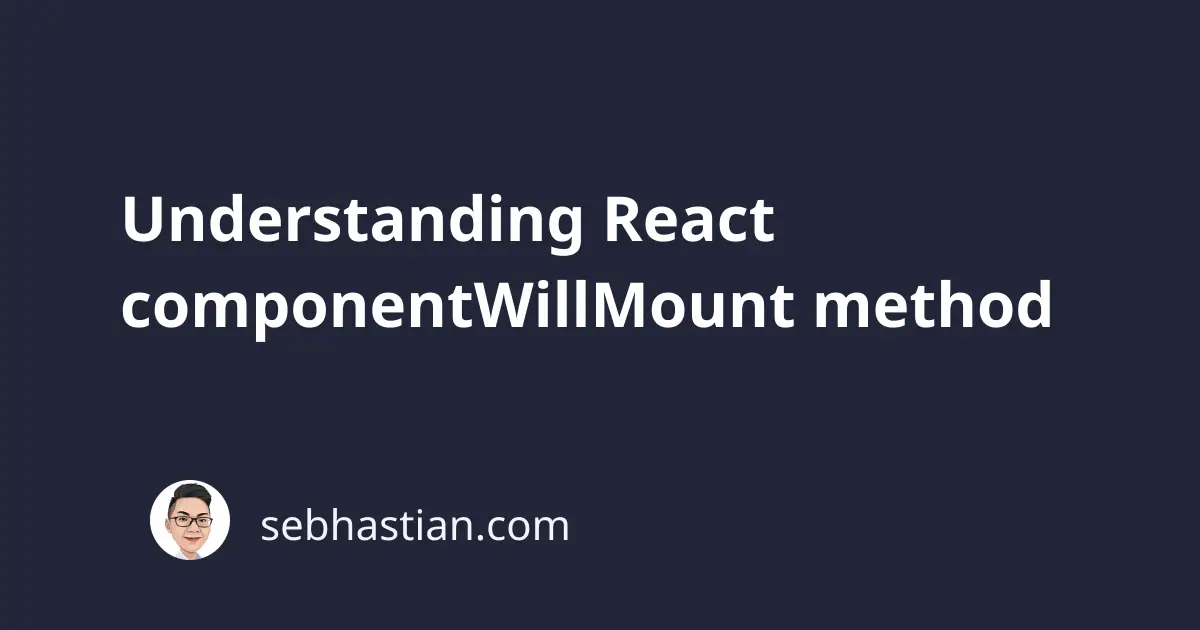
The componentWillMount
method is a lifecycle method of React library that gets executed inside components right before the component gets attached to the DOM. This lifecycle method is generally used to update the state value right before the component is rendered to the DOM.
Years after the release of React, the React team noticed that this lifecycle method is problematic for several reasons:
Fetching remote data is inefficient
Generally, the componentWillMount
method is used for fetching data from remote servers into the component. A typical pattern of the method looks like this:
function componentWillMount(){
axios.post()
.then( res => this.setState({res}) )
}
But the problem with this approach is that React component doesn’t wait until the componentWillMount
is finished before rendering the UI. This means that although you can start fetching data before the component gets rendered, the response will most likely be returned after the component is rendered to the screen.
This means you still need to initialize and handle empty state because React will throw an error if you execute operations on undefined
state value.
The function gets called twice with server rendering
The componentWillMount
method is the only function called on server rendering, but the irony with this method is that the method also gets called on the client-side. This means that the method will cause performance issue when you need to do server-side rendering.
The function is replaced with class constructor
Before the release of ES6 class syntax, the componentWillMount
method is used to initialize the state value. But with the release of class syntax, you can use the constructor method to initialize state value and bind event handlers.
As ES6 classes became the main style of writing React components, the use of componentWillMount
became unnecessary.
How to replace componentWillMount
When you need to initialize state, you can replace componentWillMount()
with the constructor()
. When you need to fetch data from remote servers, you can use the componentDidMount
method.
The componentDidMount()
is executed after React component has been rendered to the DOM, so if you fetch remote data with it, make sure you handle the initial state properly.
Learn more about React lifecycle methods: Understanding React lifecycle methods