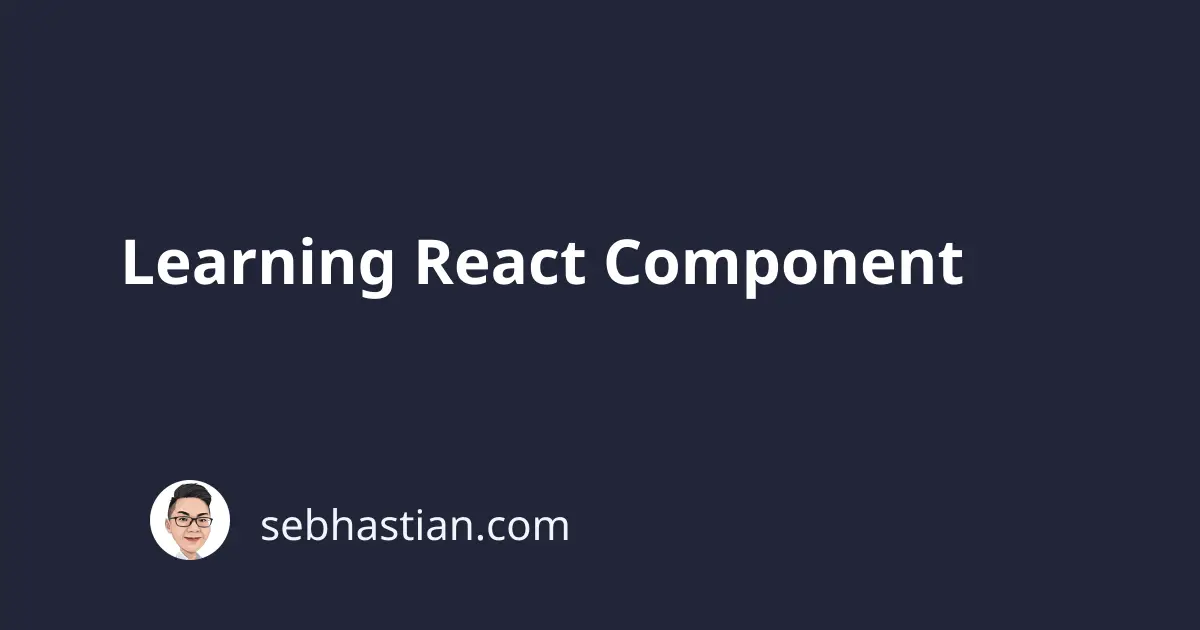
Components and props
A component acts as the container to the whole UI code: it must include a piece of interface logic, which is what you write using JSX, and optional manipulation logic, which is written in JavaScript functions. A component can be declared in two ways: using the function syntax and class syntax.
A class component would be written like this:
class sampleComponent extends React.Component {
render() {
return (
<React.Fragment>
<h1>Hello World!</h1>
<h2>Good to see you here. I'm currently testing React components</h2>
</React.Fragment>
);
}
}
While it’s function counterpart would look like this:
function sampleComponent() {
return (
<React.Fragment>
<h1>Hello World!</h1>
<h2>Good to see you here. I'm currently testing React components</h2>
</React.Fragment>
);
}
React interprets them as the same thing — that’s right, a component. Keep in mind that a class component must always implement the render()
method, and a component must always have a return
statement.
(Which means both class and function component must have a return
. In order to return nothing, write return null
.)
Composing components
A component can be nested inside another component’s render method. Composing component means forming the user interface by using loosely coupled components in a top-down hierarchies. It’s kind of like making a robot out of lego blocks, as I will show you in this demo.
First, let’s make a Card
component that display a card filled with information.
function Card() {
return (
<div className='card'>
<img
className='card-img-top'
src='https://sebhastian.com/static/4d13c75e6afd3976800de29628da5ba5/fcc29/feature-image.png'
alt='JavaScript Basics Before You Learn React'
/>
<div className='card-body'>
<h5 className='card-title'>JavaScript Basics Before You Learn React</h5>
<p className='card-text'>
Learn the prerequisites of learning React fast
</p>
<a
href='https://sebhastian.com/js-before-react'
className='btn btn-primary'>
Learn more
</a>
</div>
</div>
);
}
There’s nothing new inside this Card
component, just ordinary React elements written in JSX with bootstrap classes for styling. Now let’s write another component named CardList
:
function CardList() {
return (
<div className='row'>
<div className='col-sm-4'>
<Card />
</div>
<div className='col-sm-4'>
<Card />
</div>
<div className='col-sm-4'>
<Card />
</div>
</div>
);
}
Notice how Card
component is being called on CardList
component. Component call to needs to be accompanied by the less than <
and greater than >
symbol, just like regular HTML.
