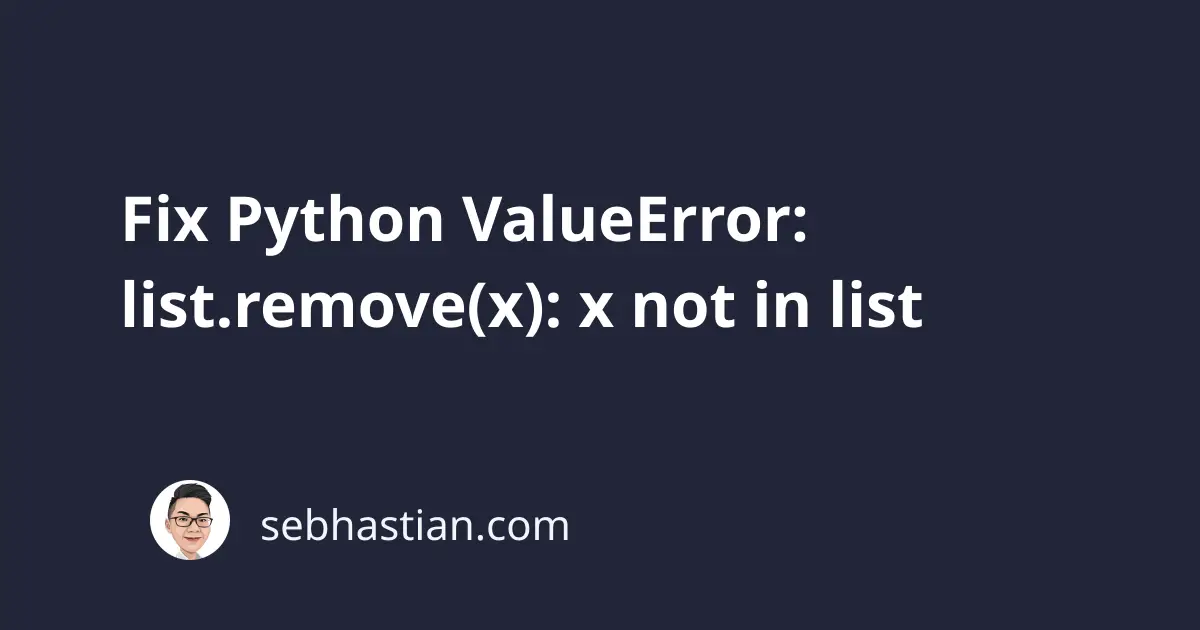
The Python ValueError: list.remove(x): x not in list means that you are trying to remove an element that doesn’t exist in the list.
To fix this error, you need to make sure the item to remove exists on your list.
See the following example with the animals
list:
# Create a list of animal names
animals = ["horse", "lion", "cat"]
animals.remove("dog") # ❗️ValueError
In the code above, the remove()
function is called to remove the dog
item from the animals
list.
But since the list doesn’t have the item, Python responds with the ValueError message as shown below:
Before you call the remove()
function, you can check whether the item exists on the list using the if .. in
statement.
The following code shows how to use the in
keyword to test whether the dog
string exists in the list:
animals = ["horse", "lion", "cat"]
if "dog" in animals:
animals.remove("dog")
print(animals)
else:
print("Item doesn't exist on the list")
When the value you want to remove exists, you can remove it.
Besides an if .. else
block, you can also use a try .. except
block to handle the error:
animals = ["horse", "lion", "cat"]
try:
animals.remove("dog")
except ValueError:
print("Item doesn't exist on the list")
Finally, keep in mind that the remove()
function accepts exactly one argument.
Some developers not familiar with this causes the error by trying to remove more than one item at once:
animals = ["horse", "lion", "cat"]
animals.remove("horse, lion") # ❗️ValueError
The error happens because the list contains two separate values: horse
and lion
.
The remove()
function in the code above tries to remove a single value horse, lion
, which doesn’t exist.
To remove two items, you need to call the remove()
function twice:
animals = ["horse", "lion", "cat"]
animals.remove("horse") # ✅
animals.remove("lion") # ✅
If you have too many items, you can use a for
loop to avoid repeating your code.
Consider the following example:
animals = ["horse", "lion", "cat", "fish"]
items_to_remove = ["horse", "lion", "fish"]
for item in items_to_remove:
animals.remove(item)
print(animals) # ['cat']
The items_to_remove
list contains values that you want to remove from your list.
Next, use the for
loop to iterate over the items in the items_to_remove
list.
For each item
in the list, it calls the remove()
method on the animals
list to remove the item
from the list.
Finally, the print()
function is called on the modified animals
list. The output shows ['cat']
, which means that the horse
, lion
, and fish
items were successfully removed from the animals
list.
Conclusion
The Python “ValueError: list.remove(x): x not in list” error occurs when you try to remove an item from a list using the list.remove() method, but the item is not present in the list.
To fix this error, you need to make sure that the item you are trying to remove exists in the list.
One way to do this is to use the if .. in
statement to check whether the item is present in the list before calling the list.remove()
method.
Alternatively, you can use a try .. except
block to handle the ValueError exception that is raised when the item is not found in the list.
It’s also important to remember that the list.remove()
method only accepts a single argument, so you cannot pass multiple items to be removed in a single call.
If you want to remove multiple items from a list, you need to call the method multiple times or use a for
loop to iterate over the items you want to remove.
Great work learning how to fix the Python error! 👍