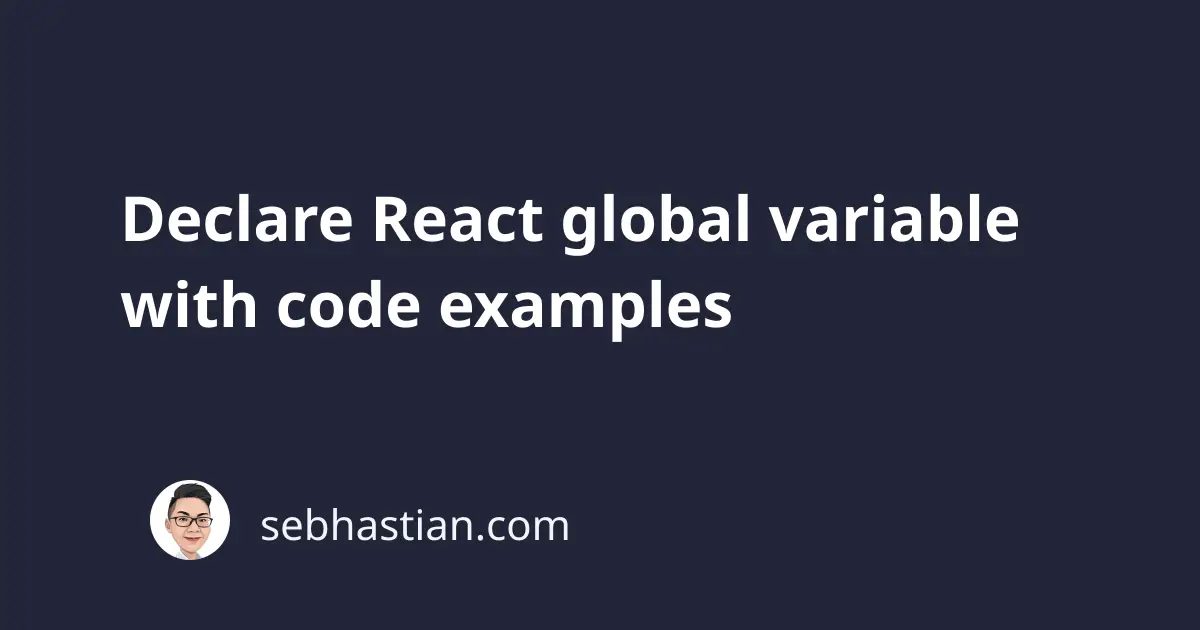
There are two ways you can declare a global variable in a React application:
This tutorial will cover both ways mentioned above.
Attaching global variable to the window object
One way to declare a global variable in React is to attach a new variable as the property of the window
object.
For example, create a window.name
variable in the index.js
file like this:
import React from "react";
import ReactDOM from "react-dom";
import App from "./App";
window.name = "John";
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
);
Once the variable is declared, you can call it from another React component you have in your project.
Here’s an example of calling the variable from the App.js
file:
import React from "react";
export default function App() {
return <h1>Hello {window.name}</h1>;
}
The window
object can be created anywhere inside your code (it doesn’t have to be in the parent component)
In the code below, the global variable is declared inside the App.js
file:
import React from "react";
window.user = "Nathan";
export default function App() {
return <h1>Hello World!</h1>;
}
The user
variable can still be called from the index.js
file as shown below:
root.render(
<React.StrictMode>
<App />
<p>Logged in as {window.user}</p>
</React.StrictMode>
);
Although it’s not required, you can prefix the variable name with the dollar sign ($
) to identify it as a global variable.
The $
will differentiate a global variable from a regular one:
window.$name = "John";
Add the $
when calling it from other components:
export default function App() {
return <h1>Hello {window.$name}</h1>;
}
And that’s how you declare a global variable using the window
object.
Using an env file to declare global variables
When you bootstrap your application using Create React App or Next.js, your application will automatically load a .env
file that you created in the root directory.
You can create the .env
file and declare a global variable there. It will be accessible from all the components you have in your application.
First, create a file named .env
in your root directory:
touch .env
Then declare a global variable in the env file. The convention in .env
file is to use all uppercase letters for the variable name:
REACT_APP_NAME_VARIABLE = John
To call on the environment variable, use the process.env.{ your variable name }
syntax as follows:
export default function App() {
return (
<div className="App">
<h1>Hello {process.env.REACT_APP_NAME_VARIABLE}</h1>
<h2>Start editing to see some magic happen!</h2>
</div>
);
}
For further information, you can look at the documentation of Create React App or Next.js.
Unlike state and props, the global variables you declared inside the window
or process.env
object won’t be tracked for change.
This means React will not update the UI when you change them. They are good for variables that have constant value and never change through the lifetime of your application.
If you want global variables that get tracked by React and update your UI according to its changes, then you need to use React Context API instead.
And that’s it for declaring a React global variable. Thanks for reading! 🙏