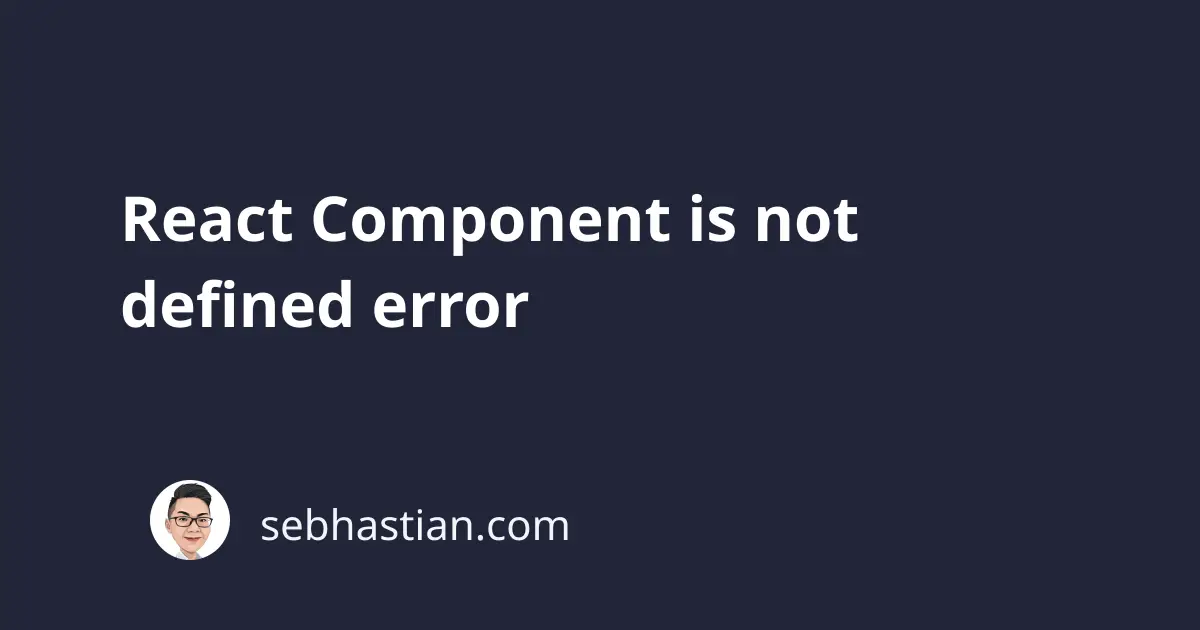
The error “Component is not defined” is triggered when you use the Component
class in React without actually importing it. For example:
import React from "react";
class Hello extends Component {
render(){
return <h1> Hello World~ </h1>
}
}
export default Hello;
Inside the code above, the class Hello
extends React’s Component
class, but you haven’t imported any Component
class at all. There are two ways to fix this.
First, you can import Component
from React:
import React, {Component} from "react";
Or you can extends React.Component
:
class Hello extends React.Component {
// ...
}
And that’s how you fix the Component is not defined error!
By the way, please don’t feel embarrassed if you encounter this error. This is due to React allowing you to create a component using both JavaScript’s class and function declaration. When you’re still learning React, it’s natural to find this error and don’t know what caused it.