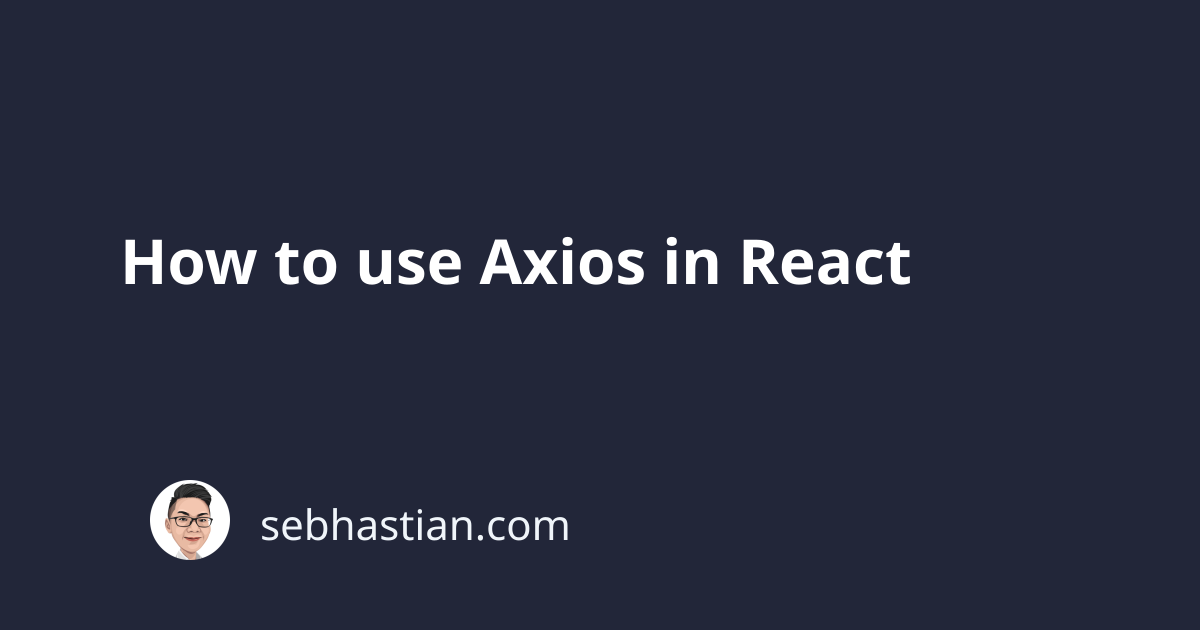
Axios is a JavaScript library for creating HTTP requests. It’s similar to the native fetch
API, but has more useful features, including:
- Make XMLHttpRequests from the browser
- Make http requests from node.js
- Supports the Promise API
- Intercept request and response
- Transform request and response data
- Cancel requests
- Automatic transforms for JSON data
- Client side support for protecting against XSRF
Generally, Axios is more popular with JavaScript developers because it automatically converts JSON response into a valid JavaScript array or object and can be used both in the server and the browser (fetch
is not native to Node.js)
Using Axios in React
Just like using Fetch, you need to put your Axios request inside the componentDidMount()
function or a useEffect
hook because the ideal place to fetch data with React.
Here’s a sample code for class-based component:
componentDidMount() {
axios.get("https://api.github.com/users?per_page=3")
.then(
(response) => {
this.setState({
data: response.data
});
}
)
.catch(error => {
console.log(error)
})
}
And here’s a sampe for function-based component:
useEffect(() => {
axios.get("https://api.github.com/users?per_page=3")
.then((response) => {
setData(response.data);
})
.catch((error) => {
console.log(error);
});
}, []);
Requests example with Axios
Axios supports all HTTP request methods. you can specify the request method using the axios object configuration:
axios({
method: 'get',
url: 'https://api.github.com/users'
})
.then(function (response) {
console.log(response)
});
// or
axios({
method: 'post',
url: 'https://api.github.com/users',
data: {
firstName: 'Fred',
lastName: 'Flintstone'
}
})
.then(function (response) {
console.log(response)
});
Or using function aliases by calling the right method:
axios.get("https://api.github.com/users")
axios.post("https://api.github.com/users")
axios.put("https://api.github.com/users")
axios.patch("https://api.github.com/users")
axios.delete("https://api.github.com/users")
You can put the request data
argument right after the url
:
axios.post("https://api.github.com/users", {
firstName: 'Fred',
lastName: 'Flintstone'
})
Executing multiple concurrent requests
You can use Promise.all()
function with Axios to wait for multiple requests to return a response before your JavaScript app execute the next line of code:
function getUserAccount() {
return axios.get('/user/12345');
}
function getUserPermissions() {
return axios.post('/user/12345/permissions', {
firstName: 'Fred',
lastName: 'Flintstone'
});
}
Promise.all([getUserAccount(), getUserPermissions()])
.then(function (results) {
const acct = results[0];
const perm = results[1];
});
Axios will return the response as an array, following the order of the functions you put as the arguments in Promise.all()
function.
For more information, you can read Axios documentation