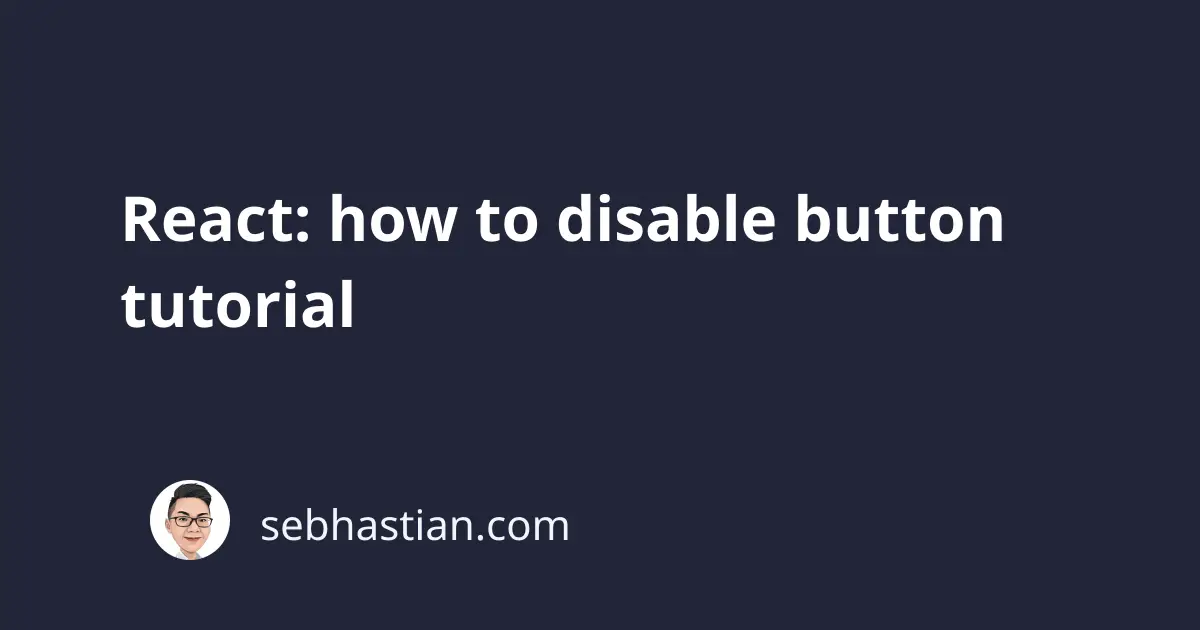
When you need to disable a button using React, you can simply add the disabled
prop to your <button>
element:
function App() {
return <button disabled={true}>Click me!</button>;
}
Knowing this, you can easily modify the code according to your requirements to disable the <button>
.
React disable button after click
For example, you may want to disable a <button>
after it has been clicked. You can do so by adding a state that controls the value of disabled
prop. Let’s name the state disable
and set its default value to false
:
const [disable, setDisable] = React.useState(false);
After that, you need to use the disable
state value as the value of disabled
prop in the <button>
element.
Finally, add an onClick
prop to the <button>
element that will set the disable
state to true
.
The full code is as shown below:
import React from "react";
function App() {
const [disable, setDisable] = React.useState(false);
return (
<button disabled={disable} onClick={() => setDisable(true)}>
Click me!
</button>
);
}
With the code above, your button will be disabled once it receives a click event.
React disable button for login form
In another example, you may have a login button that you want to disable as long as the email and password <input>
is empty.
You can easily do so by checking controlling the value of both <input>
elements using the state.
When the value of email
or password
state is empty, the disabled
prop value must be true
. You can do so by using the following code:
disabled={!email || !password}
The following code example shows how to do it:
import React, { useState } from "react";
function App() {
const [email, setEmail] = useState("");
const [password, setPassword] = useState("");
const handleEmailChange = (event) => {
setEmail(event.target.value);
};
const handlePasswordChange = (event) => {
setPassword(event.target.value);
};
const handleSubmit = (event) => {
event.preventDefault();
alert(`Your state values: \n
email: ${email} \n
password: ${password} \n
You can replace this alert with your process`);
};
return (
<form onSubmit={handleSubmit}>
<div>
<label>Email address</label>
<input
type="email"
name="email"
placeholder="Enter email"
onChange={handleEmailChange}
value={email}
/>
</div>
<div>
<label>Password</label>
<input
type="password"
name="password"
placeholder="Enter password"
onChange={handlePasswordChange}
value={password}
/>
</div>
<button type="submit" disabled={!email || !password}>
Login
</button>
</form>
);
}
This way, your <button>
element will be disabled as long as the value of email
or password
state is falsy
Conclusion
To conclude, you can disable a <button>
element in React by setting the disabled
prop to true
.
Depending on the conditions required to disable the <button>
, you can manipulate the disabled
prop through various ways, such as by checking if the form values are empty or the <button>
has been clicked by the user.