One more thing: React treats components starting with lowercase letters as DOM tags, like a <div>
. That’s why every time you write a component, please start the name with a capital letter, like Card
and CardList
. If you start a component with lowercase, React won’t render the component.
Props — a way to send data
Every React component you create comes with props
, or properties. They are like parameters that can be used to accept data when a component is called. First, you send a data to the component on call:
function CardList() {
return (
<div className='row'>
<div className='col-sm-4'>
<Card title='Props are awesome!' />
</div>
</div>
);
}
In the code above, you are sending a title
props
into the Card
component. A props
is a regular JavaScript object, and you need to add props
as parameter to your component before you use it. Now let’s use the title
props
in the card title and image alt
tag of the Card
component:
function Card(props) {
return (
<div className='card'>
<img
className='card-img-top'
src='https://sebhastian.com/static/4d13c75e6afd3976800de29628da5ba5/fcc29/feature-image.png'
alt={props.title}
/>
<div className='card-body'>
<h5 className='card-title'>{props.title}</h5>
<p className='card-text'>
Learn the prerequisites of learning React fast
</p>
<a
href='https://sebhastian.com/js-before-react'
className='btn btn-primary'>
Learn more
</a>
</div>
</div>
);
}
Props are read-only, so please don’t do something like changing the title
above:
function Card(props) {
props.title = "Changing props are bad. Please don't.";
return (
<div className='card'>
<img
className='card-img-top'
src='https://sebhastian.com/static/4d13c75e6afd3976800de29628da5ba5/fcc29/feature-image.png'
alt={props.title}
/>
<div className='card-body'>
<h5 className='card-title'>{props.title}</h5>
<p className='card-text'>
Learn the prerequisites of learning React fast
</p>
<a
href='https://sebhastian.com/js-before-react'
className='btn btn-primary'>
Learn more
</a>
</div>
</div>
);
}
Oh, and here is the same Card
component but using class declaration. You only need to add this
before the props
keyword. By the way, you can mix function and class declaration of components in a single file.
class Card extends React.Component {
render() {
return (
<div className='card'>
<img
className='card-img-top'
src='https://sebhastian.com/static/4d13c75e6afd3976800de29628da5ba5/fcc29/feature-image.png'
alt={this.props.title}
/>
<div className='card-body'>
<h5 className='card-title'>{this.props.title}</h5>
<p className='card-text'>
Learn the prerequisites of learning React fast
</p>
<a
href='https://sebhastian.com/js-before-react'
className='btn btn-primary'>
Learn more
</a>
</div>
</div>
);
}
}
Let’s wrap this part up by writing some more props for the Card
component. You can view a working demo here:
function Card(props) {
return (
<div className="card">
<img
className="card-img-top"
src={props.featureImage}
alt={props.title}
/>
<div className="card-body">
<h5 className="card-title">{props.title}</h5>
<p className="card-text">{props.description}</p>
<a href={props.link} className="btn btn-primary">
Learn more
</a>
</div>
</div>
);
}
function CardList() {
return (
<div className="row">
<div className="col-sm-4">
<Card
featureImage="https://sebhastian.com/static/eb0e936c0ef42ded5c6b8140ece37d3e/fcc29/feature-image.png"
title="How To Make Interactive ReactJS Form"
description="Let's write some interactive form with React"
link="https://sebhastian.com/interactive-react-form"
/>
</div>
<div className="col-sm-4">
<Card
featureImage="https://sebhastian.com/static/4257b49310455388f3e155f8d5ab632e/fcc29/feature-image.png"
title="Babelify your JavaScript code"
description="Babel make JavaScript code browser-compatible."
link="https://sebhastian.com/babel-guide"
/>
</div>
<div className="col-sm-4">
<Card
featureImage="https://sebhastian.com/static/4d13c75e6afd3976800de29628da5ba5/fcc29/feature-image.png"
title="JavaScript Basics Before You Learn React"
description="Learn the prerequisites of learning React fast"
link="https://sebhastian.com/js-before-react"
/>
</div>
</div>
);
}
ReactDOM.render(<CardList />, document.getElementById("root"));
The CardList
is good to go, and you have learned components and props. Congratulations!