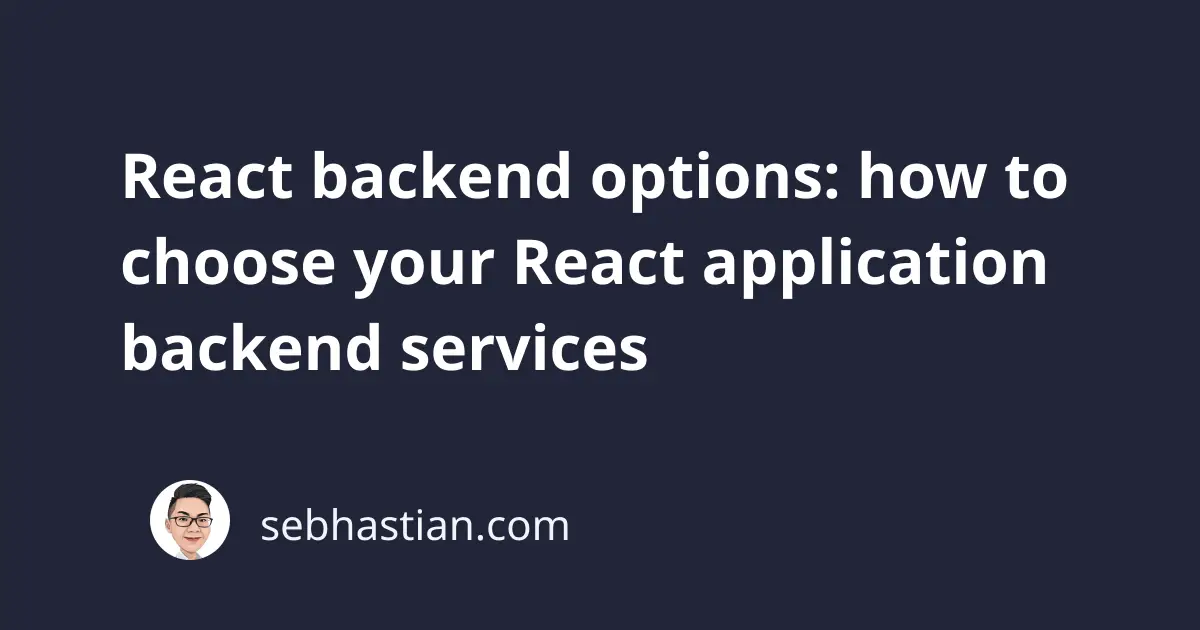
Because React is a frontend-only library, you will need some kind of server to accommodate some of the most common backend services like:
- User authentications
- Database access and manipulation
- Integration with other APIs and platforms
Accessing your database using React will create a vulnerability in your application because users can access your React source code from the browser console.
You can use any kind of backend service that you’re familiar with to serve as the backend stack of your React application.
Some of the most common React stack combinations are as follows:
- Next.js (A complete React framework where you can run database calls)
- MERN stack (MongoDB, Express, React, NodeJS)
- LARM stack (Laravel, React, MySQL)
- RRR stack (Ruby, Rails, React)
- PDR stack (Python, Django, React)
React is very flexible because it only provides you with the means to connect to backend services using HTTP requests.
For example, you can create a simple Express server with the following code:
const express = require("express");
const PORT = process.env.PORT || 5000;
const app = express();
app.get("/api", (req, res) => {
res.json({ message: "Hello from Express!" });
});
app.listen(PORT, () => {
console.log(`Server listening on ${PORT}`);
});
Then, you can execute an HTTP request from your React component to retrieve the data from the server you created above. To send an HTTP request, you can use the built-in fetch()
method or axios
library.
You need to place the request inside the componentDidMount()
lifecycle method for class components:
componentDidMount() {
fetch("http://localhost:5000/api")
.then((res) => res.json())
.then(
(data) => {
this.setState({
data: data.message
});
},
(error) => {
console.log(error)
}
);
}
Or inside the useEffect()
hook for function components:
useEffect(() => {
fetch("http://localhost:5000/api")
.then((res) => res.json())
.then((data) => setMessage(data.message));
}, []);
Once the server returns a response, you can assign that response as a value of your component’s state:
function App() {
const [message, setMessage] = useState(null);
useEffect(() => {
fetch("http://localhost:5000/api")
.then((res) => res.json())
.then((data) => setMessage(data.message));
}, []);
return (
<div className="App">
<h1>{!data ? "Loading..." : data}</h1>
</div>
);
}
What stack you need to use with React really depends on the requirement of your projects and what programming language you and your team are comfortable with.
One stack that I’d recommend you to try out is Next.js because it’s a production-ready React framework that’s equivalent to what Laravel and Rails are for PHP and Ruby.
As your application becomes more complex, you may also want to use Server-Side Rendering (SSR) technique with your React application.
Next.js already has support for SSR because it uses the NodeJS environment for the server under the hood.
If you’re using other environments like Laravel and Rails, then you may want to check out the support for SSR first.
To conclude, I’d say you can use any backend services you’re comfortable with, including BaaS like Google Firebase and Microsoft Azure.
If you don’t have any preferences, then I’d suggest you try out Next.js for your next React project. It has everything you need for creating a full-stack React application.