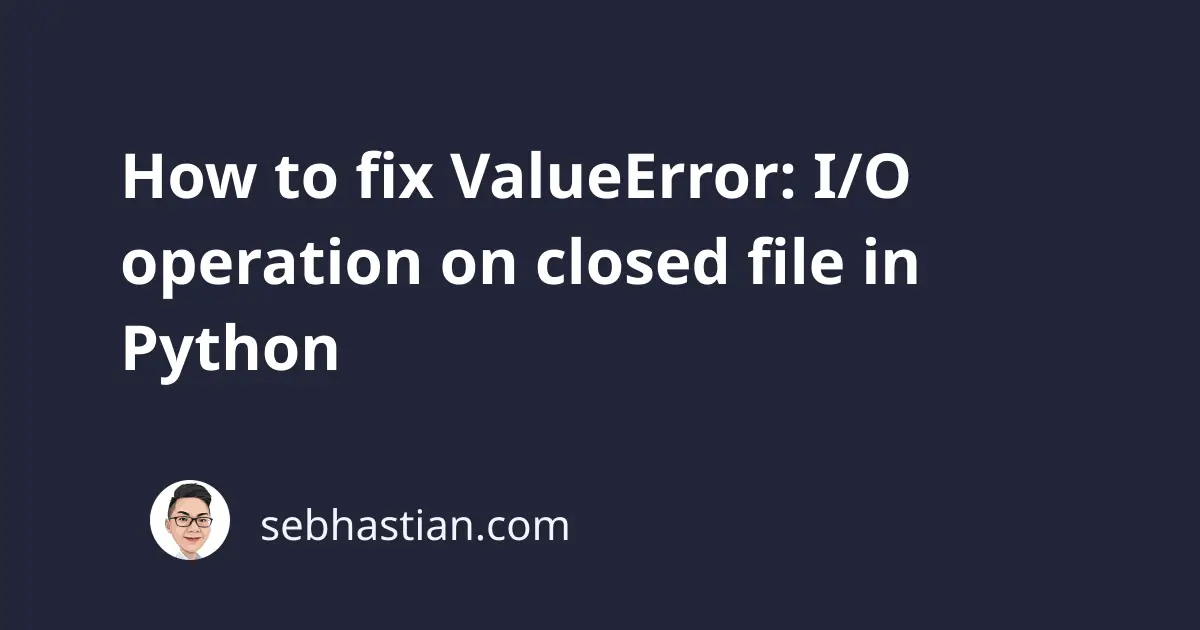
When working with files using Python code, you might get the following error:
ValueError: I/O operation on closed file.
From my experience and research, I found that this error usually occurs when you try to perform a read or write operation on a file that’s already closed. For example:
- You manipulate the file outside of the with block
- You read or write to CSV files after the file is closed
This tutorial will show you examples that cause this error and how to fix them.
1. You manipulate the file outside of the with
block
When you use the with
statement to open a file, you need to perform operations on the file inside the with
block.
The following code opens a text file named file.txt
file and writes a line in that file:
file_name = "file.txt"
with open(file_name, "w", encoding="utf-8") as f_obj:
f_obj.write("first line" + "\n")
If you try to write a second line outside of the with
block as follows:
file_name = "file.txt"
with open(file_name, "w", encoding="utf-8") as f_obj:
f_obj.write("first line" + "\n")
f_obj.write("Second line" + "\n")
You’ll get this error:
Traceback (most recent call last):
File "main.py", line 6, in <module>
f_obj.write("Second line" + "\n")
ValueError: I/O operation on closed file.
To fix this error, you need to put the write operation inside the with
block by indenting the code:
file_name = "file.txt"
with open(file_name, "w", encoding="utf-8") as f_obj:
f_obj.write("first line" + "\n")
f_obj.write("Second line" + "\n") # ✅
Python automatically closes the file when it reaches the end of the with
block, so you need to make sure any code that has something to do with the file is inside the block.
Alternatively, you can also open the file without using the with
statement as follows:
file_name = "file.txt"
f_obj = open(file_name, "w", encoding="utf-8")
f_obj.write("first line" + "\n")
f_obj.write("Second line" + "\n")
f_obj.close()
In the above code, the result of calling the open()
function is stored in the f_obj
variable.
Unlike when using the with
statement, you can manipulate the file even when you include line breaks.
When you’re done processing the file, you need to call the close()
method on the file object to avoid memory leaks.
Notice that if you read or write after calling the close()
method, the same error will be raised.
2. Working with CSV files
The same error also appears no matter what file extension your file has. You might be opening a .csv
or a .json
file, this error still appears when you try to read or write outside the with
block.
For example, you might have the following code to read a CSV file:
import csv
with open('data.csv', newline='') as f:
reader = csv.reader(f)
for row in reader:
print(row)
The for
loop in the code above lacks an indent, so the same error is triggered.
To fix this, indent the for
loop as follows:
import csv
with open('data.csv', newline='') as f:
reader = csv.reader(f)
for row in reader:
print(row)
Here’s an alternative syntax without the with
statement:
import csv
f_obj = open('data.csv', newline='')
reader = csv.reader(f_obj)
for row in reader:
print(row)
f_obj.close()
Notice that you didn’t get the “I/O operation on closed file” error this time.
Summary
To sum it up, the ValueError: I/O operation on closed file
occurs when you attempt to read from or write to a file that’s already been closed by Python.
To resolve this error, make sure that you’re executing a read or write operation on a file that’s still open. If you’re using the with
statement, make sure that any operations on the file are done inside the statement.
Otherwise, make sure you’re not manipulating the file after calling the close()
method.
I hope this tutorial is helpful. I’ll see you again in other articles! 👋