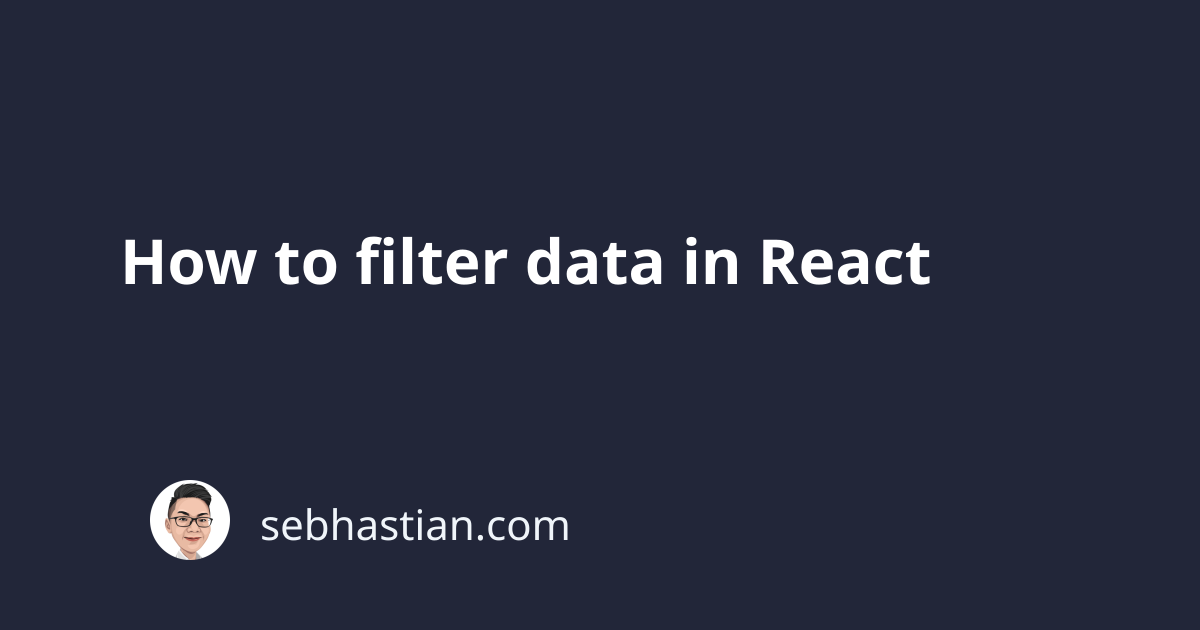
As you develop your React application, there will be times when you need to filter some data and display only those that meets your criteria.
The JSX syntax doesn’t introduce any feature for filtering data at all. You need to use the filter()
function that’s already provided by JavaScript language.
For example, you can filter an array of numbers and return those greater than 60:
function App(){
const array = [ 23, 45, 67, 89, 12 ]
const largerThanSixty = array.filter( number => {
return number > 60
})
return (
<ul> Number greater than 60:
{ largerThanSixty.map(number => <li>{number}</li>) }
</ul>
)
}
The filter()
function accepts a callback function and use it to test the value of each element. It will then return an array with the elements that pass the test. If no elements pass the test, an empty array will be returned. You can then use the map()
function to render the new array.
You can chain the filter()
and map()
function together to shorten your code like this:
function App(){
const array = [ 23, 45, 67, 89, 12 ]
return (
<ul>
{
array
.filter(number => number > 60)
.map(number => <li>{number}</li>)
}
</ul>
)
}
The result will be the same. You can use the filter with any type of data.
Filtering an array of objects
You can filter an array of objects by checking on the object value. For example, let’s say you have an array of tasks:
const tasks = [
{
taskId : 1,
taskName : 'Clean the bathroom',
taskStatus: 'Complete'
},
{
taskId : 2,
taskName : 'Learn filtering data in React',
taskStatus: 'To do'
},
// ... the rest of the data
]
When you only want to display tasks that haven’t been completed yet, you can filter the array using the value of taskStatus
property, as follows:
function App(){
const tasks = [
{
taskId : 1,
taskName : 'Clean the bathroom',
taskStatus: 'Complete'
},
{
taskId : 2,
taskName : 'Learn filtering data in React',
taskStatus: 'To do'
},
{
taskId : 3,
taskName : 'Fix the bug on React project',
taskStatus: 'To do'
},
{
taskId : 4,
taskName : 'Fix the car',
taskStatus: 'Complete'
}
]
return (
<ul> To-do list:
{
tasks
.filter(task => task.taskStatus === 'To do')
.map(task => <li key={task.taskId}>{task.taskName}</li>)
}
</ul>
)
}
And that’s how you can filter data in React. There’s no extra syntax or template, just pure JavaScript.