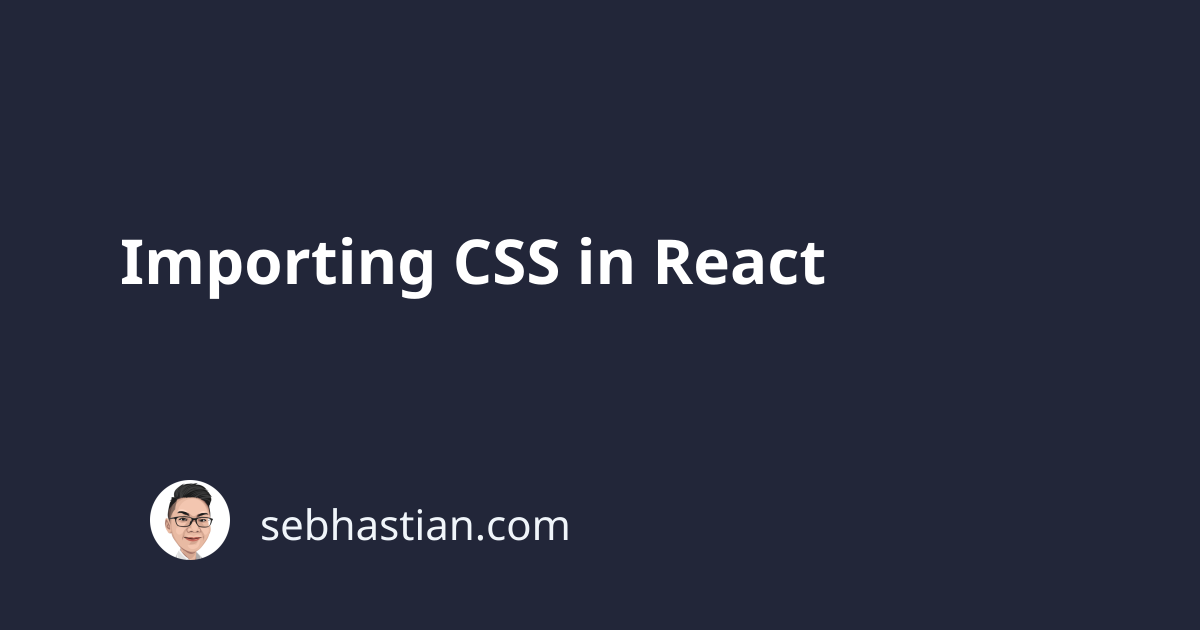
Popular React application bootstrappers like Create React App and Next.js already support importing CSS inside JavaScript files.
CSS in Create React App
In Create React App, all you need to do is write the CSS and import it once inside your application.
For example, let’s create a .css
file named style.css
:
.App {
font-family: sans-serif;
text-align: center;
}
.hello {
color: blue;
}
Next, write an App.js
file and write a simple React component with classes:
import React from "react";
export default function App() {
return (
<div className="App">
<h1 className="Hello">Hello World! My name is John</h1>
</div>
);
}
Finally, write an index.js
file where you import the component and render it to the DOM. You need to import the CSS file here also:
import React from "react";
import ReactDOM from "react-dom";
import "./styles.css";
import App from "./App";
const rootElement = document.getElementById("root");
ReactDOM.render(
<App />,
rootElement
);
And you will see the <h1>
text will have a blue color, even though you didn’t import the CSS directly in the component. In production build, all imported CSS files will be concatenated into a single minified CSS file and get imported to the application in the <head>
.
CSS in Next.js
Just like CRA, Next.js already has built-in support for CSS. The only difference is where you need to import the file. To add a global CSS file to your Next.js app, you need to import the file inside pages/_app.js
file.
The _app.js
file will override the default component in which Next.js renders your pages. That’s why this is the ideal place to import a global CSS file:
import '../styles.css'
// This default export is required in a new `pages/_app.js` file.
export default function MyApp({ Component, pageProps }) {
return <Component {...pageProps} />
}
For further information, please visit Next.js documentations for CSS here
What about custom boilerplates?
Now if you’re not using either Create React App or Next.js, then you have to configure the Style and CSS loaders in your module bundler.
It will be different for each bundler, but if you use Webpack, you can follow this boilerplate example that I’ve created before.