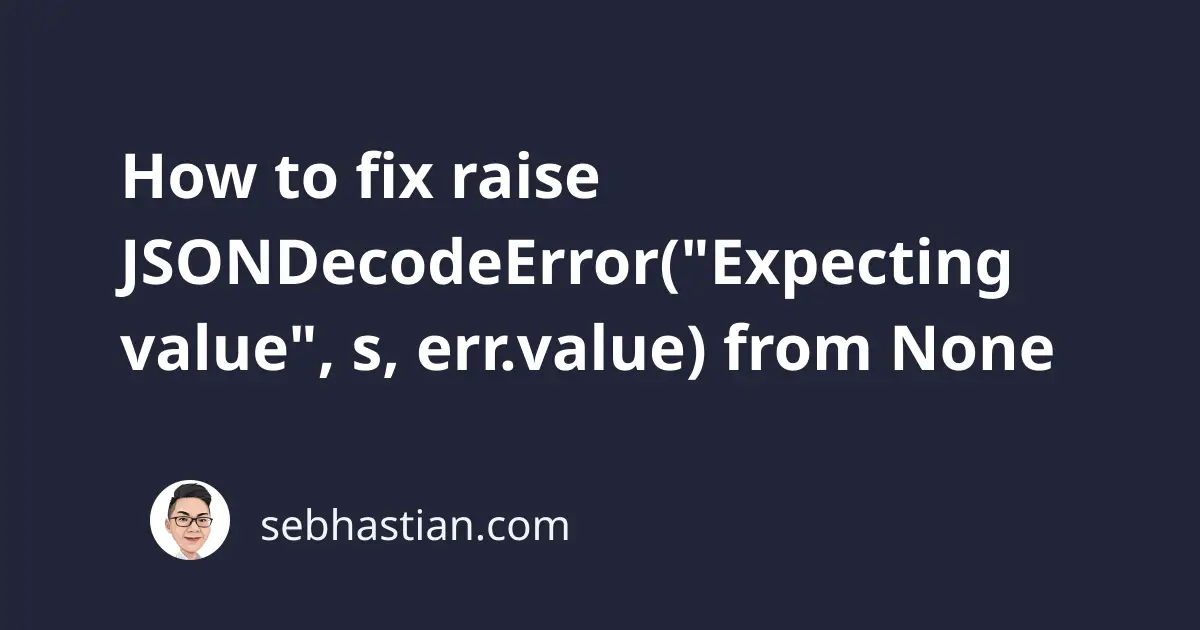
When working with JSON data in Python, you might get the following error:
raise JSONDecodeError("Expecting value", s, err.value) from None
This error occurs when you specify an incompatible data type as an argument to the json.loads()
or json.load()
method.
The following tutorial will show you an example that causes this error and how to fix it.
How to reproduce this error
Suppose you get some JSON data from a file or from an API request, then you call the loads()
method to convert the JSON string into a dictionary as follows:
data = res.read()
json_object = json.loads(data)
When loading the data
object, you get the following error:
Traceback (most recent call last):
File "main.py", line 6, in <module>
json_object = json.loads(data)
^^^^^^^^^^^^^^^^
File ...
return _default_decoder.decode(s)
^^^^^^^^^^^^^^^^^^^^^^^^^^
File ...
obj, end = self.raw_decode(s, idx=_w(s, 0).end())
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File ...
raise JSONDecodeError("Expecting value", s, err.value) from None
The error happens because the loads()
method can’t convert the data
object.
This usually happens when you pass a bytes
object to the method. You can check for the type of the object using the type()
function:
print(type(data)) # <class 'bytes'>
# json_object = json.loads(data)
If the type()
function returns the bytes
class, then you need to convert this data before passing it to the loads()
method.
How to fix this error
To resolve this error, you need to pass a valid JSON string in UTF-8 format to the loads()
method.
You can use the bytes.decode()
method to convert the bytes object as follows:
data = res.read()
json_object = json.loads(data.decode("UTF-8"))
By adding the call to decode()
as shown above, the bytes object gets converted into UTF-8 string, which is a valid JSON format that Python can handle.
If that doesn’t work, then you need to check if you have a valid JSON string format. You can use an online validator like JSONLint to help you verify the string.
I hope this tutorial is helpful. Happy coding! 👍